How to Search Data in the DataGridView Using RadioButton in C#
Submitted by janobe on Wednesday, September 4, 2019 - 19:41.
In this tutorial, I will teach how to search for data in the datagridview using radiobutton in c#. This program illustrates how the data change in the datagridview according to the selected radiobutton. This is very helpful for you if you want to retrieve a group of data in the database. I use Microsoft Visual Studio 2015 and MySQL Database to create this project.
Download the complete source code and run it on your computer.
For any questions about this article. You can contact me @
Email – [email protected]
Mobile No. – 09305235027 – TNT
Or feel free to comment below.
Creating Database
Go to localhost/PHPMyAdmin/ and create a database named “tuts_persondb” Write the following query to create a table.
Write the following query to insert the data in the table
Creating Application
Step 1
Open Microsoft Visual Studio 2015 and create a new windows form application c#.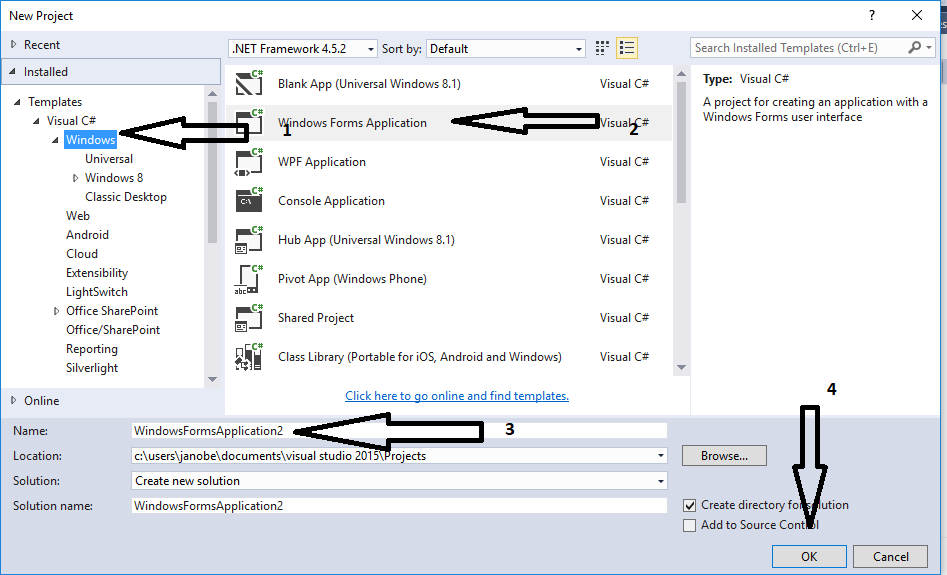
Step 2
Add a GroupBox, two RadioButtons, and a DataGridView in the form. After that, design the form just like shown below.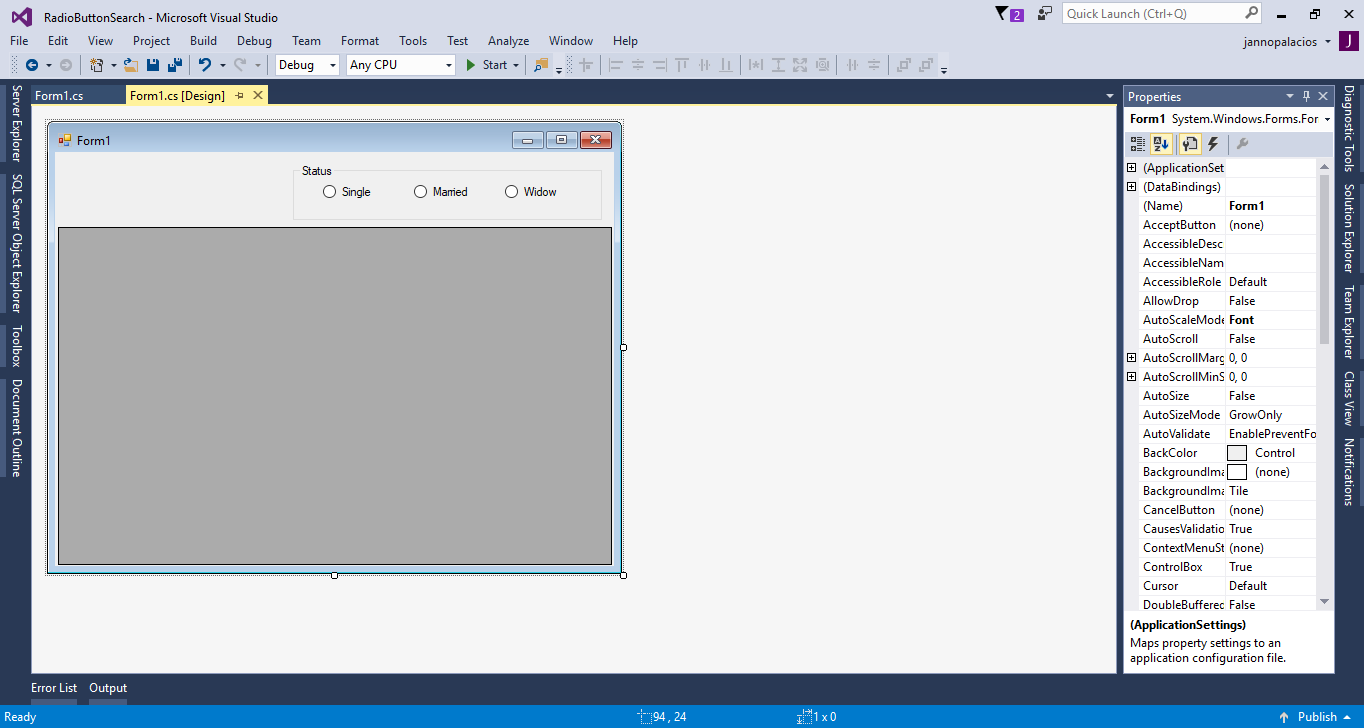
Step 3
Add MySQL.data.dllStep 4
Press F7 to open the code editor. After that, add a namespace to accessMySQL
libraries.
- using MySql.Data.MySqlClient;
Step 5
