Angular JS Load more data using PHP/MySQLi
Submitted by nurhodelta_17 on Thursday, January 11, 2018 - 12:54.
Getting Started
I've used CDN for Bootstrap in this tutorial so you need internet connection for it to work.Creating our Database
First, we're going to create our database and insert sample data to use in our load more.- (1, 'Apple'),
- (2, 'Orange'),
- (3, 'Strawberry'),
- (4, 'Pineapple'),
- (5, 'Star Apple'),
- (7, 'Banana'),
- (8, 'Lemon'),
- (9, 'Mango'),
- (10, 'Guava'),
- (11, 'Watermelon'),
- (12, 'Avocado'),
- (13, 'Apricot'),
- (14, 'Blackberry'),
- (15, 'Coconut'),
- (16, 'Melon'),
- (17, 'Papaya'),
- (18, 'Peach'),
- (19, 'Pomelo'),
- (20, 'Grapes');
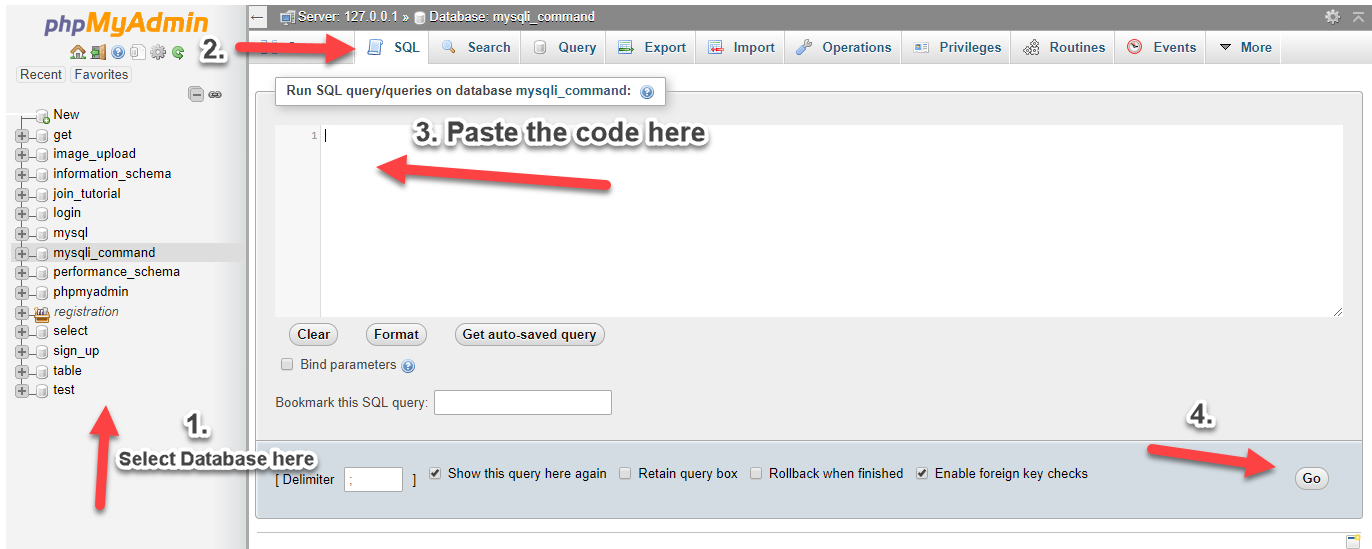
index.html
This is our index where we display our data.- <!DOCTYPE html>
- <html lang="en" ng-app="app">
- <head>
- <meta charset="utf-8">
- <link href="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.7/css/bootstrap.min.css" rel="stylesheet">
- </head>
- <body ng-controller="myController" ng-init="fetch()">
- <div class="container">
- <div class="col-md-4 col-md-offset-4">
- <div class="panel panel-default" ng-repeat="fruit in fruits">
- <div class="panel-body text-center">
- </div>
- </div>
- </div>
- </div>
- </body>
- </html>
angular.js
This contains our angular js scripts.- var app = angular.module('app', []);
- app.controller('myController', function($scope, $http){
- $scope.loadName = 'Load More';
- $scope.fetch = function(){
- $http.get('fetch.php')
- .success(function(data){
- $scope.fruits = data;
- console.log($scope.fruits);
- });
- }
- $scope.loadmore = function(){
- var lastid = $scope.fruits.length - 1;
- var lastobj = $scope.fruits[lastid];
- $http.post('loadmore.php', lastobj)
- .success(function(data){
- if(data == ''){
- $scope.loadName = 'No more Data to Load';
- }
- else{
- angular.forEach(data, function(newfruit){
- $scope.fruits.push(newfruit);
- });
- }
- console.log($scope.fruits);
- });
- }
- });
fetch.php
This is our PHP api/code in fetching our initial data.- <?php
- $conn = new mysqli('localhost', 'root', '', 'angular');
- $sql = "SELECT * FROM fruits ORDER BY fruitid ASC LIMIT 4";
- $query=$conn->query($sql);
- while($row=$query->fetch_array()){
- $output[] = $row;
- }
- ?>
loadmore.php
Lastly, this is our PHP code/api to fetch our addition data.- <?php
- $conn = new mysqli('localhost', 'root', '', 'angular');
- $id = $data->fruitid;
- $sql = "SELECT * FROM fruits WHERE fruitid > '$id' ORDER BY fruitid ASC LIMIT 4";
- $query=$conn->query($sql);
- while($row=$query->fetch_array()){
- $output[] = $row;
- }
- ?>
Add new comment
- 95 views