C++ Tutorial : Exceptions in C++/CLI
Submitted by Bright777 on Tuesday, February 18, 2014 - 09:16.
Hi :) It’s Bright777 and today I want to show you a mechanism of catching exceptions in C++. First of all you need to know that is the exception how it can be caused. Exception is the action that can’t be completed due to the errors. For example, if you divide some number and zero, you will have an exception.
C++ can help you to catch exceptions. For this purposes C++ has special construction:
As you can see, block try includes the code, which can cause the exception. And block catch() catches the exception. In our example it catches all exception from the block try because catch has parameter(…) . Also you can catch exceptions of class, which you can do by yourself.
Example
Now I will show you an example of program with exception catching mechanism. It is a Windows Forms Application. Program is very simple. In the edit control you need to type a number and press button to show it. If you type something else, it causes the exception. Here the code:
So here you can see the source of the problem: if you assign String value to the variable of type double it causes the exception. So we put it in the block try and after that in the block catch we output the message about the exception (error). In that message we also add full information about the exception, like name, type, where it was caused.
Output of the program
- try
- {
- //code, that can cause the exception
- }
- Catch(…)
- {
- //catching the exception
- }
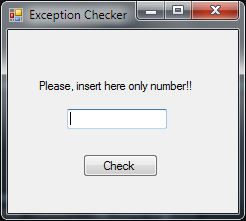
- private: System::Void button1_Click(System::Object^ sender, System::EventArgs^ e) {
- double a;
- try
- {
- a = Convert::ToDouble(textBox1->Text);
- MessageBox::Show("Inserted number: "+a.ToString());
- }
- catch(Exception^ ex)
- {
- MessageBox::Show( "Error!! Program has throwed an exception:\n\n "+ex->GetBaseException(),"Error",MessageBoxButtons::OK,MessageBoxIcon::Error);
- }
- }
- };
- Without exception:
- With exception:
Add new comment
- 24 views