How to Create a Simple Addition Using Console Application in VB.net
Submitted by janobe on Wednesday, January 23, 2019 - 20:24.
In writing a program, there are times that you have to deal with the input and output process. The question is what kind of method you have to use to work it. So, in this tutorial I will teach you how to create a simple addition using console application in VB.net. This simple method will help you process the command line itself to find out what kind of output the user wants to prompt.
Creating Application
Step 1
Open Microsoft Visual Studio 2015 and create a new console application.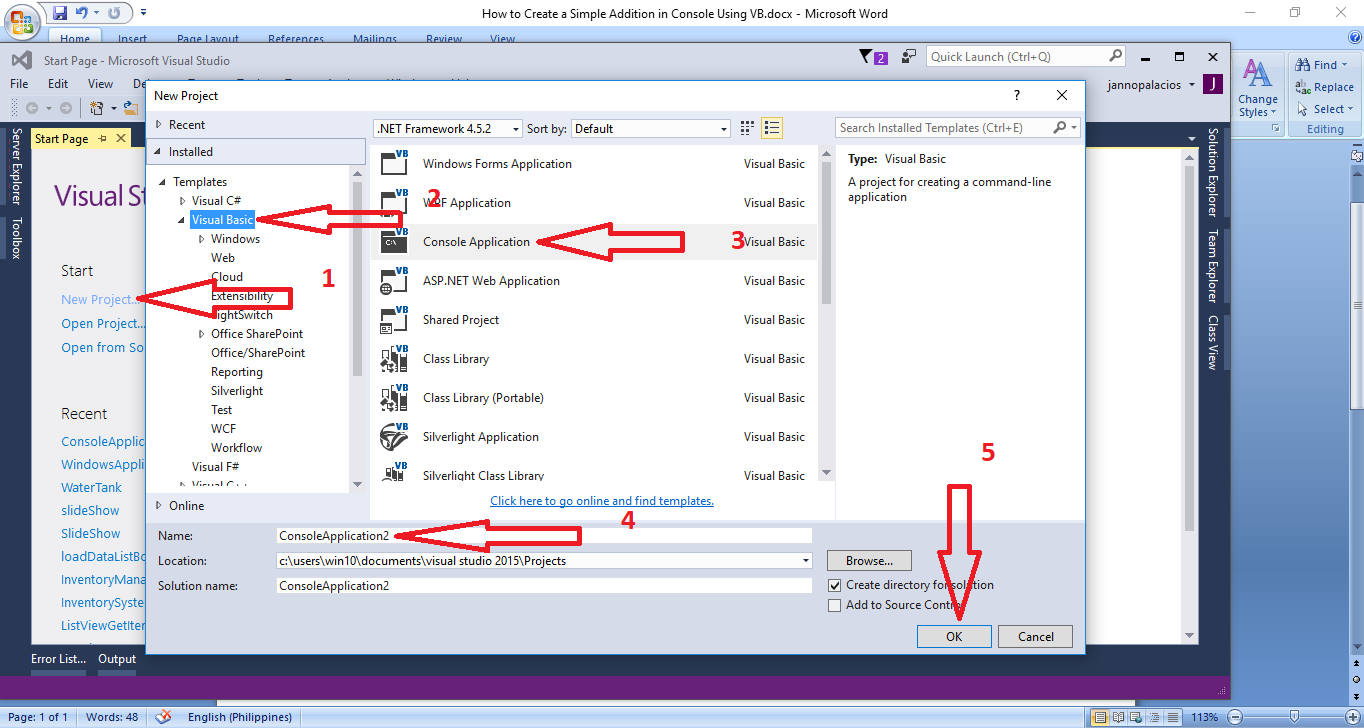
Step 2
Write this following code for the input and output of the program.- 'DECLEARING VARIABLE
- Dim num1
- Dim num2
- Dim total
- 'WRITES THE SPECIFIED STRING VALUE
- Console.WriteLine("Input first number : ")
- 'READ THE NEXT LINE OF CHARACTER FROM THE STANDARD INPUT STREAM
- num1 = Console.ReadLine()
- 'WRITES THE SPECIFIED STRING VALUE
- Console.WriteLine("Input second number : ")
- 'READ THE NEXT LINE OF CHARACTER FROM THE STANDARD INPUT STREAM
- num2 = Console.ReadLine()
- 'FORMULA FOR ADDITION
- total = Val(num1) + Val(num2)
- 'WRITE THE OUTPUT FOR ADDITION
- Console.WriteLine("Total is : " & total)
- Console.ReadLine()
Step 3
Full source code.- Module Module1
- Sub Main()
- 'DECLEARING VARIABLE
- Dim num1
- Dim num2
- Dim total
- 'WRITES THE SPECIFIED STRING VALUE
- Console.WriteLine("Input first number : ")
- 'READ THE NEXT LINE OF CHARACTER FROM THE STANDARD INPUT STREAM
- num1 = Console.ReadLine()
- 'WRITES THE SPECIFIED STRING VALUE
- Console.WriteLine("Input second number : ")
- 'READ THE NEXT LINE OF CHARACTER FROM THE STANDARD INPUT STREAM
- num2 = Console.ReadLine()
- 'FORMULA FOR ADDITION
- total = Val(num1) + Val(num2)
- 'WRITE THE OUTPUT FOR ADDITION
- Console.WriteLine("Total is : " & total)
- Console.ReadLine()
- End Sub
- End Module
Comments
Add new comment
- Add new comment
- 1554 views