Get the Pressed Key using C#
Submitted by donbermoy on Saturday, June 14, 2014 - 13:27.
In this tutorial, i will teach you how to create a program that will capture, obtain, or get a key pressed by the user.
Now, let's start this tutorial!
1. Let's start with creating a Windows Form Application in C# for this tutorial by following the following steps in Microsoft Visual Studio: Go to File, click New Project, and choose Windows Application and name your project as Get the Pressed Key.
We don't need to design an interface because we have to get only the pressed key by the user.
2. Now put this code for your code module.
We choose the Form control and its Keypress event to have the pressed key obtained. Keypress event occurs when the user presses and releases a key. You can use the KeyPress event for intercepting keystrokes entered in a control.
We will have to create an If Statement and Strings.Asc(e.KeyChar) > 1 which means that there is a pressed key done by the user. Asc Function returns an integer representing the character code corresponding to the first letter in a string. Variable e was used as a form of keychar as this variable is a variable for KeyPress Events arguments.
Then, display the message to get the pressed key by using e.KeyChar. e.KeyChar gets or sets the character corresponding to the key pressed.
Keep e.Handled to be equal to True. e.Handled gets or sets a value indicating whether the event was handled and returns boolean.
Full source code:
Output:
Best Regards,
Engr. Lyndon Bermoy
IT Instructor/System Developer/Android Developer/Freelance Programmer
If you have some queries, feel free to contact the number or e-mail below.
Mobile: 09488225971
Landline: 826-9296
E-mail:[email protected]
Add and Follow me on Facebook: https://www.facebook.com/donzzsky
Visit and like my page on Facebook at: https://www.facebook.com/BermzISware
- public void Form1_KeyPress(object sender, System.Windows.Forms.KeyPressEventArgs e)
- if (Strings.Asc(e.KeyChar) > 1)
- {
- MessageBox.Show("You have pressed " + e.KeyChar + " key");
- e.Handled = true;
- using System.Diagnostics;
- using System;
- using System.Windows.Forms;
- using System.Collections;
- using System.Drawing;
- using System.Data;
- using System.Collections.Generic;
- namespace Get_the_pressed_key
- {
- public partial class Form1
- {
- public Form1()
- {
- InitializeComponent();
- }
- public void Form1_KeyPress(object sender, System.Windows.Forms.KeyPressEventArgs e)
- {
- if (Strings.Asc(e.KeyChar) > 1)
- {
- MessageBox.Show("You have pressed " + e.KeyChar + " key");
- e.Handled = true;
- }
- }
- }
- }
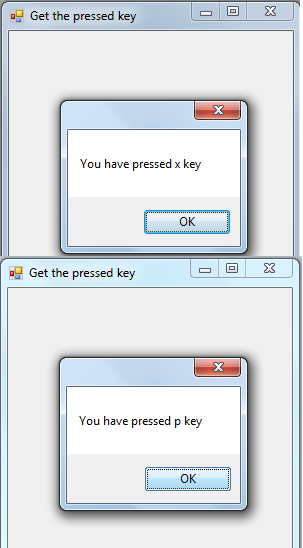
Add new comment
- 548 views