Validating a TextBox Using an Error Provider in Visual Basic 2008
Submitted by janobe on Wednesday, April 30, 2014 - 20:45.
Today, I will teach you how to validate a Textbox using an ErrorProvider in Visual Basic 2008. With this, you will know what are the inputs you are going to put in the textbox and it will notify you whatever errors that you might encounter.
Let’s begin:
Open Visual Basic 2008 and create a new windows application. Drag the two TextBoxes, a Button and an Error Provider in the Form. And it will look like this.
After that, double click the Button to fire the
Run your project and click the Button to perform the code in the method.
Output:
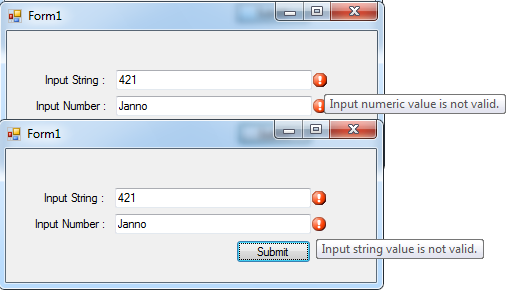
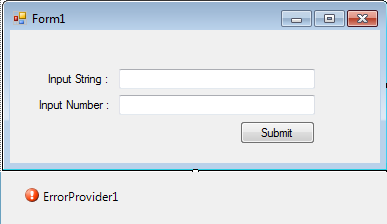
click
event handler and do the following code to the method.
- Private Sub Button1_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles Button1.Click
- 'CHECKING IF THE TWO TEXTBOXES ARE CLEARED
- If txtstring.Text = "" And txtnumber.Text = "" Then
- ErrorProvider1.SetError(txtstring, "Field must be filled up.")
- ErrorProvider1.SetError(txtnumber, "Field must be filled up.")
- Else
- 'THE ERROR PROVIDER WILL BE CLEARED.
- ErrorProvider1.SetError(txtstring, "")
- ErrorProvider1.SetError(txtnumber, "")
- 'CONDITION FOR THE STRING VALUE
- 'CHECK IF THE INPUT IS A NUMERIC VALUE THEN PERFORM THE ERROR PROVIDER
- 'IF NOT, CLEAR THE ERROR PROVIDER.
- 'THE ERROR PROVIDER WILL APPEAR AND WILL NOTIFY THE PROBLEM TO THE USER.
- ErrorProvider1.SetError(txtstring, "Input string value is not valid.")
- Else
- 'THE ERROR PROVIDER WILL BE CLEARED.
- ErrorProvider1.SetError(txtstring, "")
- End If
- 'CONDITION FOR THE NUMERIC VALUE
- 'CHECK IF THE INPUT IS NOT A NUMERIC VALUE THEN PERFORM THE ERROR PROVIDER
- 'IF NUMERIC, CLEAR THE ERROR PROVIDER.
- 'THE ERROR PROVIDER WILL APPEAR AND WILL NOTIFY THE PROBLEM TO THE USER.
- ErrorProvider1.SetError(txtnumber, "Input numeric value is not valid.")
- Else
- 'THE ERROR PROVIDER WILL BE CLEARED.
- ErrorProvider1.SetError(txtnumber, "")
- End If
- End If
- End Sub
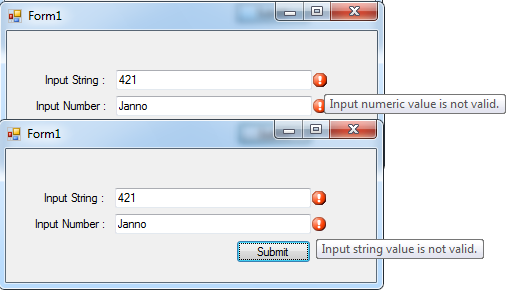
Add new comment
- 251 views