Blog Post Slider
Submitted by rinvizle on Friday, August 5, 2016 - 14:14.
In this tutorial we are going to create a Blog Post Slider that shows the latest post of your favorite blogs. We will be using jQuery, PHP and XSL. We will apply XSL to transform the feed into HTML and cache the result for subsequent use. We will be using a lot of CSS3 properties for a polished look.
index.html - or the mark up.
Hope that you learn in this tutorial and enjoy coding. Don't forget to LIKE & SHARE this website.
Sample Code
Class.php- <?php
- $source = $_GET['source'];
- $rss = new RSS($source);
- $rss->getLatest();
- class RSS{
- private $cache_dir = 'cache';
- private $cache_lifetime = 300;
- private $source;
- private $xsl_file = 'html.xsl';
- public function __construct($source){
- $this->source = $source;
- }
- public function getLatest(){
- switch($this->source){
- case 'PHP':
- $xml = 'http://www.sourcecodester.com/php';
- break;
- case 'C':
- $xml = 'http://www.sourcecodester.com/cpp';
- break;
- case 'PYTHON':
- $xml = 'http://www.sourcecodester.com/other';
- break;
- case 'JAVASCRIPT':
- $xml = 'http://www.sourcecodester.com/javascript';
- break;
- case 'CC':
- $xml = 'http://www.sourcecodester.com/c-sharp';
- break;
- case 'CPROGRAMMING':
- $xml = 'http://www.sourcecodester.com/cpp';
- break;
- case 'JAVA':
- $xml = 'http://www.sourcecodester.com/java';
- break;
- case 'RUBY':
- $xml = 'http://www.sourcecodester.com/other';
- break;
- case 'SQL':
- $xml = 'http://www.sourcecodester.com/sql';
- break;
- }
- if($this->isCached($this->source . '.xml')){
- echo $this->getCache($this->source . '.xml');
- }
- }
- private function addCache($content, $file){
- $directory = $this->cache_dir;
- throw new Exception("Unable to write to cache");
- }
- }
- private function getCache($file){
- }
- public function isCached($file){
- $isCached = true;
- }
- }
- }
- }
- ?>
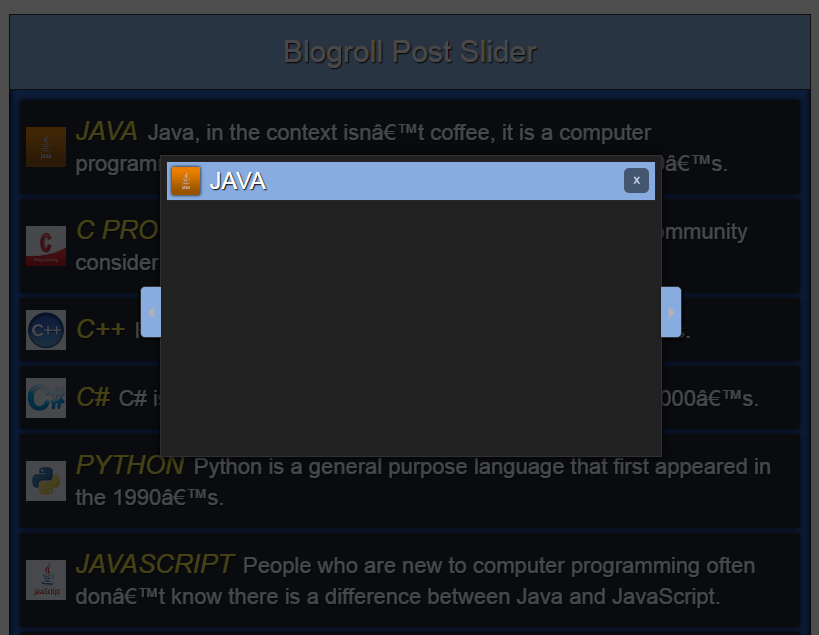
- <!DOCTYPE html>
- <html>
- <head>
- <link rel="stylesheet" href="css/style.css" type="text/css" media="screen"/>
- </head>
- <body>
- <div id="modal" class="modal" style="display:none;">
- </div>
- <div class="content">
- <ul id="friendsList" class="friendsList">
- </ul>
- </div>
- <script type="text/javascript">
- $(function() {
- $.ajaxSetup({cache: false});
- var current = -1;
- var total = $('#friendsList').children().length;
- $('#friendsList a').bind('click',function(e){
- var $this = $(this);
- show();
- var $elem = $this.parent();
- current = $elem.index() + 1;
- var source = $elem.attr('class');
- $('#blog_info').empty()
- $.get('class.php', {source:source} , function(data) {
- $('#latest_post').removeClass('loading').html(data);
- });
- e.preventDefault();
- });
- function show(){
- $('#overlay').show();
- if(!$('#modal').is(':visible'))
- $('#modal').css('left','-260px')
- .show()
- .stop()
- .animate({'left':'50%'}, 500);
- }
- function hide(){
- $('#modal').stop()
- .animate({'left':'150%'}, 500, function(){
- $(this).hide();
- $('#overlay').hide();
- $('#latest_post').empty();
- });
- }
- $('#modal .close').bind('click',function(){
- hide();
- });
- $('#modal .next').bind('click',function(e){
- if(current == total){
- e.preventDefault();
- return;
- }
- $('#latest_post').empty().addClass('loading');
- $('#friendsList li:nth-child('+ parseInt(current+1) +')').find('a').trigger('click');
- e.preventDefault();
- });
- $('#modal .prev').bind('click',function(e){
- if(current == 1){
- e.preventDefault();
- return;
- }
- $('#latest_post').empty().addClass('loading');
- $('#friendsList li:nth-child('+ parseInt(current-1) +')').find('a').trigger('click');
- e.preventDefault();
- });
- });
- </script>
- </body>
- </html>
Add new comment
- 218 views