Creating a Paint Program when Moving Mouse using C#
Submitted by donbermoy on Thursday, June 19, 2014 - 16:57.
In this tutorial, i will teach you how to create a program that paints the form when clicking and moving the mouse using C#.
Now, let's start this tutorial!
1. Let's start with creating a Windows Form Application in C# for this tutorial by following the following steps in Microsoft Visual Studio: Go to File, click New Project, and choose Windows Application and name your program as Mouse Move Paint.
2. You don't have to design your interface because we will only focus on the mouse event.
3. Now put this code for your code module.
Output:
For more inquiries and need programmer for your thesis systems in any kind of programming languages, just contact my number below.
Best Regards,
Engr. Lyndon Bermoy
IT Instructor/System Developer/Android Developer/Freelance Programmer
If you have some queries, feel free to contact the number or e-mail below.
Mobile: 09488225971
Landline: 826-9296
E-mail:[email protected]
Add and Follow me on Facebook: https://www.facebook.com/donzzsky
Visit and like my page on Facebook at: https://www.facebook.com/BermzISware
- using System.Diagnostics;
- using System;
- using System.Windows.Forms;
- using System.Collections;
- using System.Drawing;
- using System.Data;
- using System.Collections.Generic;
- namespace Mouse_Move_Paint
- {
- public partial class Form1
- {
- public Form1()
- {
- InitializeComponent();
- }
- public void Form1_Load(System.Object sender, System.EventArgs e)
- {
- // make your cursor as a hand cursor when painting in the form
- Cursor = Cursors.Hand;
- }
- // create a boolean variable named mustPaint and has a False value in the first run of the program
- bool mustPaint = false;
- public void MouseEvent_MouseDown(object sender, System.Windows.Forms.MouseEventArgs e)
- {
- //make mustPaint variable to True when
- mustPaint = true;
- }
- public void MouseEvent_MouseMove(object sender, System.Windows.Forms.MouseEventArgs e)
- {
- //This code is for moving the mouse, when making the mustPaint variable into True
- if (mustPaint)
- {
- // This Graphics class delivers methods for drawing objects to the display form.
- Graphics graphic = CreateGraphics();
- // The graphics variable has the FillEllipse Method which fills the inner of an ellipse defined by a rectangle
- // specified by a pair of coordinates (x and y in our program), a width (that is 10), and a height (5).
- // The SolidBrush Class defines a brush of a single color in which we use Green color.
- }
- }
- public void MouseEvent_MouseUp(object sender, System.Windows.Forms.MouseEventArgs e)
- {
- //make the mustPaint variable into false
- mustPaint = false;
- }
- }
- }
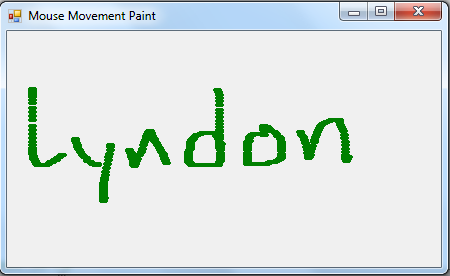
Add new comment
- 223 views