Determine Even or Odd Number in C#
Submitted by donbermoy on Monday, June 16, 2014 - 09:05.
In this tutorial, I'm going to teach you how to create a program that determines if the inputted number is an odd or an even number using C#. This was one of the laboratory exercises in C# for students enrolled in this subject. Even Numbers are any integer that can be divided exactly by 2. The last digit will be 0, 2, 4, 6 or 8. If it is not an even number, it is called an odd number. The last digit will be 1, 3, 5, 7 or 9.
Now, let's start this tutorial!
1. Let's start with creating a Windows Form Application in C# for this tutorial by following the following steps in Microsoft Visual Studio: Go to File, click New Project, and choose Windows Application.
2. Next, add only one Button named Button1 and labeled it as "Determine if Odd or Even Number". Insert also Textbox and named it as Textbox1 for inputting the desired number. You must design your interface like this:
3. Now put this code for your code module. This code is for Button1_Click.
We will have to initialize variable Mynumber as Integer to hold the value inputted in textbox1 as we will use the Int.Parse function in C# that is equivalent to the Val function of VB.NET. The main reason to get the even number is to get their value as divisible by 2 that is equal to zero. So that is why we used the % function. % Operator (the same with Mod statement in VB.NET) divides two numbers and returns only the remainder. The number inputted in textbox1 will be divided by 2 and if the remainder is zero then we considered it as an even number. Otherwise, it is an odd number in our If-Else statement.
Full source code:
Press F5 to run the program.
Output:
Best Regards,
Engr. Lyndon Bermoy
IT Instructor/System Developer/Android Developer/Freelance Programmer
If you have some queries, feel free to contact the number or e-mail below.
Mobile: 09488225971
Landline: 826-9296
E-mail:[email protected]
Add and Follow me on Facebook: https://www.facebook.com/donzzsky
Visit and like my page on Facebook at: https://www.facebook.com/BermzISware
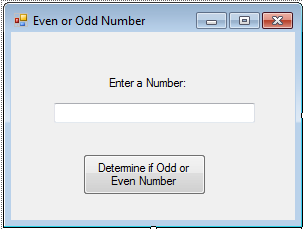
- public void Button1_Click(System.Object sender, System.EventArgs e)
- {
- int Mynumber = default(int);
- Mynumber = (int.Parse(TextBox1.Text));
- if (Mynumber % 2 == 0)
- {
- MessageBox.Show("The number " + " " + Mynumber.ToString() + " is an even number");
- }
- else
- {
- MessageBox.Show(Mynumber.ToString() + " " + "is an Odd number");
- }
- }
- using System.Diagnostics;
- using System;
- using System.Windows.Forms;
- using System.Collections;
- using System.Drawing;
- using System.Data;
- using System.Collections.Generic;
- namespace Even_or_Odd
- {
- public partial class Form1
- {
- public Form1()
- {
- InitializeComponent();
- }
- public void Button1_Click(System.Object sender, System.EventArgs e)
- {
- int Mynumber = default(int);
- Mynumber = (int.Parse(TextBox1.Text));
- if (Mynumber % 2 == 0)
- {
- MessageBox.Show("The number " + " " + Mynumber.ToString() + " is an even number");
- }
- else
- {
- MessageBox.Show(Mynumber.ToString() + " " + "is an Odd number");
- }
- }
- }
- }
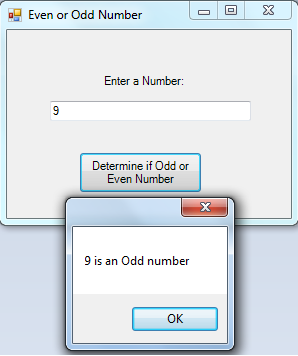
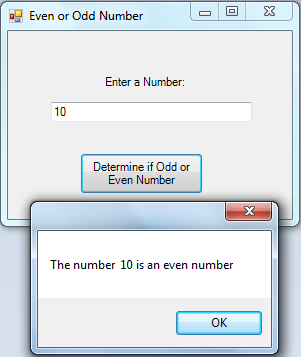
Add new comment
- 1224 views