AngularJS Delete Multiple Rows using PHP/MySQLi
Submitted by nurhodelta_17 on Monday, January 8, 2018 - 19:25.
Getting Started
I've used CDN for Bootstrap and Angular JS so you need internet connection for them to work.Creating our Database
First, we're going to create our database and insert sample data that you can use in deleting. 1. Open phpMyAdmin. 2. Click databases, create a database and name it as angular. 3. After creating a database, click the SQL and paste the below codes. See image below for detailed instruction.- (1, 'Neovic', 'Devierte', 'Silay City'),
- (2, 'Julyn', 'Divinagracia', 'E.B. Magalona'),
- (3, 'Gemalyn', 'Cepe', 'Bohol'),
- (4, 'Matet', 'Devierte', 'Silay City'),
- (5, 'Tintin', 'Devierte', 'Silay City'),
- (6, 'Bien', 'Devierte', 'Cebu City'),
- (7, 'Cherry', 'Ambayec', 'Cebu City'),
- (8, 'Jubilee', 'Limsiaco', 'Silay City'),
- (9, 'Janna ', 'Atienza', 'Talisay City'),
- (10, 'Desire', 'Osorio', 'Bacolod City'),
- (11, 'Debbie', 'Osorio', 'Talisay City'),
- (12, 'Nipoy ', 'Polondaya', 'Victorias City'),
- (13, 'Johnedel', 'Balino', 'Cauyan, Negros'),
- (14, 'Nereca', 'Tajonera', 'Cauayan, Negros'),
- (15, 'Jerome', 'Robles', 'Cebu City');
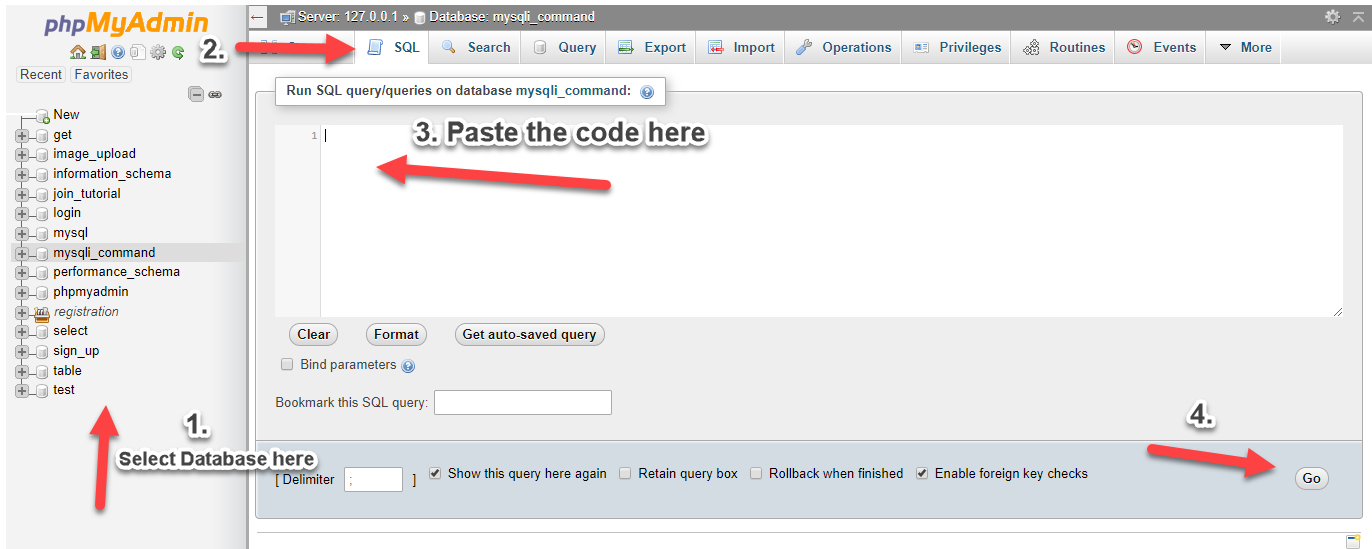
index.html
Next, this is our index that contains our table.- <!DOCTYPE html>
- <html lang="en" ng-app="app">
- <head>
- <meta charset="utf-8">
- <link href="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.7/css/bootstrap.min.css" rel="stylesheet">
- </head>
- <body ng-controller="memberdata" ng-init="fetch()">
- <div class="container">
- <div class="row">
- <div class="col-md-8 col-md-offset-2">
- <div class="alert alert-success text-center" ng-show="success">
- </div>
- <div class="alert alert-danger text-center" ng-show="error">
- </div>
- <table class="table table-bordered table-striped" style="margin-top:10px;">
- <thead>
- <tr>
- </tr>
- </thead>
- <tbody>
- <tr ng-repeat="member in members">
- </tr>
- </tbody>
- </table>
- </div>
- </div>
- </div>
- </div>
- </body>
- </html>
angular.js
This contains our angular.js script.- var app = angular.module('app', []);
- app.controller('memberdata',function($scope, $http){
- $scope.success = false;
- $scope.error = false;
- $scope.fetch = function(){
- $http.get("fetch.php").success(function(data){
- $scope.members = data;
- });
- }
- $scope.toggleAll = function(){
- for (var i = 0; i < $scope.members.length; i++) {
- $scope.members[i].Selected = $scope.checkAll;
- }
- };
- $scope.toggleOne = function(){
- $scope.checkAll = true;
- for (var i = 0; i < $scope.members.length; i++) {
- if (!$scope.members[i].Selected) {
- $scope.checkAll = false;
- break;
- }
- };
- };
- $scope.deleteAll = function(){
- checkedId = [];
- for (var i = 0; i < $scope.members.length; i++) {
- if ($scope.members[i].Selected) {
- checkedId.push($scope.members[i].memid);
- }
- }
- $http.post("delete.php", checkedId)
- .success(function(data){
- console.log(data);
- if(data.error){
- $scope.error = true;
- $scope.success = false;
- $scope.errorMessage = data.message;
- $scope.checkAll = false;
- }
- else{
- $scope.success = true;
- $scope.error = false;
- $scope.successMessage = data.message;
- $scope.fetch();
- $scope.checkAll = false;
- }
- });
- }
- $scope.clearMessage = function(){
- $scope.success = false;
- $scope.error = false;
- }
- });
fetch.php
This is our PHP api/code that fetches table data from our MySQL Database.- <?php
- $conn = new mysqli('localhost', 'root', '', 'angular');
- $sql = "SELECT * FROM members";
- $query=$conn->query($sql);
- while($row=$query->fetch_array()){
- $output[] = $row;
- }
- ?>
delete.php
Lastly, this is our PHP code/api in deleting the checked rows of our table.- <?php
- $conn = new mysqli('localhost', 'root', '', 'angular');
- foreach ($data as $key => $value) {
- $sql = "DELETE FROM members WHERE memid = '".$value."'";
- $query = $conn->query($sql);
- }
- if($query){
- $out['message'] = "Member(s) deleted Successfully";
- }
- else{
- $out['error'] = true;
- $out['message'] = "Something went wrong. Cannot delete Member(s)";
- }
- ?>
Add new comment
- 262 views