Android Progressbar Tutorial using Basic4Android
Submitted by donbermoy on Wednesday, January 29, 2014 - 09:31.
Hi! In this tutorial, I will introduce another component/controls in Basic4Android, the ProgressBar. ProgressBar displays a bar to the user representing how far the operation has progressed; the application can change the amount of progress modifying the length of the bar as it moves forward.
On this, you need to create one ProgressBar and named it as "pgb1", two Labels and named it as "lblPercent" for the percentage, and "Label1" and label it as "%". Next, named your abstract design as "tutorial". Your abstract designer will be like this one below:
Declare your variable in the Sub_Global like this:
For more inquiries and need programmer for your thesis systems in any kind of programming languages, just contact my number below.
Best Regards,
Engr. Lyndon R. Bermoy
IT Instructor/System Developer/Android Developer
STI College - Surigao City
Mobile: 09126450702
E-mail:[email protected]
Follow and add me in my Facebook Account: https://www.facebook.com/donzzsky
Visit and like my page on Facebook at: https://www.facebook.com/BermzISware
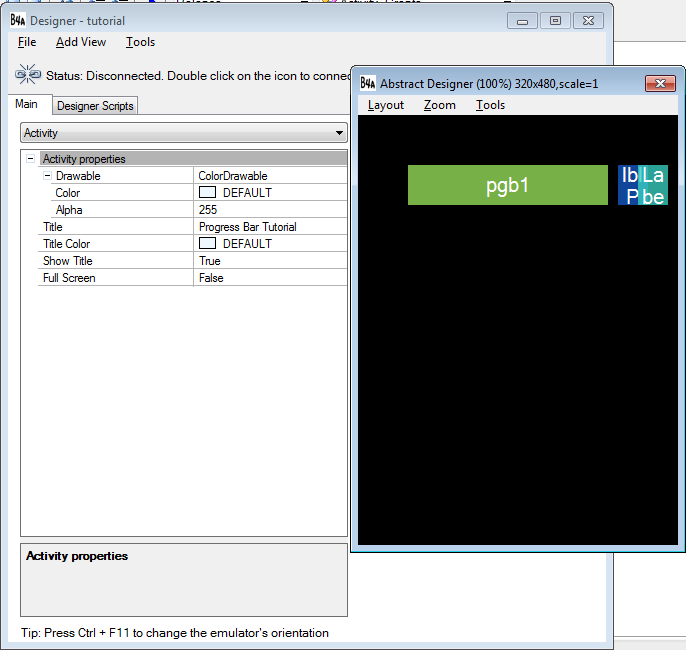
- Sub Globals
- 'These global variables will be redeclared each time the activity is created.
- 'These variables can only be accessed from this module.
- Dim timer1 As Timer
- Dim pgb1 As ProgressBar
- Dim num As Int
- Dim lblPercent As Label
- End Sub
Note:
timer1 - our variable used for the Timer. We need timer to determine the increasing progress of our ProgressBar. Timer schedules one-shot or recurring tasks for execution. pgb1 - our variable for our ProgressBar. num - we used num as an integer to increment the value of the ProgressBar that complements in time. lblPercent - this is our variable for our Label to display the number in percentage of the ProgressBar. Next, Declare the Timer and its method name Tick.- Sub timer1_Tick
- num = num + 1
- pgb1.Progress = num
- lblPercent.Text = num
- If num > 100 Then
- timer1.Enabled=False
- Msgbox("","Completed")
- pgb1.Visible=False
- End If
- End Sub
Note:
timer1_Tick
- timer1 here is our control and its method name is Tick in which it defines that the timer will now start.
num = num + 1
- num is declared to increment its value by 1.
pgb1.Progress = num
- the value of our progressbar will be equal to the incrementation of our num.
lblPercent.Text = num
- the value of our lblPercent as Label will be equal to the incrementation of our num in number.
- If num > 100 Then
- timer1.Enabled=False
- Msgbox("","Completed")
- pgb1.Visible=False
- End If
Note:
If our variable num will reach at 99, then our timer will be disabled and it will display "Completed". Also, our ProgressBar will be vanished. Now, We will create our Activity_Create:- Sub Activity_Create(FirstTime As Boolean)
- 'Do not forget to load the layout file created with the visual designer. For example:
- Activity.LoadLayout("tutorial")
- timer1.Initialize("timer1",400)
- timer1.Enabled=True
- End Sub
Note:
Activity.LoadLayout("tutorial")
- the named tutorial here is the design in which we created in the abstract designer.
timer1.Initialize("timer1",400)
- we initialized our timer as the named of timer1 and the 400 here is the time interval in milliseconds.
timer1.Enabled=True
- we initialized our timer to be enabled to perform its action.
Here's the full code of this tutorial:
- Sub Process_Globals
- 'These global variables will be declared once when the application starts.
- 'These variables can be accessed from all modules.
- End Sub
- Sub Globals
- 'These global variables will be redeclared each time the activity is created.
- 'These variables can only be accessed from this module.
- Dim timer1 As Timer
- Dim pgb1 As ProgressBar
- Dim num As Int
- Dim lblPercent As Label
- End Sub
- Sub Activity_Create(FirstTime As Boolean)
- 'Do not forget to load the layout file created with the visual designer. For example:
- Activity.LoadLayout("tutorial")
- timer1.Initialize("timer1",400)
- timer1.Enabled=True
- End Sub
- Sub timer1_Tick
- num = num + 1
- pgb1.Progress = num
- lblPercent.Text = num
- If num > 100 Then
- timer1.Enabled=False
- Msgbox("","Completed")
- pgb1.Visible=False
- End If
- End Sub
- Sub Activity_Resume
- End Sub
- Sub Activity_Pause (UserClosed As Boolean)
- End Sub
Add new comment
- 452 views