Python TypeError: bad operand type for unary +: 'str' [Solved]
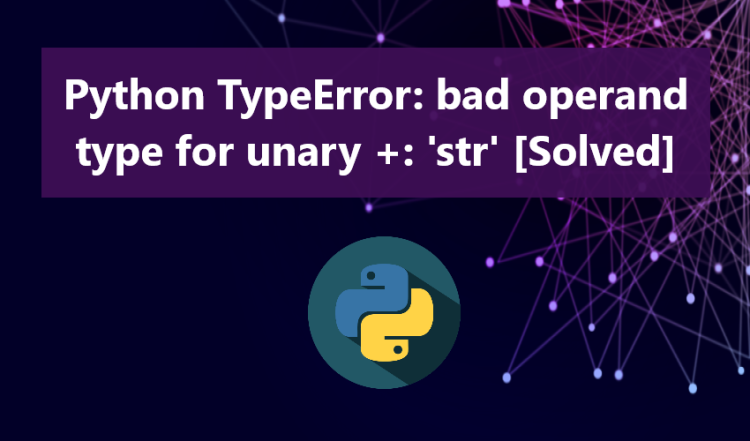
This article delves into an exploration of the cause and solution for a Python Type Error Message specifically thrown as TypeError: bad operand type for unary +: 'str'. If you're currently grappling with this error in your Python development, this article aims to provide insights into its nature and offers sample code snippets to replicate the error scenarios as well as solutions.
What is TypeError message in Python?
The TypeError is an exception or error that occurs when there is an inappropriate data type for an object or variable in an operation. This error can also arise when we try to use a specific method or object with incorrect argument types.
In Python, TypeError messages take various forms, some of which are represented as follows:
- TypeError: 'dict_values' object is not subscriptable
- TypeError: can't compare offset-naive and offset-aware datetimes
- Typeerror: can't multiply sequence by non-int of type 'numpy.float64'
What is unary operator in Python?
Python's Unary Operators are computational operators that yield a single result and operate on a single operand. Commonly used unary operators include (+) and (-), which have the capability to transform positive values or numbers into negative ones and vice versa. For instance, if we have a positive value of 23
and we apply the negative operator (-)
, it will convert the value into a negative one, resulting in -23
.
Why does TypeError: bad operand type for unary +: 'str' message in Python occurs?
The TypeError: bad operand type for unary +: 'str' error occurs when we try to apply unary operators to a string. As previously mentioned, these operators are computational and are intended to use with integer or float values.
Common mistakes that trigger this error include the following:
- Defining number values as strings: This error can occur when integer or float values are defined as string types.
- Concatenation: The error can also occur when strings are concatenated incorrectly.
Examples
Here are the following sample code snippets illustrating potential scenarios that can lead to the Python's bad operand type for unary str error message being raised:
Scenario #1
- # Sample String
- data = "SourceCodester"
- output = +data
- # print output
- print(output)
In the provided snippet above, we attempt to use the (+)
unary operator, resulting in an error due to the invalid type of value provided. This error can be easily rectified using the following approach:
Solution #1
If your intention is to use the defined value as a string, you can resolve the issue by simply removing the unary operator.
- # Sample String
- data = "SourceCodester"
- output = data
- # print output
- print(output)
Solution #2
If your intention is to execute the functionality of a unary operator, it's crucial to ensure that the defined value is indeed an integer or float value.
- # Sample String
- data = "2306"
- output = +int(data)
- # print output
- print(output)
Scenario #2
- # Sample String
- label = "Total Sales:"
- # Sample Amount
- total = "500.00"
- # print output
- print(label, + total)
In this scenario, the main purpose of utilizing the (+)
operator is to concatenate or combine two strings or values. The error arises from an incorrect approach to concatenating these two values.
To avoid the error in this scenario, you can use either the (,)
or the (+)
symbol exclusively for concatenation.
Solution 1
- # Sample String
- label = "Total Sales:"
- # Sample Amount
- total = "500.00"
- # print output
- print(label, total)
Solution 2
- # Sample String
- label = "Total Sales: "
- # Sample Amount
- total = "500.00"
- # print output
- print(label + total)
Moreover, the TypeError: bad operand type for unary +: 'str' error can be remedied by incorporating a conditional parameter to verify the type of the defined value. This practice is considered one of the best practices in Python coding. Refer to the following code snippet for a clearer illustration:
- # Sample String
- data = "-2306"
- if type(data) == str:
- data = int(data)
- output = -data
- print(output)
Conclusion
In simple terms, the Python error TypeError: bad operand type for unary +: 'str' occurs when an operation is attempted with an improperly defined value type. The solution to this error is to ensure that the defined value is either an integer or a floating-point number.
And there you have it! I trust that this article will assist you in resolving the issue you are currently facing. For further resources, feel free to explore this website for additional Free Source Code, Tutorials, and articles covering various programming topics.
Happy Coding =)
- 4109 views