How to Fix JavaScript Error Message "DOMException: Failed to execute 'appendChild' on 'Node'"?
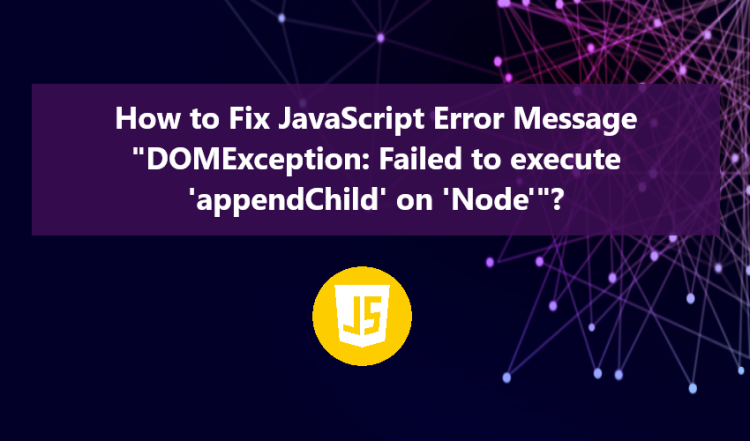
This article delves into an exploration of the JavaScript error message that reads "DOMException: Failed to execute 'appendChild' on 'Node'". I will provide snippets to illustrate when this error occurs and the corresponding code solutions to rectify it.
The DOM, which stands for the Data Object Model, in JavaScript serves as the data representation of objects that constitute the structure and content of a web document. This error can arise within the context of DOM Manipulation techniques, aimed at enhancing the user experience within specific web applications.
Why does the DOMException: Failed to execute 'appendChild' on 'Node' JS error occurs?
The JavaScript error thrown as DOMException: Failed to execute 'appendChild' on 'Node' occurs when there's an improper provision of a Node Element within the appendChild() function or method.
The appendChild() function is a built-in method in JavaScript that enables the addition of a child node to the end of a particular parent node's list.
Sample 1:
Here's a sample snippet that simulate the error to occur:
- //Sample Node
- const textNode = document.createTextNode(`Sample Text`)
- //Sample Node Element
- const el = document.createElement('div')
- //Attempting to append Element to the text Node
- textNode.appendChild(el)
The script given above portrays a piece of code involving DOM Manipulation, which endeavors to append or include a particular node within the child list of another node. Executing this script will result in an error being generated, primarily because an inappropriate Node Type has been designated as the parent node.
Solution 1:
The presented snippet can be rectified by ensuring the validity of the Parent Node Type. Refer to the following code snippet for clarity:
- //Sample Node
- const textNode = document.createTextNode(`Sample Text`)
- //Sample Node Element
- const el = document.createElement('div')
- //Attempting to append Text Node to the Element Node
- el.appendChild(textNode)
- document.querySelector('section').appendChild(el)
In the above snippet, it's evident that the el constant represents a valid element capable of accommodating child elements. The resulting output of the code will resemble the image provided below:
Solution 2
Another approach to prevent the occurrence of the error message is to incorporate an additional conditional script parameter before executing the appendChild method. In JavaScript, the node's type can be determined using .nodeType, and this value can be compared with the default node constant, Node.ELEMENT_NODE.
- //Sample Node
- const textNode = document.createTextNode(`Sample Text`)
- if(textNode.nodeType == Node.ELEMENT_NODE){
- //Sample Node Element
- const el = document.createElement('div')
- //Attempting to append Element to the text Node
- textNode.appendChild(el)
- }else{
- // do something if node is not valid
- console.warn(` textNode constant is not a valid Node Element! `)
- }
Sample 2
Below is an additional example snippet that triggers the "DOMException: Failed to execute 'appendChild' on 'Node'" error:
- //Sample Element to Append
- const ElementToAppend = `<p>Sample Text only</p>`;
- //Sample Node Element
- const el = document.createElement('div')
- //Attempting to append Element to the text Node
- el.appendChild(ElementToAppend)
The error is generated because of an incorrect type in the argument or parameter supplied to the appendChild function.
Solution 2.1
In the second example, the issue can be resolved by transforming the element string data into a Node Element. This can be achieved using the following code snippet:
- //Sample Element to Append
- const ElementToAppend = document.createElement('p');
- ElementToAppend.innerText = 'Sample Text only';
- //Sample Node Element
- const el = document.createElement('div')
- //Attempting to append Element to the text Node
- el.appendChild(ElementToAppend)
- document.querySelector('section').appendChild(el)
Conclusion
To put it simple, the DOMException: Failed to execute 'appendChild' on 'Node' error in JavaScript occurs when we try to attach a child Node to an invalid parent or vice versa. This issue can be resolved by ensuring that the provided node types are appropriate, where the parent node can accept a child node, and the child node is accurate. Additionally, a recommended practice to avert this error when dealing with DOM Manipulation is to include a conditional parameter that checks the node type.
And there you have it! I hope that this article assists you with your inquiries or challenges during your development phase. For further insights, feel free to explore this website for more Free Source Codes, Tutorials, and articles on various programming languages.
You might also want to read about the following:
- Add new comment
- 838 views