How to Upload and Read PDF File in VB.Net and MySQL Database
Submitted by janobe on Friday, September 7, 2018 - 17:29.
In this tutorial, I will teach you how to Upload and Read PDF File in VB.Net and MySQL Database. This Method will help you Upload the file in the database and view it into the Adobe PDF Reader.This is a step by step guide and it's very easy to learn.
Before we start:
Create a database named "test".
Create a table in the database that you have created.
Note: add
Lets begin:
Step 1
Open Microsoft Visual Studio 2015 and create a new windows form application. After that, do the following form just like shown below.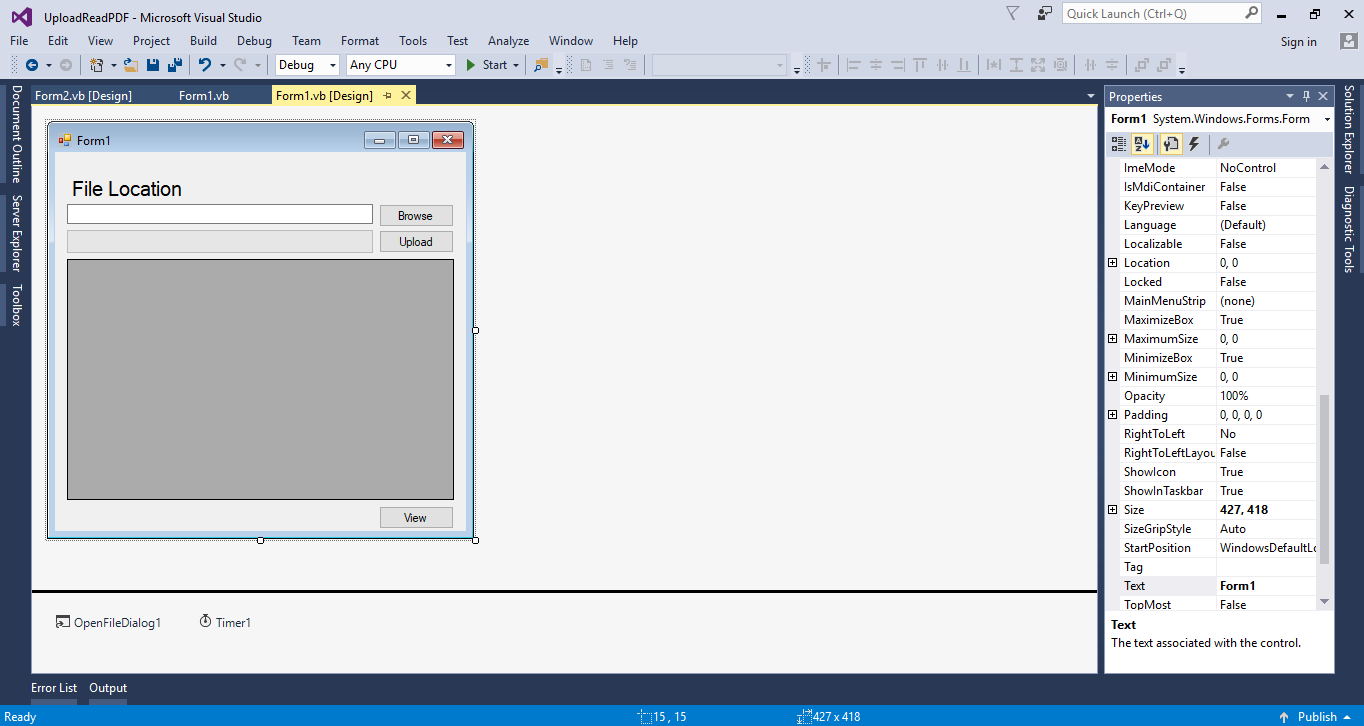
Step 2
Add another form and add PDF Reader inside the form.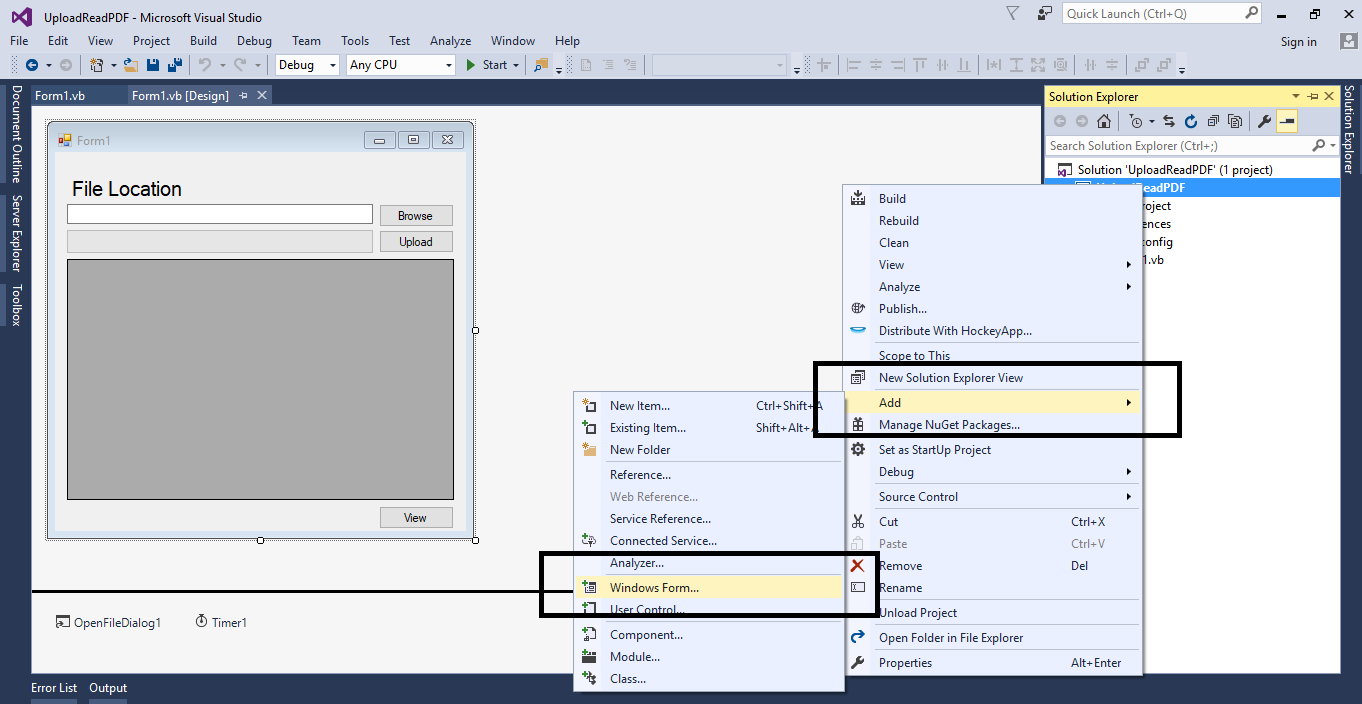
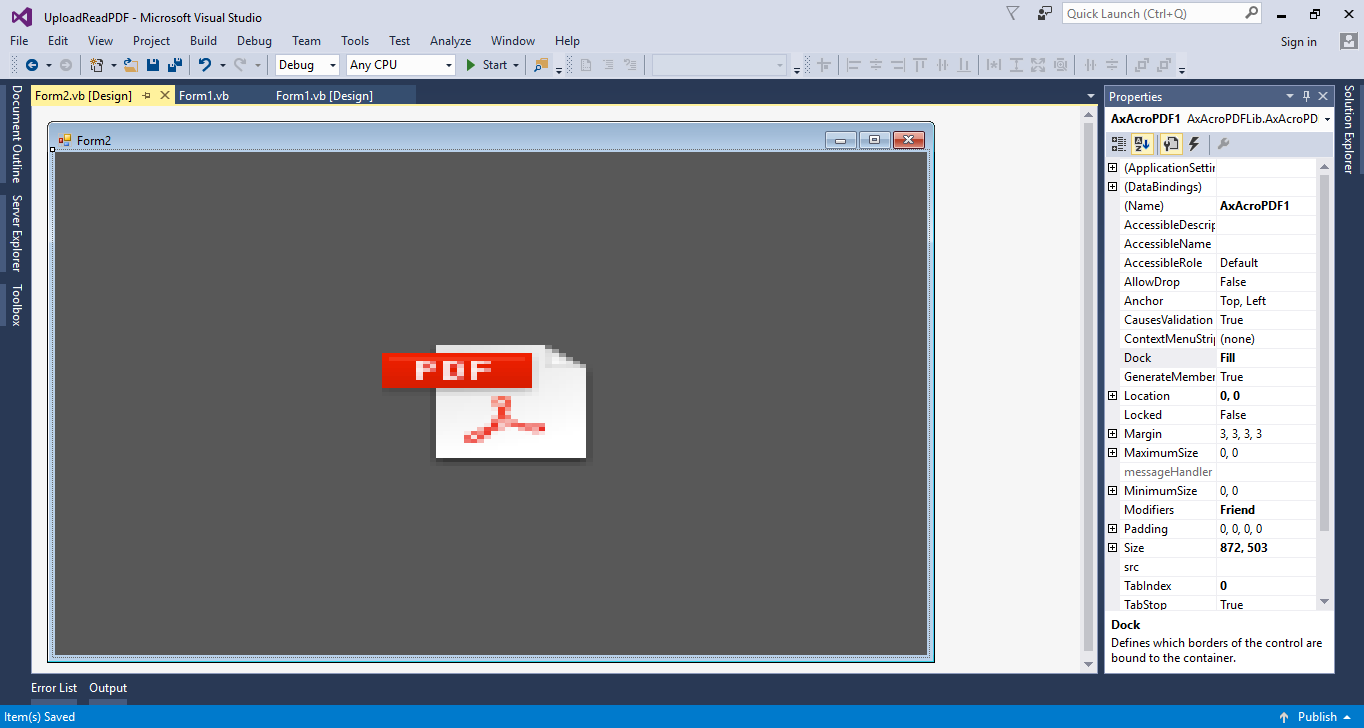
Step 3
In the Form1, double click the Browse button to fire theclick event handler
and do the following code for finding the PDF file.
