CRUD Operation using PHP/MySQLi with Bootstrap Tutorial
This tutorial will teach you the basic Create, Read, Update, and Delete Operations using PHP/MySQLi with Boostrap/Modal. Note: Bootstrap and Javascripts used in this tutorial are hosted so you need an internet connection for them to work.
Creating our Database
The first step is to create our database.
- Open phpMyAdmin.
- Click databases, create a database, and name it as "sample".
- After creating a database, click the SQL and paste the below code. See the image below for detailed instructions.
- CREATE TABLE `user` (
- `userid` INT(11) NOT NULL AUTO_INCREMENT,
- `firstname` VARCHAR(30) NOT NULL,
- `lastname` VARCHAR(30) NOT NULL,
- `address` VARCHAR(100) NOT NULL,
- PRIMARY KEY(`userid`)
- ) ENGINE=InnoDB DEFAULT CHARSET=latin1;
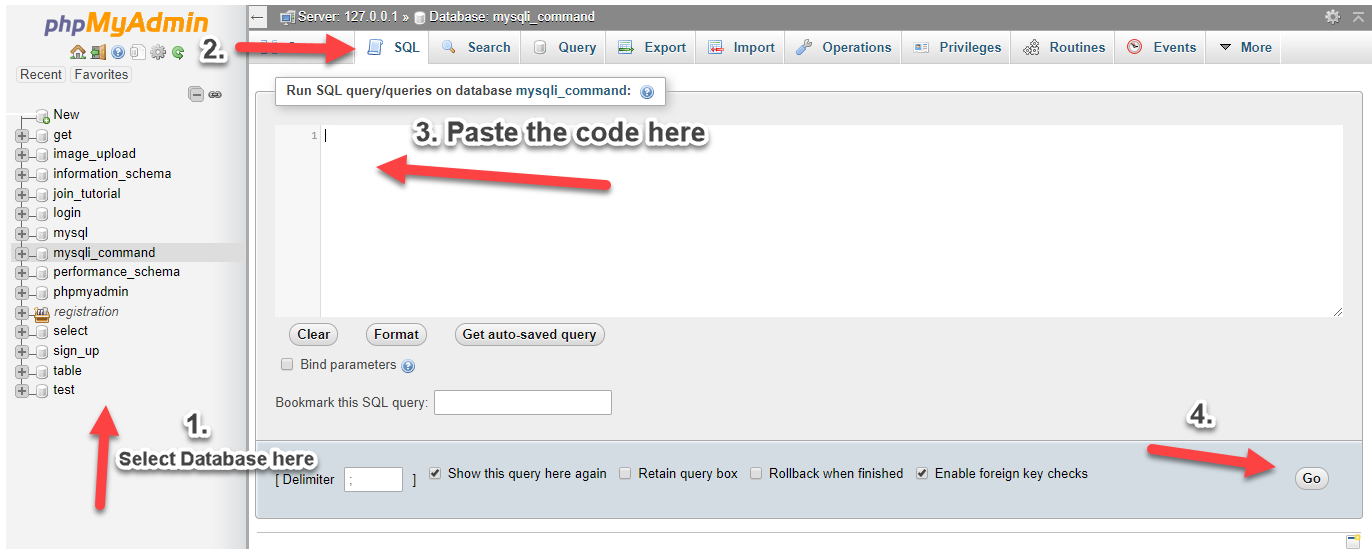
Creating our Connection
Next, we create our connection to our database. This will serve as the bridge between our forms and database. We name this as "conn.php".
- <?php
- //MySQLi Procedural
- if (!$conn) {
- }
- ?>
index.php
The next step is to create our sample table. This will be our Read.
- <!DOCTYPE html>
- <html>
- <head>
- <link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.6/css/bootstrap.min.css" />
- </head>
- <body>
- <div class="container">
- <div class="well" style="margin:auto; padding:auto; width:80%;">
- <table class="table table-striped table-bordered table-hover">
- <thead>
- </thead>
- <tbody>
- <?php
- include('conn.php');
- $query=mysqli_query($conn,"select * from `user`");
- while($row=mysqli_fetch_array($query)){
- ?>
- <tr>
- <td>
- <?php include('button.php'); ?>
- </td>
- </tr>
- <?php
- }
- ?>
- </tbody>
- </table>
- </div>
- <?php include('add_modal.php'); ?>
- </div>
- </body>
- </html>
add_modal.php
This is our form to add a new row to our database.
