Creating a Slot Machine Program in VB.NET
This is my another tutorial in VB.NET. In this tutorial we add three picture boxes, a timer, a button and a label. Set the timer interval to 10, which means the images will refresh every 0.01 second. In the code, we shall introduce four variables m,a, b and c, where m is used to stop the timer and a,b,c are used to generate random images using the syntax Int(1 + Rnd() * 3).
Getting Started
First thing you need to do is Download and Install Microsoft Visual Studio.
Then, download or save the images below in your vb.net program.
This is used as your default image in your three picture boxes.
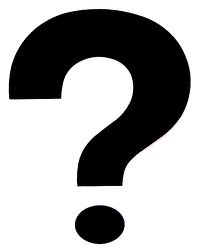
These are the three images for our slot machines. The apple, grapes, and strawberry image.
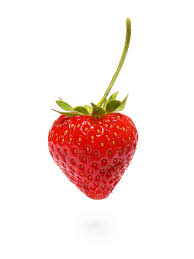
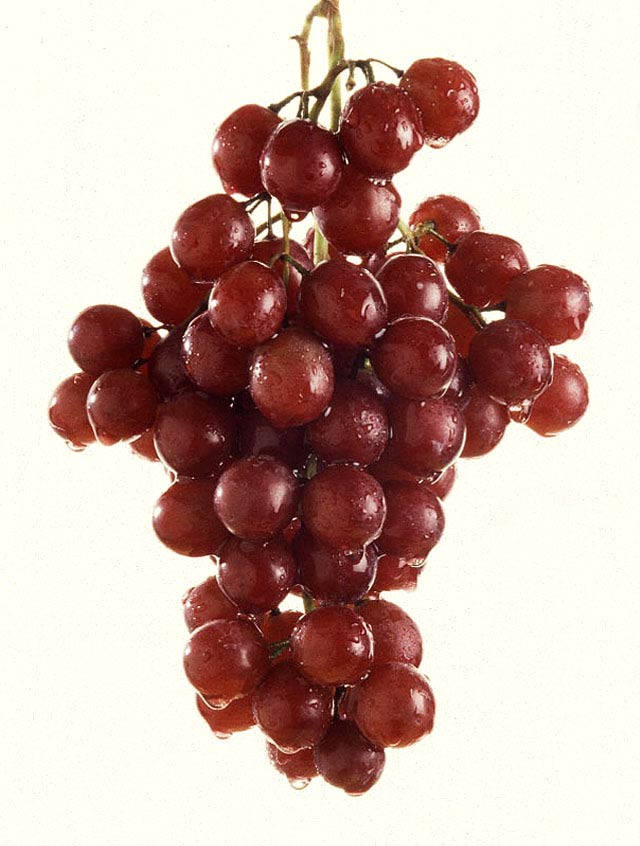
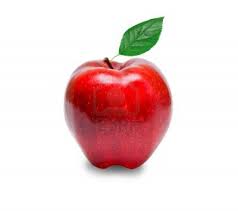
To load the images, we use the following syntax:
- PictureBox.Image = Image.FromFile(Path of the image file)
Your design of the program must be like this image below.

Setting Integer Variables
We need to declare the variables of the integers for Image values and the timer interval. Below code is a declared variables for, m = Timer Interval and a,b, and c are the images values variables.
- Public Class Form1
- Dim m, a, b, c As Integer
- End Class
Creating a Click Event
Below code is a script to spin the slot machine.
- Private Sub Button1_Click(sender As Object, e As EventArgs) Handles Button1.Click
- 'Removing the text value of the label
- lblMsg.Text = ""
- 'Starting the timer
- Timer1.Enabled = True
- 'Disable the spin button
- Button1.Enabled = False
- End Sub
Creating a Random Value for the Picturebox
Below code is the script to randomize the picture boxes images every the 0.01 seconds. Each picture box will stop spinning every 3 seconds which means the slot machine will be done randomizing after 9 seconds and display the text result in the label field provided.
- Private Sub Timer1_Tick(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles Timer1.Tick
- m = m + 10
- If m < 3000 Then
- If m < 1000 Then
- Select Case a
- Case 1
- PictureBox1.Image = Image.FromFile("C:\Users\Personal-01\Desktop\sample images\slot machine\apple.jpg")
- Case 2
- PictureBox1.Image = Image.FromFile("C:\Users\Personal-01\Desktop\sample images\slot machine\grapes.jpg")
- Case 3
- PictureBox1.Image = Image.FromFile("C:\Users\Personal-01\Desktop\sample images\slot machine\strawberry.jpg")
- End Select
- End If
- If m < 2000 Then
- Select Case b
- Case 1
- PictureBox2.Image = Image.FromFile("C:\Users\Personal-01\Desktop\sample images\slot machine\apple.jpg")
- Case 2
- PictureBox2.Image = Image.FromFile("C:\Users\Personal-01\Desktop\sample images\slot machine\grapes.jpg")
- Case 3
- PictureBox2.Image = Image.FromFile("C:\Users\Personal-01\Desktop\sample images\slot machine\strawberry.jpg")
- End Select
- End If
- If m < 3000 Then
- Select Case c
- Case 1
- PictureBox3.Image = Image.FromFile("C:\Users\Personal-01\Desktop\sample images\slot machine\apple.jpg")
- Case 2
- PictureBox3.Image = Image.FromFile("C:\Users\Personal-01\Desktop\sample images\slot machine\grapes.jpg")
- Case 3
- PictureBox3.Image = Image.FromFile("C:\Users\Personal-01\Desktop\sample images\slot machine\strawberry.jpg")
- End Select
- End If
- Else
- Timer1.Enabled = False
- Button1.Enabled = True
- m = 0
- If a = b And a = c And b = c Then
- lblMsg.Text = "Jackpot! You won P1,000,000″"
- lblMsg.ForeColor = Color.Green
- 'MessageBox.Show($"{a} {b} {c} Equal")
- Else
- lblMsg.Text = "No luck, try again"
- lblMsg.ForeColor = Color.Red
- 'MessageBox.Show($"{a} {b} {c} Unequal")
- End If
- End If
- End Sub
We employ the If…Then structure to control the timer and the Select Case…..End Select structure to generate the random images. The label is used to display the message of the outcomes.
