PHP - OOP Login using Angular JS
Submitted by nurhodelta_17 on Friday, November 3, 2017 - 21:51.
Getting Started
Please take note that CSS and angular.js used in this tutorial are hosted so you need internet connection for them to work.Creating our Database
1. Open phpMyAdmin. 2. Click databases, create a database and name it as angular_db. 3. After creating a database, click the SQL and paste the below codes. See image below for detailed instruction.- CREATE TABLE `user` (
- `userid` INT(11) NOT NULL AUTO_INCREMENT,
- `username` VARCHAR(50) NOT NULL,
- `password` VARCHAR(50) NOT NULL,
- PRIMARY KEY(`userid`)
- ) ENGINE=InnoDB DEFAULT CHARSET=latin1;
- INSERT INTO `user` (`userid`, `username`, `password`) VALUES
- (1, 'neovic', 'devierte');
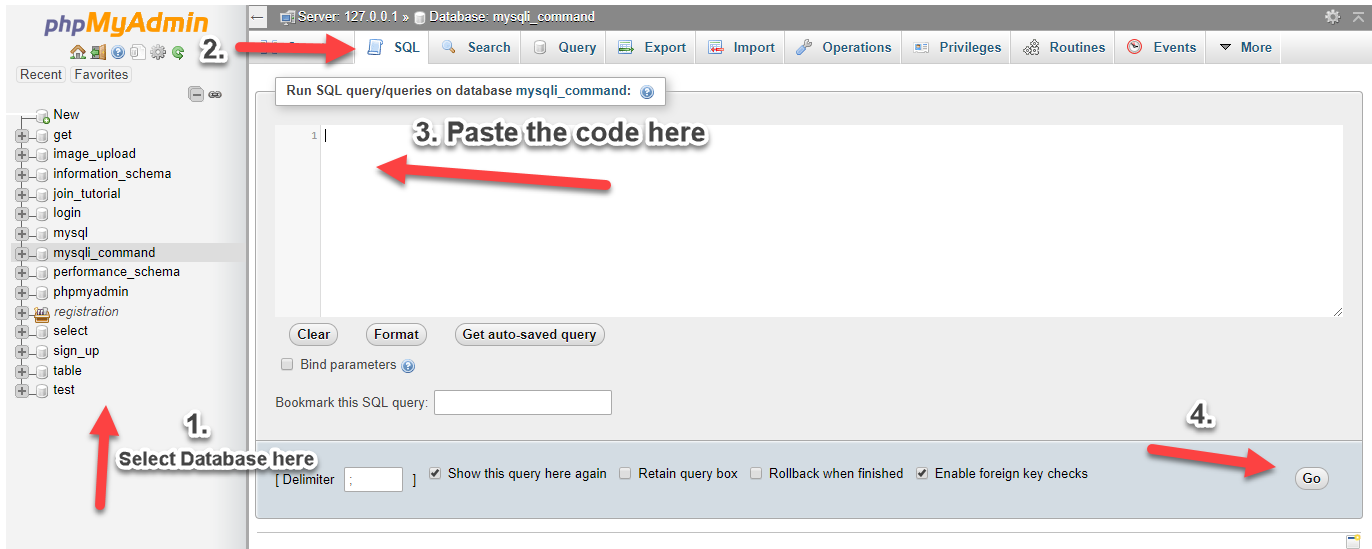
Creating our Connection
Next, we create our connection to our database. This will serve as the bridge between our forms and database. We name this as conn.php.- <?php
- $conn = new mysqli("localhost", "root", "", "angular_db");
- if ($conn->connect_error) {
- }
- ?>
index.php
This contains our login form.- <?php
- }
- ?>
- <!DOCTYPE html>
- <html ng-app="myapp" ng-controller="controller">
- <head>
- <title>PHP - OOP Login using Angular JS</title>
- <link href="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.7/css/bootstrap.min.css" rel="stylesheet">
- <script src="http://ajax.googleapis.com/ajax/libs/angularjs/1.4.8/angular.min.js"></script>
- </head>
- <body>
- <div class="container">
- <div class="row">
- <div class="col-md-6 col-md-offset-3">
- <center><h2>PHP - OOP Login using Angular JS</h2></center>
- </div>
- </div>
- <hr>
- <div class="row" id="loginform">
- <div class="col-md-4 col-md-offset-4">
- <div class="login-panel panel panel-primary">
- <div class="panel-heading">
- <h3 class="panel-title"><span class="glyphicon glyphicon-lock"></span> Sign In</h3>
- </div>
- <div class="panel-body">
- <form ng-submit="myFunct()">
- <fieldset>
- <div class="form-group">
- <input class="form-control" placeholder="Username" name="username" id="username" type="text" ng-model="username" autofocus>
- </div>
- <div class="form-group">
- <input class="form-control" placeholder="Password" name="password" id="password" type="password" ng-model="password">
- </div>
- <button type="submit" class="btn btn-lg btn-primary btn-block" ng-click="login()"><span class="glyphicon glyphicon-log-in"></span> {{btnName}}</button>
- </fieldset>
- </form>
- </div>
- </div>
- </div>
- </div>
- <div class="row">
- <div class="col-md-4 col-md-offset-4">
