How to Convert String to Datetime in Python
In this tutorial, we’ll learn how to program "How to Convert a String to Datetime in Python." The objective is to safely convert a string into a datetime format. This tutorial will demonstrate the process of transforming a string data type into a datetime format using Python's built-in libraries. A sample program will be provided to guide you through the implementation. So, let’s get started!
This topic is simple to understand. Just follow the instructions I provide, and you’ll be able to complete it with ease. The program I’ll demonstrate will show you the correct way to convert a string into a datetime format. So, let’s start coding!
Getting Started:
First you will have to download & install the Python IDLE's, here's the link for the Integrated Development And Learning Environment for Python https://www.python.org/downloads/.
Creating Main Function
This is the main function of the application. The following code will display a simple GUI in terminal console that will display program. To do this, simply copy and paste these blocks of code into the IDLE text editor.- from datetime import datetime
- while True:
- print("\n================= Convert String to Datetime =================\n\n")
- my_date_string = "Dec 29 2024 10:30PM"
- datetime_object = datetime.strptime(my_date_string, '%b %d %Y %I:%M%p')
- print(datetime_object)
- opt = input("\nDo you want to try again?(yes/no): ")
- if opt.lower() == 'yes':
- ret=False
- elif opt.lower() == 'no':
- ret=True
- print("Exiting program....")
- else:
- print("Please enter yes/no:")
- break
- if ret == False:
- continue
Output:
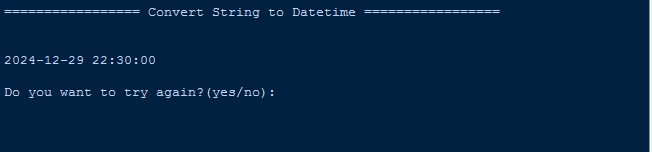
There you have it we successfully created How to Convert String to Datetime in Python. I hope that this simple tutorial help you to what you are looking for. For more updates and tutorials just kindly visit this site. Enjoy Coding!
More Tutorials for Python Language
Add new comment
- 102 views