Inside the class, create a connection between MySQL Database and C#. After that, declare all the classes that are needed.- MySqlConnection con = new MySqlConnection("server=localhost;user id=root;password=;database=tuts_persondb;sslMode=none");
- MySqlCommand cmd;
- MySqlDataAdapter da;
- DataTable dt;
- string sql;
Step 5
Create a method for retrieving data in the database.- private void loaddata(string sql,DataGridView dtg)
- {
- try
- {
- con.Open();
- cmd.Connection = con;
- cmd.CommandText = sql;
- da.SelectCommand = cmd;
- da.Fill(dt);
- dtg.DataSource = dt;
- }
- catch(Exception ex)
- {
- MessageBox.Show(ex.Message);
- }
- finally
- {
- con.Close();
- da.Dispose();
- }
- }
Step 6
Do the following codes for retrieving data in the first load of the form.- private void Form1_Load(object sender, EventArgs e)
- {
- sql = "Select * From tblperson";
- loaddata(sql, dataGridView1);
- }
Step 7
Do the following codes for finding records when the radio button is checked.- private void rdoSingle_CheckedChanged(object sender, EventArgs e)
- {
- sql = "Select * From tblperson WHERE CivilStatus = '" + rdoSingle.Text + "'";
- loaddata(sql, dataGridView1);
- }
- private void btnMarried_CheckedChanged(object sender, EventArgs e)
- {
- sql = "Select * From tblperson WHERE CivilStatus = '" + rdoMarried.Text + "'";
- loaddata(sql, dataGridView1);
- }
- private void btnWidow_CheckedChanged(object sender, EventArgs e)
- {
- sql = "Select * From tblperson WHERE CivilStatus = '" + rdoWidow.Text + "'";
- loaddata(sql, dataGridView1);
- }
Add new comment
- 1104 views