Simple AutoComplete ComboBox in a DataGridView
Submitted by janobe on Monday, December 23, 2013 - 11:59.
Today I will show you how to create an AutoComplete ComboBox in the DataGridView, add the ComboBox on the DataGridView and add a list of items in the ComboBox programmatically.
Let’s begin:
Open the Visual Basic 2008, create a project and set your Form just like this.
Then, double click the Form and create a code for adding and putting a list of items in the ComboBox on the DataGridView.
Go back to the Design Views, double click the DataGridView and select the method name of the DataGridView in EditingControlShowing. After that, do the code for the AutoComplete ComboBox.
You can download the complete Source Code and run it on your computer.
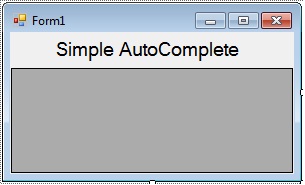
- Private Sub Form1_Load(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles MyBase.Load
- 'Declaring variable as new DataGridViewComboBoxColumn
- 'That represents a column in the datagridview.
- Dim combo As New DataGridViewComboBoxColumn
- 'Putting a list of items in the combobox.
- With combo
- .Items.Add("Mark")
- .Items.Add("Marie")
- .Items.Add("Maybel")
- .Items.Add("Mikmik")
- .Items.Add("Minmin")
- .Items.Add("Mely")
- .Items.Add("Mentor")
- End With
- 'Adding a combobox in the column on the datagridview.
- DataGridView1.Columns.Add(combo)
- End Sub
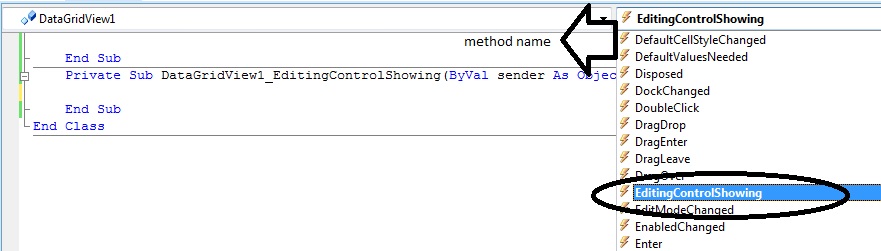
- Private Sub DataGridView1_EditingControlShowing(ByVal sender As Object, ByVal e As System.Windows.Forms.DataGridViewEditingControlShowingEventArgs) Handles DataGridView1.EditingControlShowing
- 'declare variable(cb) as a combobox
- Dim cb As ComboBox
- 'e represent the editing control in the datagridview
- 'the condition is, if the type of e is combobox then set your code for autocomplete
- If TypeOf e.Control Is ComboBox Then
- cb = e.Control
- 'set the dropdown style of a combobox
- cb.DropDownStyle = ComboBoxStyle.DropDown
- 'set the propety of a combobox to autocomplete mode.
- cb.AutoCompleteMode = AutoCompleteMode.SuggestAppend
- cb.AutoCompleteSource = AutoCompleteSource.ListItems
- End If
- End Sub
Add new comment
- 512 views