How to Create a Simple Search Based on RadioButton in C# and MySQL Database
Submitted by janobe on Friday, June 28, 2019 - 16:34.
In this tutorial, I will teach you how to create a simple search based on radiobutton using c# and mysql database. This method has the ability to select one option from a group that is used to control the data, then it will be displayed in the datagriview. Follow the step by step guide to see how it works.
The complete source code is included, you can download it and run it on your computer.
For any questions about this article. You can contact me @
Email – [email protected]
Mobile No. – 09305235027 – TNT
Or feel free to comment below.
Creating Application
Step 1
Open Microsoft Visual Studio 2015 and create a new windows form application for c#.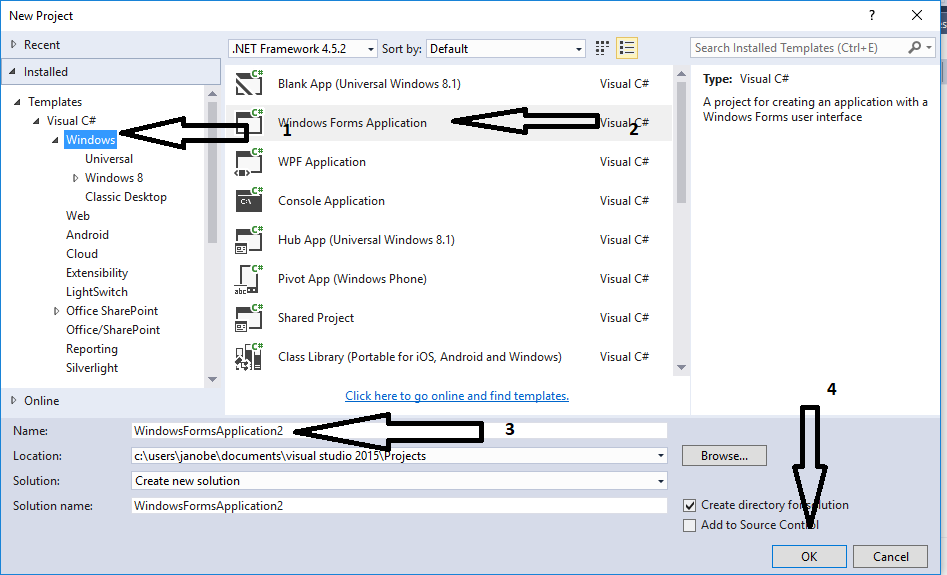
Step 2
Do the form just like shown below.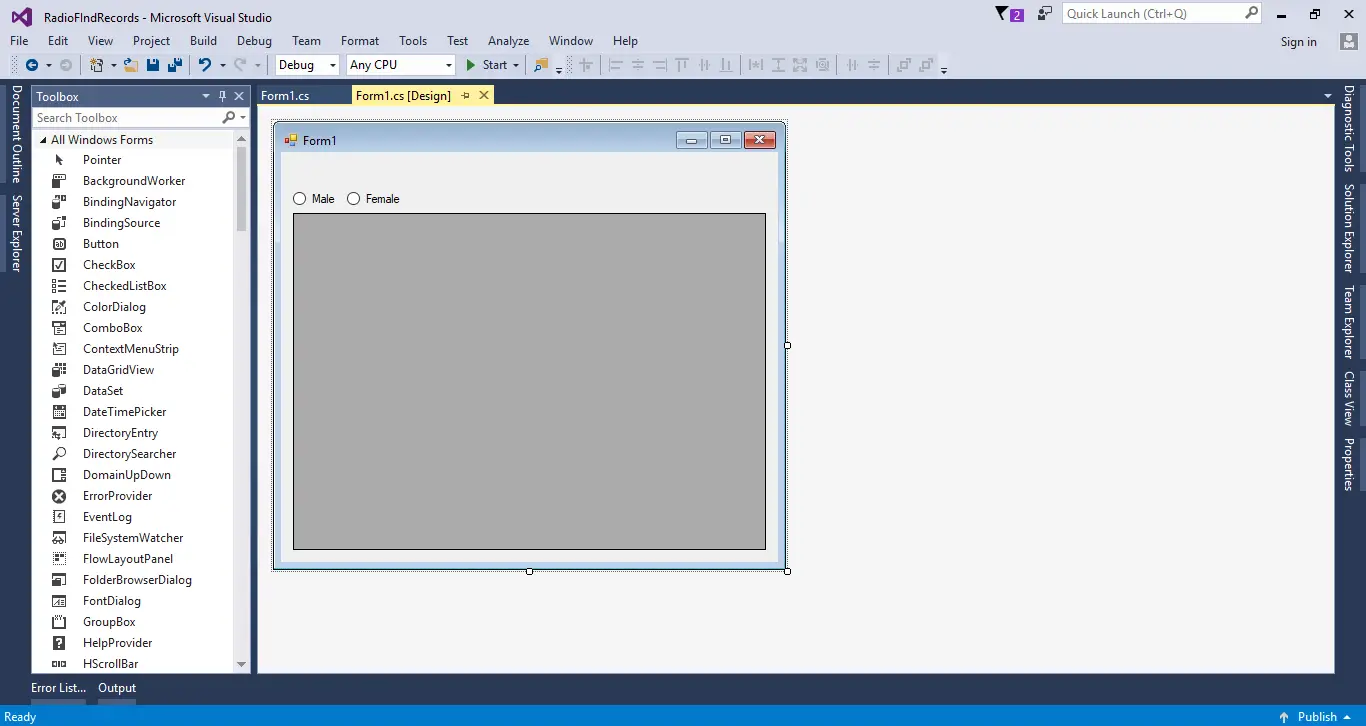
Step 3
Open the code editor by pressing the F7 on the keyboard. In the code editor, add a namespace to access MySQL Libraries.- using MySql.Data.MySqlClient;
Step 4
Create a connection between C# and MySQL Database. After that, declare all the classes that are needed.- MySqlConnection con = new MySqlConnection("server=localhost;user id=root;password=;database=profile;sslMode=none");
- MySqlCommand cmd;
- MySqlDataAdapter da;
- DataTable dt;
- string sql;
Step 5
Create a method for retrieving data in the database and it will be displayed inside the datagridview.- private void LoadData(string sql,DataGridView dtg)
- {
- try
- {
- con.Open();
- cmd.Connection = con;
- cmd.CommandText = sql;
- da.SelectCommand = cmd;
- da.Fill(dt);
- dtg.DataSource = dt;
- }
- catch(Exception ex)
- {
- MessageBox.Show(ex.Message);
- }
- finally
- {
- con.Close();
- da.Dispose();
- }
- }
Step 6
Write the following code for retrieving data in the database in the first load of the form.- private void Form1_Load(object sender, EventArgs e)
- {
- sql = "SELECT * FROM `profile`";
- LoadData(sql, dataGridView1);
- }
Step 7
Write this code for finding data in the database.- private void rdoFemale_CheckedChanged(object sender, EventArgs e)
- {
- sql = "SELECT * FROM `profile` WHERE `gender`='" + rdoFemale.Text + "'";
- LoadData(sql, dataGridView1);
- }
- private void rdoMale_CheckedChanged(object sender, EventArgs e)
- {
- sql = "SELECT * FROM `profile` WHERE `gender`='" + rdoMale.Text + "'";
- LoadData(sql, dataGridView1);
- }
Add new comment
- 169 views