JTextField Component in Java
Submitted by donbermoy on Saturday, November 22, 2014 - 23:30.
This is a tutorial in which we will going to create a program that has the JTextField Component using Java. The JTextField is a basic text control component that enables the user to type a small amount of text.
So, now let's start this tutorial!
1. Open JCreator or NetBeans and make a java program with a file name of jTextFieldComponent.java.
2. Import the following packages:
We have created a JTextField component with variable named txtName. The 20 inside the JTextField components indicate the number of characters allowed on the text field.
To create the size of the textfield, we will use the setPreferredSize method and will access the Dimension class.
The 100 is the width of the textfield dimension and 30 is the height.
3. Now, we will add a KeyEvent and KeyListener to the textfield. We will have a text field that if we input text in there, it will automatically converted into uppercase letters. Have this code below:
We have created a text variable for our TextField. The getText method here gets the string input of the user. The setText method will set the text to the textfield and it will be converted into uppercase letters using toUpperCase method.
4. Now, add the JLabel variable to the frame using the default BorderLayout of West position and the JTextField variable using the default BorderLayout of South position of the getContentPane method. Lastly, set the size, visibility, and the close operation of the frame. Have this code below:
Output:
Here's the full code of this tutorial:
For more inquiries and need programmer for your thesis systems in any kind of programming languages, just contact my number below.
Best Regards,
Engr. Lyndon Bermoy
IT Instructor/System Developer/Android Developer/Freelance Programmer
Mobile: 09488225971
Landline: 826-9296
E-mail:[email protected]
Add and Follow me on Facebook: https://www.facebook.com/donzzsky
Visit and like my page on Facebook at: https://www.facebook.com/BermzISware
- textField.setText(text.toUpperCase());
- }
- frame.getContentPane().add(name,"West");
- frame.getContentPane().add(txtName,"South");
- frame.setSize(400, 200);
- frame.setVisible(true);
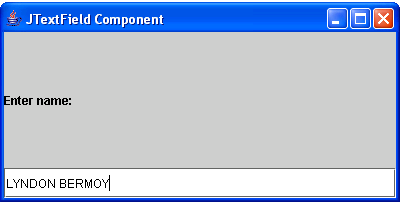
- import java.awt.*; //used to access the Dimension class
- import java.awt.event.*; //used to access the KeyAdapter and KeyEvent class
- import javax.swing.*; //used to access the JFrame, JLabel, and JTextField class
- public class jTextFieldComponent {
- textField.setText(text.toUpperCase());
- }
- });
- frame.getContentPane().add(name,"West");
- frame.getContentPane().add(txtName,"South");
- frame.setSize(400, 200);
- frame.setVisible(true);
- }
- }
Add new comment
- 31 views