How to Check if the Number is a Fibonacci Number in Python
In this tutorial, we will program 'How to Check if a Number is a Fibonacci Number in Python.' We will learn how to determine if a given number is a Fibonacci number. The objective is to check whether the entered number is a Fibonacci number. I will provide a sample program to demonstrate the actual coding process in this tutorial.
This topic is very easy to understand. Just follow the instructions I provide, and you will be able to do it yourself with ease. The program I will show you covers the basics of programming to determine whether a number is a Fibonacci number. I will do my best to provide you with a simple method for finding Fibonacci numbers. So, let's start with the coding.
Getting Started:
First you will have to download & install the Python IDLE's, here's the link for the Integrated Development And Learning Environment for Python https://www.python.org/downloads/.
Creating Main Function
This is the main function of the application. The following code will display a simple GUI in terminal console that will display program. To do this, simply copy and paste these blocks of code into the IDLE text editor.- import math
- def isPerfectSquare(x):
- s = int(math.sqrt(x))
- return s*s == x
- def isFibonacci(n):
- return isPerfectSquare(5*n*n + 4) or isPerfectSquare(5*n*n - 4)
- ret = False
- while True:
- print("\n================== Check if the Number is a Fibonacci Number ==================\n\n")
- num=int(input("Enter a number: "))
- if(isFibonacci(num) == True):
- print(num, "is a Fibonacci Number")
- else:
- print(num, "is a not Fibonacci Number ")
- opt = input("\nDo you want to try again?(yes/no): ")
- if opt.lower() == 'yes':
- ret=False
- elif opt.lower() == 'no':
- ret=True
- print("Exiting program....")
- else:
- print("Please enter yes/no:")
- break
- if ret == False:
- continue
This script checks if a user-provided number is a Fibonacci number. It repeatedly prompts the user to input a number, checks if the number is a Fibonacci number using a mathematical property, and displays the result. After each check, the user is asked if they want to try again, allowing them to either continue or exit the program.
Output:
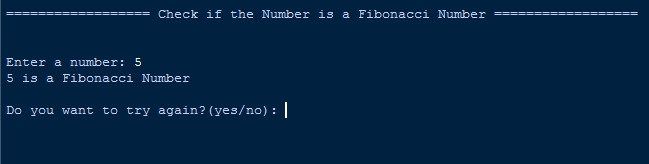
There you have it we successfully created How to Check if the Number is a Fibonacci Number in Python. I hope that this simple tutorial help you to what you are looking for. For more updates and tutorials just kindly visit this site. Enjoy Coding!
More Tutorials for Python Language
Add new comment
- 30 views