- <div class="alert alert-success"><center>{{alert}}</center></div>
- </div>
- </div>
- </div>
- <script src="myangular.js"></script>
- </body>
- </html>
myangular.js
This is our angular js code.- var app = angular.module("myapp", []);
- app.controller("controller", function($scope, $http) {
- $scope.btnName = "Login";
- $scope.alert = "Input User Credentials";
- $scope.login = function() {
- if ($scope.username == null) {
- alert("Please input Username");
- }
- else if ($scope.password == null) {
- alert("Please input Password");
- }
- else {
- $scope.btnName = "Logging in...";
- $scope.alert = "Checking Account. Please Wait...";
- $http.post(
- "login.php", {
- 'username': $scope.username,
- 'password': $scope.password,
- }
- ).success(function(data) {
- if(data!=''){
- setTimeout(function() {
- $scope.alert = "Login Failed. User not Found!";
- $scope.btnName = "Login";
- $scope.$apply();
- }, 3000);
- }
- else{
- setTimeout(function() {
- $scope.username = null;
- $scope.password = null;
- $scope.alert = "Login Successful. Welcome!";
- $scope.btnName = "Login";
- $scope.$apply();
- }, 3000);
- setTimeout(function() {
- window.location.reload();
- }, 4000);
- }
- });
- }
- }
- });
login.php
This is our PHP login code.- <?php
- include('conn.php');
- $query=$conn->query("select * from user where username='$username' and password='$password'");
- if($query->num_rows > 0){
- $row=$query->fetch_array();
- $_SESSION['user']=$row['userid'];
- }
- else{
- echo "1";
- }
- ?>
home.php
This is our goto page after a successful login.- <?php
- }
- include('conn.php');
- $query=$conn->query("select * from user where userid='".$_SESSION['user']."'");
- $row=$query->fetch_array();
- ?>
- <!DOCTYPE html>
- <html>
- <head>
- <title>PHP - OOP Login using Angular JS</title>
- <link href="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.7/css/bootstrap.min.css" rel="stylesheet">
- <script src="http://ajax.googleapis.com/ajax/libs/angularjs/1.4.8/angular.min.js"></script>
- </head>
- <body>
- <div class="container">
- <div class="row">
- <div class="col-md-6 col-md-offset-3">
- <center><h2>PHP - OOP Login using Angular JS</h2></center>
- </div>
- </div>
- <hr>
- <div class="row">
- <div class="col-md-4 col-md-offset-4">
- <h2><center>Welcome, <?php echo $row['username']; ?></center></h2>
- <center><a href="logout.php" class="btn btn-danger"><span class="glyphicon glyphicon-trash"></span> Logout</a></center>
- </div>
- </div>
- </div>
- </body>
- </html>
logout.php
Lastly, this is logout to destroy our user session.- <?php
- ?>
Add new comment
- 199 views