How to Find the Largest Prime Factor in Python
In this tutorial, we’ll learn how to program "How to Find the Largest Prime Factor in Python." We’ll focus on finding the largest prime factor of a given number. The objective is to carefully determine and display the largest prime factor. A sample program will be provided to demonstrate the coding process, making it simple and easy to understand. So, let’s get started!
This topic is easy to understand. Simply follow the instructions I provide, and you’ll be able to complete it effortlessly. The program I’ll demonstrate shows the correct way to calculate the largest prime factor. I’ll also provide a simple and efficient method to accomplish this effectively. So, let’s start coding!
Getting Started:
First you will have to download & install the Python IDLE's, here's the link for the Integrated Development And Learning Environment for Python https://www.python.org/downloads/.
Creating Main Function
This is the main function of the application. The following code will display a simple GUI in terminal console that will display program. To do this, simply copy and paste these blocks of code into the IDLE text editor.- import math
- def maxPrimeFactors (n):
- maxPrime = -1
- while n % 2 == 0:
- maxPrime = 2
- n >>= 1
- for i in range(3, int(math.sqrt(n)) + 1, 2):
- while n % i == 0:
- maxPrime = i
- n = n / i
- if n > 2:
- maxPrime = n
- return int(maxPrime)
- while True:
- print("\n================= Find the Largest Prime Factor =================\n\n")
- number = int(input("Enter a number: "))
- print("\nLargest Prime Factor of a number: ", maxPrimeFactors(number))
- opt = input("\nDo you want to try again?(yes/no): ")
- if opt.lower() == 'yes':
- ret=False
- elif opt.lower() == 'no':
- ret=True
- print("Exiting program....")
- else:
- print("Please enter yes/no:")
- break
- if ret == False:
- continue
This program calculates the largest prime factor of a given number by iteratively dividing the number by its smallest prime factors until only the largest prime factor remains. It begins by handling the factor of 2 and then checks all odd numbers up to the square root of the input, dividing the number whenever it finds a factor. If the remaining number is greater than 2 after these divisions, it is considered the largest prime factor. The program then displays the result and prompts the user to continue or exit, repeating the process based on user input.
Output:
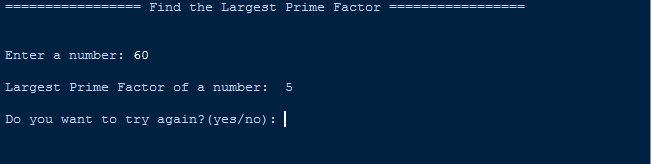
There you have it we successfully created How to Find the Largest Prime Factor in Python. I hope that this simple tutorial help you to what you are looking for. For more updates and tutorials just kindly visit this site. Enjoy Coding!
More Tutorials for Python Language
Add new comment
- 42 views