TUTORIAL NO 1
Your First JAVA Game
In this tutorial you will learn:
1. Event handling
2. JAVA awt basics
3. JAVA swing basics
4. Intefaces
This program will guide you how to make your own very basic JAVA game. We are going to call this game CLICK ME IF YOU CAN.
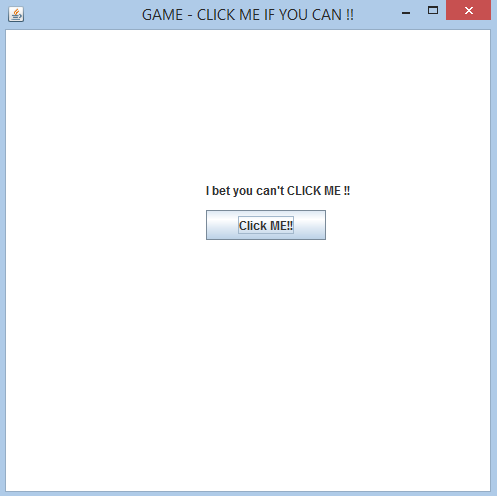
In this tutorial we will be working in JAVA SWING. The first thing that we are going to do is setting up a JFrame and adding the required panels and Components. We will construct everything without the use of visual designers.
Basic step:
Download and install ECLIPSE and set up a JAVA PROJECT. Then Create a new class and name it ClickMe. Then follow the steps
1.IMPORT STATEMENTS
First of all write these import statements in your .java file
import java.awt.Color;
import java.awt.event.*;
import javax.swing.*;
import java.util.*;
Here the two most important statement are java.awt.event.*; and javax.swing.*; . The function of the event statement is to handle what to do when the mouse button is clicked or is near the click me button. The swing statement is for creating the components making JFrame and JButton.
2.SETTING UP THE CLASS
{
String msg1
= "I bet you can't CLICK ME !!";
Now we are declaring our public class and extending it from JPanel because we will add every thing in a panel then add that panel in the frame. Secondly we are implementing the MouseListener interface to respond to mouse hover or mouse click. After that we are creating objects for JFrame , JPanel , JButton and Random class object ( for random positioning).
3.SETTING THE FRAME
public ClickMe()
{
jf.setSize(500, 500); //setting frame size
jf.
setDefaultCloseOperation(JFrame.
EXIT_ON_CLOSE); //for closing
jf.setTitle("GAME - CLICK ME IF YOU CAN !!"); //setting title
jf.setVisible(true); // setting the frame to visible
jp.
setBackground(Color.
WHITE); //setting the panel color to white
}
Creating a constructor and setting the frame size , close operation , title and visibility. In the end setting the panel color to white (Note that jp is the panel object).
4.SETTING THE COMPONENTS
public void setComponents()
{
jf.add(jp); //adding panel in the frame
jp.setLayout(null); // null layout to move button freely in a frame
label.setBounds(200, 150, 200, 20); //setting the label
jp.add(label); //adding label
jp.setBounds(0, 0, 500, 500); // setting panel boundaries
jp.add(jb); //adding button in panel
jb.setBounds(200,180, 120, 30); //positioning button in panel
jb.addMouseListener(this); // adding mouselistener to the button
}
First of all making a setComponents function and adding the panel in the frame then setting its layout to null. After that add the the label, then add the button in the panel with and call the mouse listener for the button (In addMouseListener(this) indicates it’s present in this class).
5.OVERRIDING MOUSE ENTERED FUNCTION
@Override
{
if(me.getSource() == jb) //checking if mouse entered jbutton
jb.setBounds(rand.nextInt(jf.getWidth()-100),rand.nextInt(jf.getHeight()-100),120,30); // randomizing position
}
Here we are overriding the mouseEntered(MouseEvent me) function which is defined in the MouseListener interface. Then we are checking whether the cursor entered the boundary of jb (which is our JButton) or not. If the cursor has entered we immediately randomize the x axis and y axis of the button, and we are using jf.getwidth() -100 to auto adjust it according to the screen size e.g when you press the maximize button.
6. PROVIDING NULL BODY IMPLEMENTATION TO THE REMAINING METHODS
/* providing null body implementation for the
* remaining abstract methods of mouselistener interface
*/
7. WRITING MAIN FUNCTION AND COMPLETING THE APP
public static void main
(String[] args
)
{
ClickMe cm = new ClickMe(); // making the class object
cm.setComponents(); // call the setComponents function
}//end main
}//end class
In the end writing the main function and creating the object of our Clickme class using the constructor (REMEMBER we have already set up the frame in the constructor). When the object is created constructor is called automatically and the frame will be set up because we have added the frame set up code in the constructor. Then call the setComponents function to add the button and the label. Close main and then the class. Here your first simple GAME- CLICK ME IF YOU CAN IS COMPLETE.
OUTPUT:
Button moves here and there when you bring your mouse near it.