- Try
- OpenFileDialog1.Filter = "PDF | *.pdf"
- If OpenFileDialog1.ShowDialog = DialogResult.OK Then
- TextBox1.Text = OpenFileDialog1.FileName
- End If
- Catch ex As Exception
- MsgBox(ex.Message)
- End Try
Step 4
Set the imports above the Public Class.- Imports MySql.Data.MySqlClient
Step 5
Initialize all the classes that are needed.- Dim con As MySqlConnection = New MySqlConnection("server=localhost;user id=root;password=janobe;database=test;sslMode=none")
- Dim cmd As MySqlCommand
- Dim da As MySqlDataAdapter
- Dim dt As DataTable
- Dim sql As String
Step 6
Do the following code for retrieving data from the database to the datagridview.- Private Sub Form1_Load(sender As Object, e As EventArgs) Handles MyBase.Load
- Try
- con.Open()
- sql = "SELECT * FROM tblfiles"
- cmd = New MySqlCommand
- With cmd
- .Connection = con
- .CommandText = sql
- End With
- da = New MySqlDataAdapter
- dt = New DataTable
- da.SelectCommand = cmd
- da.Fill(dt)
- DataGridView1.DataSource = dt
- Catch ex As Exception
- MsgBox(ex.Message)
- Finally
- da.Dispose()
- con.Close()
- End Try
- End Sub
Step 7
Go back to the design view (Form1), double click the timer to fire thetick event handler
of it and do the following code for uploading pdf file in the database.
- Try
- ProgressBar1.Value += 1
- If ProgressBar1.Value = 100 Then
- Timer1.Stop()
- con.Open()
- sql = "INSERT INTO `tblfiles` (`FileName`) VALUES ('" & System.IO.Path.GetFileName(TextBox1.Text) & "')"
- cmd = New MySqlCommand
- With cmd
- .Connection = con
- .CommandText = sql
- .ExecuteNonQuery()
- End With
- If TextBox1.Text <> "" Then
- System.IO.File.Copy(TextBox1.Text, Application.StartupPath & "\PDF_Files\" & System.IO.Path.GetFileName(TextBox1.Text), True)
- End If
- MsgBox("Scanned file uploaded successfully.")
- TextBox1.Clear()
- ProgressBar1.Value = 0
- End If
- Catch ex As Exception
- MsgBox(ex.Message)
- Finally
- con.Close()
- End Try
- 'call to retrieve file
- Call Form1_Load(sender, e)
Step 8
Go back to the design view (Form1) again, double click the Upload button to fireclick event handler
and set the timer to start.
- Timer1.Start()
Step 9
Double click the View button to fire theclick event handler
and do the following code to view the uploaded pdf file.
- Try
- con.Open()
- sql = "SELECT * FROM tblfiles WHERE FileId=" & DataGridView1.CurrentRow.Cells(0).Value
- cmd = New MySqlCommand
- With cmd
- .Connection = con
- .CommandText = sql
- End With
- da = New MySqlDataAdapter
- dt = New DataTable
- da.SelectCommand = cmd
- da.Fill(dt)
- With Form2
- .Show()
- .Focus()
- .AxAcroPDF1.src = Application.StartupPath & "\PDF_Files\" & dt.Rows(0).Item("FileName")
- End With
- Catch ex As Exception
- MsgBox(ex.Message)
- Finally
- da.Dispose()
- con.Close()
- End Try
mysql.data.dll
for the references to access MySQL Library
The complete sourcecode is included. You can download it and run it on your computer.
For more question about this article. You can contact me @
Email – [email protected]
Mobile No. – 09305235027 – TNT
FB Account – https://www.facebook.com/onnaj.soicalap
Add new comment
- 6917 views