Pie/Doughnut Chart using ChartJS, AngularJS and PHP/MySQLi
Submitted by nurhodelta_17 on Wednesday, January 17, 2018 - 22:14.
Getting Started
I've used CDN for Bootstrap, Angular JS and Chart JS so you need internet connection for them to work.Creating our Database
First, we're gonna create our MySQL Database where we get our data. 1. Open phpMyAdmin. 2. Click databases, create a database and name it as angular. 3. After creating a database, click the SQL and paste the below codes. See image below for detailed instruction.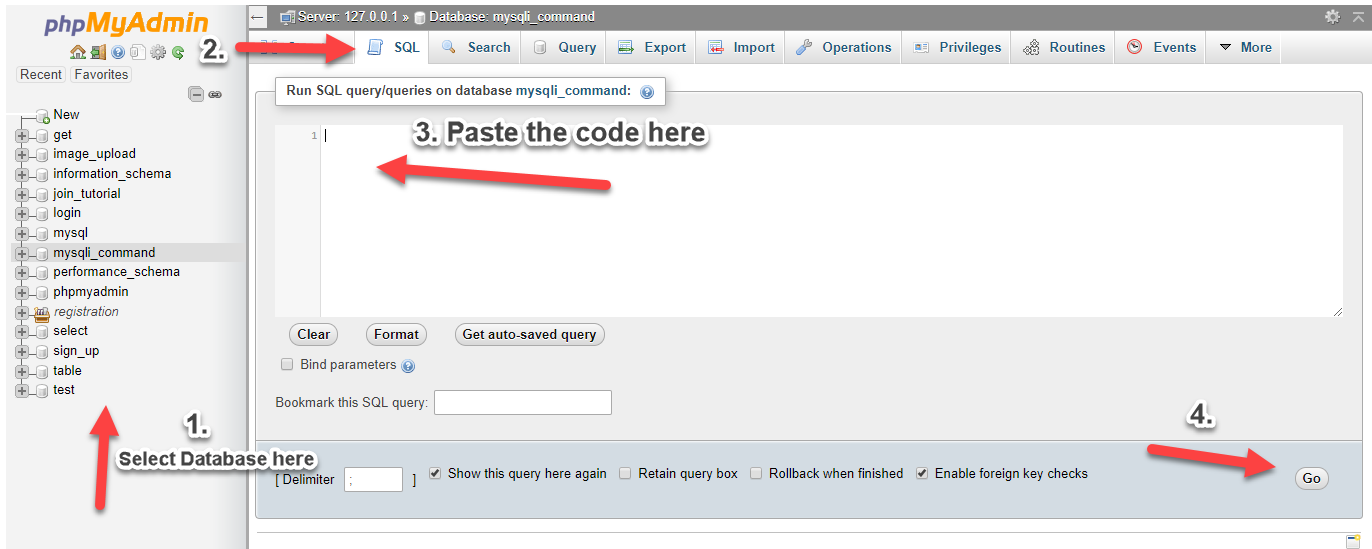
index.html
This is our index which contains our add form to update our chart and the chart itself.- <!DOCTYPE html>
- <html ng-app="app">
- <head>
- <meta charset="utf-8">
- <link href="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.7/css/bootstrap.min.css" rel="stylesheet">
- <style type="text/css">
- canvas{
- margin:auto;
- }
- .alert{
- margin-top:20px;
- }
- </style>
- </head>
- <body ng-controller="myCtrl">
- <div class="container">
- <div class="row">
- <div class="col-sm-3 col-md-offset-1" ng-init="fetchfruit()">
- <div class="form-group">
- <select ng-model="buy.fruitid" class="form-control">
- </select>
- </div>
- <div class="form-group">
- <input type="text" class="form-control" ng-model="buy.amount">
- </div>
- <div class="alert alert-success text-center" ng-show="success">
- {{ message }}
- </div>
- <div class="alert alert-danger text-center" ng-show="error">
- {{ message }}
- </div>
- </div>
- <div class="col-sm-7" ng-init="fetchsales()">
- <canvas id="dvCanvas" height="400" width="400"></canvas>
- </div>
- </div>
- </div>
- </body>
- </html>
app.js
This contains our angular js scripts.- var app = angular.module('app', []);
- app.controller('myCtrl', function ($scope, $http) {
- $scope.error = false;
- $scope.success = false;
- $scope.fetchfruit = function(){
- $http.get('fetchfruit.php').success(function(data){
- $scope.fruits = data;
- });
- }
- $scope.purchase = function(){
- $http.post('purchase.php', $scope.buy)
- .success(function(data){
- if(data.error){
- $scope.error = true;
- $scope.success = false;
- $scope.message = data.message;
- }
- else{
- $scope.success = true;
- $scope.error = false;
- $scope.message = data.message;
- $scope.fetchsales();
- $scope.buy = '';
- }
- });
- }
- //this fetches the data for our table
- $scope.fetchsales = function(){
- $http.get('fetchsales.php').success(function(data){
- var ctx = document.getElementById("dvCanvas").getContext('2d');
- var myChart = new Chart(ctx, {
- type: 'pie', // change the value of pie to doughtnut for doughnut chart
- data: {
- datasets: [{
- data: data.total,
- backgroundColor: ['blue', 'green', 'red', 'yellow']
- }],
- labels: data.fruitname
- },
- options: {
- responsive: false
- }
- });
- });
- }
- $scope.clear = function(){
- $scope.error = false;
- $scope.success = false;
- }
- });
fetchfruit.php
This is our PHP api that fetches data for our add form.- <?php
- $conn = new mysqli("localhost", "root", "", "angular");
- $sql = "SELECT * FROM fruits";
- $query = $conn->query($sql);
- while($row=$query->fetch_array()){
- $out[] = $row;
- }
- ?>
purchase.php
This is our PHP api/code that adds data into our database.- <?php
- $conn = new mysqli("localhost", "root", "", "angular");
- $fruitid = $data->fruitid;
- $amount = $data->amount;
- $sql = "INSERT INTO sales (fruitid, amount) VALUES ('$fruitid', '$amount')";
- $query = $conn->query($sql);
- if($query){
- $out['message'] = "Purchase added successfully";
- }
- else{
- $out['error'] = true;
- $out['message'] = "Cannot add purchase";
- }
- ?>
fetchsales.php
Lastly, this is our PHP api that fetches data for our chart.- <?php
- $conn = new mysqli("localhost", "root", "", "angular");
- $sql = "SELECT *, sum(amount) AS total FROM sales LEFT JOIN fruits ON fruits.fruitid=sales.fruitid GROUP BY sales.fruitid";
- $query = $conn->query($sql);
- while($row=$query->fetch_array()){
- $out['total'][] = $row['total'];
- $out['fruitname'][] = $row['fruitname'];
- }
- ?>
Add new comment
- 493 views