- <!-- Add New -->
- <div class="modal fade" id="addnew" tabindex="-1" role="dialog" aria-labelledby="myModalLabel" aria-hidden="true">
- <div class="modal-dialog">
- <div class="modal-content">
- <div class="modal-header">
- </div>
- <div class="modal-body">
- <div class="container-fluid">
- <form method="POST" action="addnew.php">
- <div class="row">
- <div class="col-lg-2">
- </div>
- <div class="col-lg-10">
- <input type="text" class="form-control" name="firstname">
- </div>
- </div>
- <div class="row">
- <div class="col-lg-2">
- </div>
- <div class="col-lg-10">
- <input type="text" class="form-control" name="lastname">
- </div>
- </div>
- <div class="row">
- <div class="col-lg-2">
- </div>
- <div class="col-lg-10">
- <input type="text" class="form-control" name="address">
- </div>
- </div>
- </div>
- </div>
- <div class="modal-footer">
- </form>
- </div>
- </div>
- </div>
- </div>
addnew.php
This is our code for adding the data in our add form to our database.
- <?php
- include('conn.php');
- $firstname=$_POST['firstname'];
- $lastname=$_POST['lastname'];
- $address=$_POST['address'];
- mysqli_query($conn,"insert into user (firstname, lastname, address) values ('$firstname', '$lastname', '$address')");
- ?>
button.php
This contains our Edit Modal and Delete Modal.
- <!-- Delete -->
- <div class="modal fade" id="del<?php echo $row['userid']; ?>" tabindex="-1" role="dialog" aria-labelledby="myModalLabel" aria-hidden="true">
- <div class="modal-dialog">
- <div class="modal-content">
- <div class="modal-header">
- </div>
- <div class="modal-body">
- <?php
- $del=mysqli_query($conn,"select * from user where userid='".$row['userid']."'");
- $drow=mysqli_fetch_array($del);
- ?>
- <div class="container-fluid">
- </div>
- </div>
- <div class="modal-footer">
- </div>
- </div>
- </div>
- </div>
- <!-- /.modal -->
- <!-- Edit -->
- <div class="modal fade" id="edit<?php echo $row['userid']; ?>" tabindex="-1" role="dialog" aria-labelledby="myModalLabel" aria-hidden="true">
- <div class="modal-dialog">
- <div class="modal-content">
- <div class="modal-header">
- </div>
- <div class="modal-body">
- <?php
- $edit=mysqli_query($conn,"select * from user where userid='".$row['userid']."'");
- $erow=mysqli_fetch_array($edit);
- ?>
- <div class="container-fluid">
- <form method="POST" action="edit.php?id=<?php echo $erow['userid']; ?>">
- <div class="row">
- <div class="col-lg-2">
- </div>
- <div class="col-lg-10">
- <input type="text" name="firstname" class="form-control" value="<?php echo $erow['firstname']; ?>">
- </div>
- </div>
- <div class="row">
- <div class="col-lg-2">
- </div>
- <div class="col-lg-10">
- <input type="text" name="lastname" class="form-control" value="<?php echo $erow['lastname']; ?>">
- </div>
- </div>
- <div class="row">
- <div class="col-lg-2">
- </div>
- <div class="col-lg-10">
- <input type="text" name="address" class="form-control" value="<?php echo $erow['address']; ?>">
- </div>
- </div>
- </div>
- </div>
- <div class="modal-footer">
- </div>
- </form>
- </div>
- </div>
- </div>
- <!-- /.modal -->
edit.php
The code for our Update Form.
- <?php
- include('conn.php');
- $id=$_GET['id'];
- $firstname=$_POST['firstname'];
- $lastname=$_POST['lastname'];
- $address=$_POST['address'];
- mysqli_query($conn,"update user set firstname='$firstname', lastname='$lastname', address='$address' where userid='$id'");
- ?>
delete.php
Lastly, this our delete code to delete row in our table.
- <?php
- include('conn.php');
- $id=$_GET['id'];
- ?>
DEMO
That ends this tutorial. For any questions or comments, feel free to comment below or message me here. Hope this tutorial helps. Happy Coding :)
Note: Due to the size or complexity of this submission, the author has submitted it as a .zip file to shorten your download time. After downloading it, you will need a program like Winzip to decompress it.
Virus note: All files are scanned once-a-day by SourceCodester.com for viruses, but new viruses come out every day, so no prevention program can catch 100% of them.
FOR YOUR OWN SAFETY, PLEASE:
1. Re-scan downloaded files using your personal virus checker before using it.
2. NEVER, EVER run compiled files (.exe's, .ocx's, .dll's etc.)--only run source code.
Comments
Add new comment
- Add new comment
- 26917 views