Get and Display All Installed Fonts using C#
Submitted by donbermoy on Tuesday, June 10, 2014 - 16:09.
This is a continuation of my other tutorial in C# entitled Get and Display all COM Ports using C#. But here in this tutorial, we will going to create a program that will get and display the installed fonts in your computer.
So, now let's start this tutorial!
1. Let's start with creating a Windows Form Application in C# for this tutorial by following the following steps in Microsoft Visual Studio: Go to File, click New Project, and choose Windows Application.
2. Next, add only one ListBox named ListBox1 that can hold the values of our installed fonts. Then add a button named Button1 labeled as "Get Fonts" for us to display the fonts in the ListBox. You must design your interface like this:
3. Now, in your code tab, use the namespace
4. Now, put this code in Button1_Click. This will trigger to put all the installed fonts in the ListBox.
We will initialize f as a variable which will hold and instantiate all the installed font values using the InstalledFontCollection method.
Next, we will have a code using For Each Loop statement so that all the fonts will be displayed in the ListBox. We will have the syntax of For Each font As FontFamily In f.Families which means that we declare variable font as the FontFamily of the f as the InstalledFontColletion. Then all the fonts that was looped is displayed in our ListBox1.
Full source code:
Output:
Best Regards,
Engr. Lyndon Bermoy
IT Instructor/System Developer/Android Developer/Freelance Programmer
If you have some queries, feel free to contact the number or e-mail below.
Mobile: 09488225971
Landline: 826-9296
E-mail:[email protected]
Add and Follow me on Facebook: https://www.facebook.com/donzzsky
Visit and like my page on Facebook at: https://www.facebook.com/BermzISware
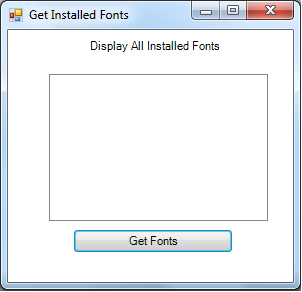
System.Drawing.Text
and put it to the top most part so that it can call the InstalledFontCollection method.
- using System.Windows.Forms;
- using System.Collections;
- using System.Drawing;
- using System.Data;
- using System.Collections.Generic;
- using System.Drawing.Text;
- foreach (FontFamily font in f.Families)
- {
- ListBox1.Items.Add(font.Name);
- }
- using System.Diagnostics;
- using System;
- using System.Windows.Forms;
- using System.Collections;
- using System.Drawing;
- using System.Data;
- using System.Collections.Generic;
- using System.Drawing.Text;
- namespace Get_Installed_Fonts
- {
- public partial class Form1
- {
- public Form1()
- {
- InitializeComponent();
- }
- public void Button1_Click(System.Object sender, System.EventArgs e)
- {
- foreach (FontFamily font in f.Families)
- {
- ListBox1.Items.Add(font.Name);
- }
- }
- }
- }
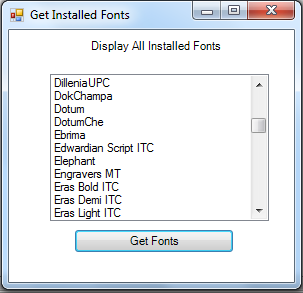
Add new comment
- 115 views