How to Create a Quicksort in Terminal using Python
In this tutorial, we will learn how to program Quicksort in Python and implement it in the terminal. The objective is to efficiently sort a list using the Quicksort algorithm. This tutorial will guide you through the process step by step, demonstrating different approaches to sorting arrays. By the end of this tutorial, you will have a solid understanding of how to implement and optimize Quicksort effectively in Python. So, let’s get started!
This topic is straightforward to understand. Just follow the instructions I provide, and you will complete it with ease. The program I will demonstrate will show you the correct and efficient way to implement Quicksort for sorting a list of arrays. By following the step-by-step process, you will gain a clear understanding of how the Quicksort algorithm works, including choosing a pivot, partitioning the array, and recursively sorting subarrays. So, let’s dive into the coding process!
Getting Started:
First you will have to download & install the Python IDLE's, here's the link for the Integrated Development And Learning Environment for Python https://www.python.org/downloads/.
Creating Main Function
This is the main function of the application. The following code will display a simple GUI in terminal console that will display program. To do this, simply copy and paste these blocks of code into the IDLE text editor.- def partition(array, low, high):
- pivot = array[high]
- i = low - 1
- for j in range(low, high):
- if array[j] <= pivot:
- i = i + 1
- (array[i], array[j]) = (array[j], array[i])
- (array[i + 1], array[high]) = (array[high], array[i + 1])
- return i + 1
- def quickSort(array, low, high):
- if low < high:
- pi = partition(array, low, high)
- quickSort(array, low, pi - 1)
- quickSort(array, pi + 1, high)
- while True:
- print("\n================== Create a Quicksort ==================\n")
- data = [1, 6, 3, 1, 10, 9, -3, -2]
- print("Unsorted Array")
- print(data)
- size = len(data)
- quickSort(data, 0, size - 1)
- print('\nSorted Array in Ascending Order:')
- print(data)
- opt = input("\nDo you want to try again?(yes/no): ")
- if opt.lower() == 'yes':
- ret=False
- elif opt.lower() == 'no':
- ret=True
- print("Exiting program....")
- else:
- print("Please enter yes/no:")
- break
- if ret == False:
- continue
This program implements the QuickSort algorithm to sort an array in ascending order. It repeatedly selects a pivot element, partitions the array so that smaller elements are on the left and larger elements are on the right, and then recursively sorts the partitions. The program runs in a loop, displaying an unsorted array, sorting it using QuickSort, and asking the user if they want to repeat the process.
Output:
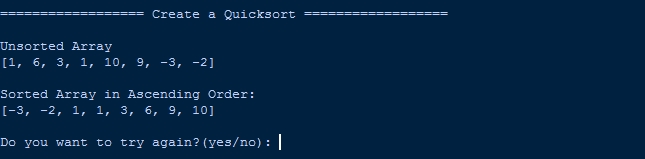
There you have it we successfully created How to Create a Quicksort in Terminal using Python. I hope that this simple tutorial help you to what you are looking for. For more updates and tutorials just kindly visit this site. Enjoy Coding!
More Tutorials for Python Language