In this tutorial I will show you how to fill and get the ValueMember of a ComboBox in VB.Net. Knowing the value member of the items that will be displayed in a combo box is very important.Why? For instance, in the
“employee’s table”, you want to display the name of the employee in the combo box, but you want to get the id of it. So, that’s the time to use the value member to get the id of the employee in the combo box.
I used
MS Access for my Database.
Let’s Begin:
1.Open Visual Studio.
2.Create a new project.
3.Make the Form just like this.
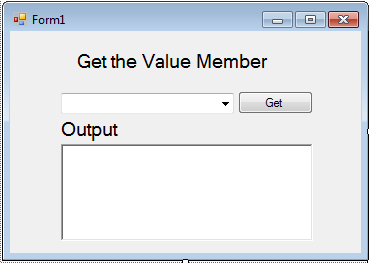
Now, double click the Form. Then, declare all the classes and variables that are needed above the
Form1_Load
.
'DECLARE A STRING VARIABLE TO STORE THE STRING CONNECTION.
Dim strcon As String = "Provider=Microsoft.ACE.OLEDB.12.0;Data Source=" & _
Application.StartupPath & "\userdb.accdb"
'INITIALIZE THE STRING CONNECTION TO BE OPEN
Dim con As OleDb.OleDbConnection = New OleDb.OleDbConnection(strcon)
'INITIALIZE THE COMMANDS THAT ARE USED TO FILL THE DATASET AND UPDATE THE DATA SOURCE
Dim da As New OleDb.OleDbDataAdapter
'INITIALIZE YOUR DATASET
Dim ds As New DataSet
'DECLARE A STRING VARIABLE TO STORE THE QUERY IN IT
Dim sql As String
After that, create a
sub procedure for filling a ComboBox with data that came from the database.
Public Sub cbofill(ByVal sql As String, ByVal cbo As ComboBox)
Try
'OPENING THE CONNECTION
con.Open()
'SET YOUR COMMANDS AND A DATABASE CONNECTION
' THAT ARE USED TO FILL THE DATASET AND UPDATE
' THE DATA SOURCE.
da = New OleDb.OleDbDataAdapter(sql, con)
'SET A NEW DATASET
ds = New DataSet
'ADDING AND REFRESHING THE ROWS OF YOUR DATATABLE
'(FILLING THE DATATABLE)
da.Fill(ds, "userdb")
With cbo
'SET YOUR DATASOURCE TO GET THE
' COLLECTION OF TABLES CONTAINED
' IN THE DATASET
.DataSource = ds.Tables(0)
'SET THE FIELDS OF THE DATATABLE TO
' BE SHOWN ON THE COMBOBOX
.DisplayMember = "username"
'SET THE ACTUAL VALUE OF THE
' ITEMS IN THE COMBOBOX
.ValueMember = "user_id"
End With
Catch ex As Exception
'CATCH ANY ERRORS
End Try
'CLOSING THE CONNECTION
End Sub
In the
Form1_Load
, set your query and call the
sub procedure that you have created.
Private Sub Form1_Load(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles MyBase.Load
'STORE YOUR QUERY IN THE STRING VARIABLE
sql = "SELECT * FROM tbluser"
'CALL YOUR SUB PROCEDURE AND PUT YOUR QUERY IN THE FIRST PARAMETER.
'AND FOR THE SECOND PARAMETER IS THE NAME OF THE COMBOBOX.
cbofill (sql, ComboBox1)
End Sub
In the
click
event handler of a Button, set the selected value of a ComboBox to put it on the RichTextBox.
Private Sub Button1_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles Button1.Click
'PUT THE VALUEMEMBER OF THE COMBOBOX IN THE RICHTEXTBOX
RichTextBox1.Text = "User Id :" & ComboBox1.SelectedValue.ToString
End Sub