How to Validate a Registration Form Using Regular Expression in C#
Submitted by janobe on Friday, July 22, 2016 - 09:15.
In this tutorial, I will teach you how to validate a Registration Form using Regular Expression in C#. With this method, you can restrict all the textboxes in a form.It is also a guide for all users when they're going to fill up a registration form.
Go to the Solution Explorer, click the “View Code” to make the code editor appear.
In the code editor, put the "
After that, do the following codes for formating the specific fields using the correct pattern.
Go back to the design view, double-click the button and do the following codes to validate the registration form.
For all students who need a programmer for your thesis system or anyone who needs a source code in any programming languages. You can contact me @ :
Email – [email protected]
Mobile No. – 09305235027 – tnt
Let’s get started:
Open Microsoft Visual Studio 2008 and create new Windows Form Application for C#. Then, do the following Form as follows.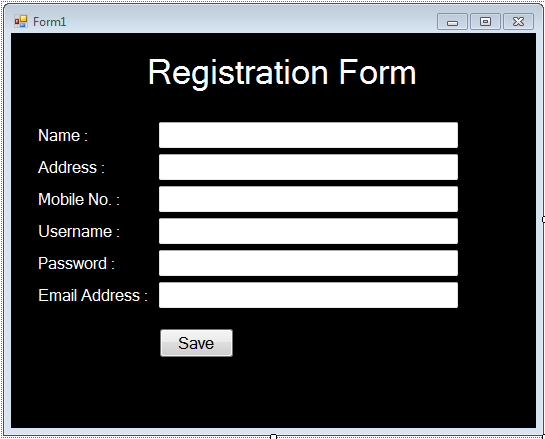
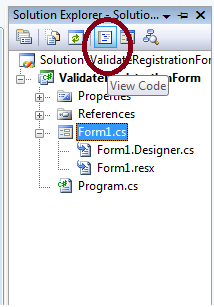
using System.Text.RegularExpressions;
" above the namespace to access regular expression library.
- //import the regular expression library
- using System.Text.RegularExpressions;
- //initialize the validating method
- static Regex Valid_Name = StringOnly();
- static Regex Valid_Contact = NumbersOnly();
- static Regex Valid_Password = ValidPassword();
- static Regex Valid_Email = Email_Address();
- //Method for validating email address
- private static Regex Email_Address()
- {
- string Email_Pattern = @"^(?!\.)(""([^""\r\\]|\\[""\r\\])*""|"
- + @"([-a-z0-9!#$%&'*+/=?^_`{|}~]|(?<!\.)\.)*)(?<!\.)"
- + @"@[a-z0-9][\w\.-]*[a-z0-9]\.[a-z][a-z\.]*[a-z]$";
- }
- //Method for string validation only
- private static Regex StringOnly()
- {
- string StringAndNumber_Pattern = "^[a-zA-Z]";
- }
- //Method for numbers validation only
- private static Regex NumbersOnly()
- {
- string StringAndNumber_Pattern = "^[0-9]*$";
- }
- //Method for password validation only
- private static Regex ValidPassword()
- {
- string Password_Pattern = "(?!^[0-9]*$)(?!^[a-zA-Z]*$)^([a-zA-Z0-9]{8,15})$";
- }
- //Validate all textboxes when the button is clicked
- private void btnSave_Click_1(object sender, EventArgs e)
- {
- //for Name
- if (Valid_Name.IsMatch(txtName.Text) != true)
- {
- MessageBox.Show("Namme accepts only alphabetical characters", "Invalid", MessageBoxButtons.OK, MessageBoxIcon.Exclamation);
- txtName.Focus();
- return;
- }
- //for Address
- if (txtAddress.Text == "")
- {
- MessageBox.Show("Address cannot be empty!", "Invalid", MessageBoxButtons.OK, MessageBoxIcon.Exclamation);
- txtAddress.Focus();
- return;
- }
- //for Contacts
- if (Valid_Contact.IsMatch(txtContactNo.Text) != true)
- {
- MessageBox.Show("Contact accept numbers only.", "Invalid", MessageBoxButtons.OK, MessageBoxIcon.Exclamation);
- txtContactNo.Focus();
- return;
- }
- //for username
- if (txtUsername.Text == "")
- {
- MessageBox.Show("Username cannot be empty!", "Invalid", MessageBoxButtons.OK, MessageBoxIcon.Exclamation);
- txtUsername.Focus();
- return;
- }
- //for password
- if (Valid_Password.IsMatch(txtPassword.Text) != true)
- {
- MessageBox.Show("Password must be atleast 8 to 15 characters. It contains atleast one Upper case and numbers.", "Invalid", MessageBoxButtons.OK, MessageBoxIcon.Exclamation);
- txtPassword.Focus();
- return;
- }
- //for Email Address
- if (Valid_Email.IsMatch(txtEmailAddress.Text) != true)
- {
- MessageBox.Show("Invalid Email Address!","Invalid",MessageBoxButtons.OK ,MessageBoxIcon.Exclamation );
- txtEmailAddress.Focus();
- return;
- }
- //success message
- MessageBox.Show("You are now successfully registered.");
- //hidding all object in the form
- foreach(Control txt in this.Controls)
- {
- {
- txt.Visible = false;
- }
- }
- }
Add new comment
- 1537 views