PHP - Populate Select Option Using SQLite
Submitted by razormist on Thursday, June 21, 2018 - 21:03.
In this tutorial we will create a Populate Select Option using SQLite. This code can compressed multiple files at the same time. PHP is a server-side scripting language designed primarily for web development. SQLite provides an interface for accessing the database. It includes class interfaces to the SQL commands. And also it allows you to create SQL functions and aggregate using PHP. So Let's do the coding...
3. Save changes and Restart Server.
food_get.php
There you have it we successfully created a Populate Select Option using SQLite. I hope that this simple tutorial help you to what you are looking for. For more updates and tutorials just kindly visit this site. Enjoy Coding!!!
Before we get started:
First you have to download & install XAMPP or any local server that run PHP scripts. Here's the link for XAMPP server https://www.apachefriends.org/index.html. And this is the link for the jquery that i used in this tutorial https://jquery.com/. Lastly, this is the link for the bootstrap that i used for the layout design https://getbootstrap.com/.Setting Up SQLite
First, we are going to enable SQLite 3 in our PHP. 1. Open localhost server folder XAMPP, etc and locate php.ini. 2. Open php.ini and enable sqlite3 by removing the semicolon in the line.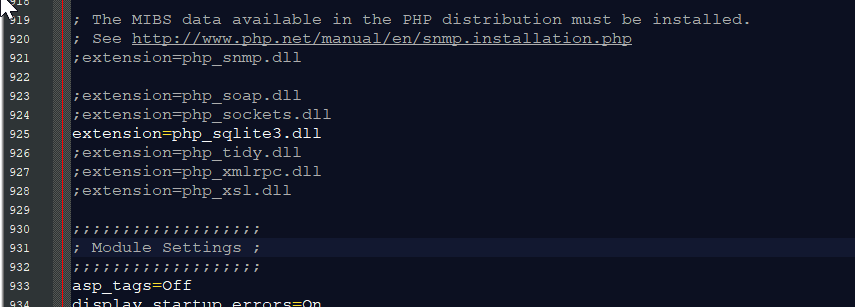
Creating the database connection
Open your any kind of text editor(notepad++, etc..). Then just copy/paste the code below then name it conn.php.- <?php
- $conn = new SQLite3('db_food.db');
- $query = "CREATE TABLE IF NOT EXISTS food (group_id INTEGER PRIMARY KEY AUTOINCREMENT NOT NULL, food_category VARCHAR, food VARCHAR)";
- ?>
Creating The Interface
This is where we will create a simple form for our application. To create the forms simply copy and write it into your text editor, then save it as index.php.- <!DOCTYPE html>
- <html lang="en">
- <head>
- <meta charset="UTF-8" name="viewport" content="width=device-width, initial-scale=1"/>
- <link rel="stylesheet" tyoe="text/css" href="css/bootstrap.css"/>
- </head>
- <body>
- <nav class="navbar navbar-default">
- <div class="container-fluid">
- <a class="navbar-brand" href="<a href="https://sourcecodester.com">Sourcecodester</a>
- </div>
- </nav>
- <div class="col-md-3"></div>
- <div class="col-md-6 well">
- <h3 class="text-primary">PHP - Populate Select Option Using SQLite</h3>
- <hr style="border-top:1px dotted #ccc;"/>
- <button class="btn btn-primary" data-toggle="modal" data-target="#form_modal">Add Data</button>
- <br /><br />
- <div class = "form-inline">
- <label>Food Category:</label>
- <select id = "category" class = "form-control" required = "required">
- <option value = "">Select a Category</option>
- <?php
- require 'conn.php';
- $query = $conn->query("SELECT * FROM `food` GROUP BY `food_category`");
- while($fetch = $query->fetchArray()){
- ?>
- <option value="<?php echo $fetch['food_category']?>"><?php echo $fetch['food_category']?></option>
- <?php
- }
- ?>
- </select>
- <label>Food:</label>
- <select id = "food" class = "form-control" disabled = "disabled" required = "required">
- <option value = "">Select a food</option>
- </select>
- </div>
- </div>
- <div class="modal fade" id="form_modal" tabindex="-1" role="dialog" aria-hidden="true">
- <div class="modal-dialog" role="document">
- <form action="save_query.php" method="POST">
- <div class="modal-content">
- <div class="modal-body">
- <div class="col-md-2"></div>
- <div class="col-md-8">
- <div class="form-group">
- <label>Food Category</label>
- <input class="form-control" type="text" name="food_category">
- </div>
- <div class="form-group">
- <label>Food</label>
- <input class="form-control" type="text" name="food" />
- </div>
- </div>
- </div>
- <div style="clear:both;"></div>
- <div class="modal-footer">
- <button type="button" class="btn btn-danger" data-dismiss="modal"><span class="glyphicon glyphicon-remove"></span> Close</button>
- <button name="save" class="btn btn-primary"><span class="glyphicon glyphicon-save"></span> Save</button>
- </div>
- </div>
- </form>
- </div>
- </div>
- </body>
- <script src="js/jquery-3.2.1.min.js"></script>
- <script src="js/bootstrap.js"></script>
- <script src="js/script.js"></script>
- </html>
Creating PHP Queries
This code contains the php query of the application. This code will try to store the data inputs to the database serve when button is clicked, and then display the data through the select options via ajax request. To do that just copy and write this block of codes inside the text editor, then save it as shown below. save_query,php- <?php
- require_once 'conn.php';
- echo "<script>alert('Data Inserted!')</script>";
- echo "<script>window.location = 'index.php'</script>";
- }else{
- echo "<script>alert('Please complete the required field!')</script>";
- echo "<script>window.location = 'index.php'</script>";
- }
- }
- ?>
- <?php
- require_once 'conn.php';
- $query = $conn->query("SELECT * FROM `food` WHERE `food_category` = '$_REQUEST[food_category]'") or die(mysqli_error());
- echo '<option value = "">Select a food</option>';
- while($fetch = $query->fetchArray()){
- echo '<option value = "'.$fetch['food'].'">'.$fetch['food'].'</option>';
- }
- ?>
Creating The jQuery Script
This is where the code that uses jquery script been used. This code will retrieve the data from the database to display it to select box inputs. To do this just copy and write these block of codes inside the text editor, then save it as script.js inside the js folder.- $(document).ready(function(){
- $('#category').on('change', function(){
- if($('#category').val() == ""){
- $('#food').empty();
- $('<option value = "">Select a food</option>').appendTo('#food');
- $('#food').attr('disabled', 'disabled');
- }else{
- $('#food').removeAttr('disabled', 'disabled');
- $('#food').load('food_get.php?food_category=' + $('#category').val());
- }
- });
- });
Comments
Add new comment
- Add new comment
- 549 views