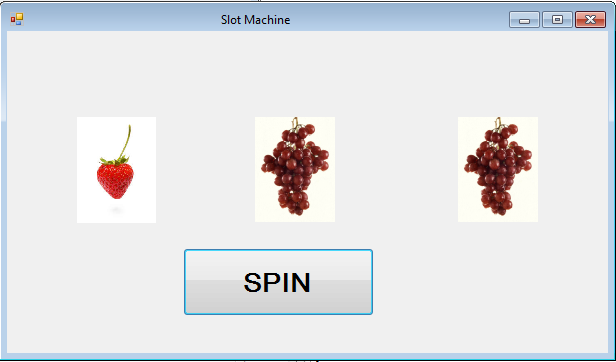
Result
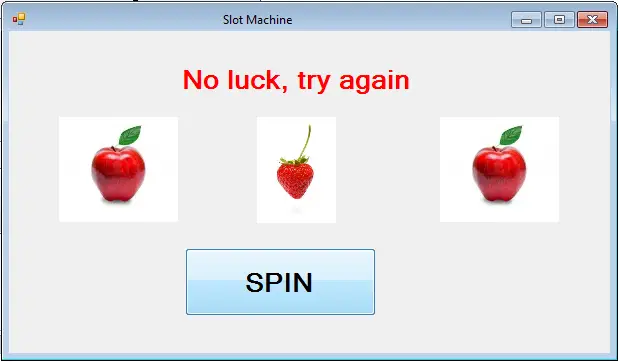
That's it you have now a Simple Slot Machine using Vb.NET. Take note that you have to configure the images location according to path where you have saved the images. "Image.FromFile("C:\Users\Personal-01\Desktop\sample images\slot machine\strawberry.jpg")"
Here's the full code:
- Public Class Form1
- Dim m, a, b, c As Integer
- Private Sub Button1_Click(sender As Object, e As EventArgs) Handles Button1.Click
- lblMsg.Text = ""
- lblMsg.ForeColor = Color.Blue
- Timer1.Enabled = True
- Button1.Enabled = False
- End Sub
- Private Sub Timer1_Tick(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles Timer1.Tick
- m = m + 10
- If m < 3000 Then
- If m < 1000 Then
- Select Case a
- Case 1
- PictureBox1.Image = Image.FromFile("C:\Users\Personal-01\Desktop\sample images\slot machine\apple.jpg")
- Case 2
- PictureBox1.Image = Image.FromFile("C:\Users\Personal-01\Desktop\sample images\slot machine\grapes.jpg")
- Case 3
- PictureBox1.Image = Image.FromFile("C:\Users\Personal-01\Desktop\sample images\slot machine\strawberry.jpg")
- End Select
- End If
- If m < 2000 Then
- Select Case b
- Case 1
- PictureBox2.Image = Image.FromFile("C:\Users\Personal-01\Desktop\sample images\slot machine\apple.jpg")
- Case 2
- PictureBox2.Image = Image.FromFile("C:\Users\Personal-01\Desktop\sample images\slot machine\grapes.jpg")
- Case 3
- PictureBox2.Image = Image.FromFile("C:\Users\Personal-01\Desktop\sample images\slot machine\strawberry.jpg")
- End Select
- End If
- If m < 3000 Then
- Select Case c
- Case 1
- PictureBox3.Image = Image.FromFile("C:\Users\Personal-01\Desktop\sample images\slot machine\apple.jpg")
- Case 2
- PictureBox3.Image = Image.FromFile("C:\Users\Personal-01\Desktop\sample images\slot machine\grapes.jpg")
- Case 3
- PictureBox3.Image = Image.FromFile("C:\Users\Personal-01\Desktop\sample images\slot machine\strawberry.jpg")
- End Select
- End If
- Else
- Timer1.Enabled = False
- Button1.Enabled = True
- m = 0
- If a = b And a = c And b = c Then
- lblMsg.Text = "Jackpot! You won P1,000,000″"
- lblMsg.ForeColor = Color.Green
- 'MessageBox.Show($"{a} {b} {c} Equal")
- Else
- lblMsg.Text = "No luck, try again"
- lblMsg.ForeColor = Color.Red
- 'MessageBox.Show($"{a} {b} {c} Unequal")
- End If
- End If
- End Sub
- End Class
Demo
For more inquiries and need programmer for your thesis systems in any kind of programming languages, just contact my number below.
Best Regards,
Engr. Lyndon R. Bermoy
IT Instructor/System Developer/Android Developer
Mobile: 09079373999
Telephone: 826-9296
E-mail:[email protected]
Visit and like my page on Facebook at: Bermz ISware Solutions
Subscribe at my YouTube Channel at: SerBermz
Comments
Add new comment
- Add new comment
- 7832 views