In this tutorial we will create a
Add Watermark To Image Using GD Library using PHP. PHP is a server-side scripting language designed primarily for web development. Using PHP, you can let your user directly interact with the script and easily to learned its syntax. It is mostly used by a newly coders for its user friendly environment. So Let's do the coding.
Before we get started:
First you have to download & install XAMPP or any local server that run PHP scripts. Here's the link for XAMPP server
https://www.apachefriends.org/index.html.
And this is the link for the jquery that i used in this tutorial
https://jquery.com/.
Lastly, this is the link for the bootstrap that i used for the layout design
https://getbootstrap.com/.
Setting Up GD Library
First, we are going to enable GD Library in PHP.
1. Open localhost server folder XAMPP, etc and locate php.ini.
2. Open php.ini and enable gd library by removing the semicolon in the line.
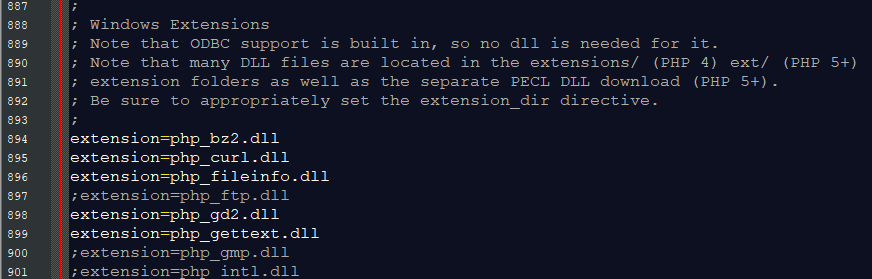
3. Save changes and Restart Server.
Creating The Interface
This is where we will create a simple form for our application. To create the forms simply copy and write it into your text editor, then save it as
index.php.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" name="viewport" content="width=device-width, initial-scale=1"/>
<link rel
="stylesheet" type
="text/css" href
="css/bootstrap.css"/>
</head>
<body>
<nav class="navbar navbar-default">
<div class="container-fluid">
<a class="navbar-brand" href="https://sourcecodester.com">Sourcecodester</a>
</div>
</nav>
<div class="col-md-3"></div>
<div class="col-md-6 well">
<h3 class="text-primary">PHP - Add Watermark To Image Using GD Library</h3>
<hr style="border-top:1px dotted #ccc;"/>
<div class="col-md-2"></div>
<div class="col-md-8">
<form method="POST" enctype="multipart/form-data" action="save.php">
<center>
<div id = "preview" style = "width:200px; height:200px; border:1px solid #000;">
<center id = "lbl">[Photo]</center>
</div>
</center>
<input type = "file" id = "file" name = "img" style="margin-left:22%;"/>
<br />
<div id="hide_form">
<label>Enter a Text</label>:
<input type="text" name="img_name" required="required"/>
<br />
<br />
<center><button class="btn btn-primary" name="save"><span class="glyphicon glyphicon-upload"></span> Save</button></center>
</div>
</form>
</div>
</div>
</body>
<script src="js/jquery-3.2.1.min.js"></script>
<script src="js/script.js"></script>
</html>
Creating PHP Script
This code contains the main function of the application. This code will get the image file then will insert some text after saving. To do that just copy and write this block of codes inside the text editor, then save it as
save.php.
<?php
if(ISSET($_POST['save'])){
if($_FILES['img']['name'] != ""){
$file = explode(".", $_FILES['img']['name']);
$text = $_POST['img_name'];
if($file[1] == "png"){
$file_name = $file[0].'.'.$file[1];
$tmp_file = $_FILES['img']['tmp_name'];
$location = "upload/".$file_name;
$font = 'Loveland Personal Use.ttf';
echo "<script>alert('Image Created!')</script>";
echo "<script>window.location = 'index.php'</script>";
}else{
echo "<script>alert('Only png files allowed!')</script>";
echo "<script>window.location = 'index.php'</script>";
}
}
}
?>
Creating jQuery Script
This is where the function that display the image.This code handles the image preview everytime the user upload some images. To do this just copy and write these block of codes inside the text editor, then save it as
script.js inside the js folder.
$(document).ready(function(){
$('#hide_form').hide();
var pic = $('<img id = "image" width = "100%" height = "100%"/>');
var lbl = $('<center id = "lbl">[Photo]</center>');
$("#file").change(function(){
$("#lbl").remove();
var files = !!this.files ? this.files : [];
if(!files.length || !window.FileReader){
$("#image").remove();
lbl.appendTo("#preview");
$('#hide_form').hide();
}
if(/^image/.test(files[0].type)){
var reader = new FileReader();
reader.readAsDataURL(files[0]);
reader.onloadend = function(){
pic.appendTo("#preview");
$("#image").attr("src", this.result);
$('#hide_form').show();
}
}
});
});
There you have it we successfully created
Add Watermark To Image Using GD Library using PHP. I hope that this simple tutorial help you to what you are looking for. For more updates and tutorials just kindly visit this site. Enjoy Coding!!!