JFileChooser Component in Java
Submitted by donbermoy on Monday, November 17, 2014 - 11:49.
This is a tutorial in which we will going to create a program that has the JFileChooser Component using Java. The JFileChooser is used to let the user to choose or open a specific file.
So, now let's start this tutorial!
1. Open JCreator or NetBeans and make a java program with a file name of jFileChooserComponent.java.
2. Import the following packages:
3. Initialize your variable in your Main, variable fileChooser for the JFileChooser class.
4. Instantiate JFileChooser class variable with its FileFilter method to filter files. We will use the accept method to accept any files that has a .gif, .png, and .jpg file extension. Then we will use the getDescription method to have the description of these file extension of images.
Now, create again the FileFilter method to filter the text files with a .txt, .docx, and .rtf file extension.
5. To open the JFileChooser component in the JFrame, initialize a variable named var with a showOpenDialog instantiating a JFrame.
If our variable var will be equal to the JFileChooser's APPROVE_OPTION, then we will get the file name of the selected file using the getSelectedFile().getName() method and will display the filename.
Output:
Here's the full code of this tutorial:
For more inquiries and need programmer for your thesis systems in any kind of programming languages, just contact my number below.
Best Regards,
Engr. Lyndon Bermoy
IT Instructor/System Developer/Android Developer/Freelance Programmer
Mobile: 09488225971
Landline: 826-9296
E-mail:[email protected]
Add and Follow me on Facebook: https://www.facebook.com/donzzsky
Visit and like my page on Facebook at: https://www.facebook.com/BermzISware
- import java.io.*; //used for accessing the file class
- import javax.swing.*// used to access the JFrame and JFileChooser class
- return f.getName().toLowerCase().endsWith(".gif")|| f.getName().toLowerCase().endsWith(".png") || f.getName().toLowerCase().endsWith(".jpg") || f.isDirectory();
- }
- return "Image Files";
- }
- });
- return f.getName().toLowerCase().endsWith(".txt")|| f.getName().toLowerCase().endsWith(".docx") || f.getName().toLowerCase().endsWith(".rtf") || f.isDirectory();
- }
- return "Text Files";
- }
- });
- }
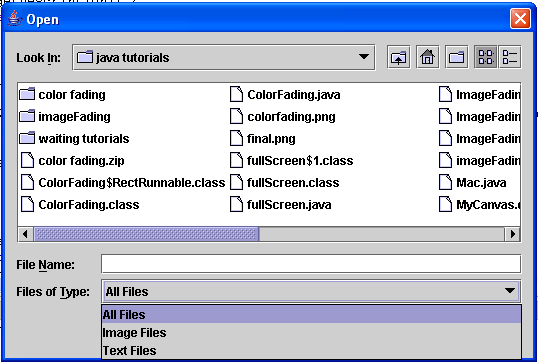
- import java.io.*; //used for accessing the file class
- import javax.swing.*;// used to access the JFrame and JFileChooser class
- public class jFileChooserComponent {
- return f.getName().toLowerCase().endsWith(".gif")|| f.getName().toLowerCase().endsWith(".png") || f.getName().toLowerCase().endsWith(".jpg") || f.isDirectory();
- }
- return "Image Files";
- }
- });
- return f.getName().toLowerCase().endsWith(".txt")|| f.getName().toLowerCase().endsWith(".docx") || f.getName().toLowerCase().endsWith(".rtf") || f.isDirectory();
- }
- return "Text Files";
- }
- });
- }
- }
- }
Add new comment
- 72 views