ProgressBar Component in Java
Submitted by donbermoy on Sunday, June 8, 2014 - 22:02.
Today in Java, i will teach you how to create a progressbar component using JProgressBar control in Java.
So, now let's start this tutorial!
1. Open JCreator or NetBeans and make a java program with a file name of ProgressBar.java.
2. Import javax.swing.* package because we will going to have the JProgressBar, JFrame, SwingUtilities in swing and also the JPanel as the container of this.
3. Initialize a variable for JProgressBa and named it as pbar. This will be below in your public class ProgressBar extends JPanel.
4. Create a constructor that is the same with the filename that will instantiate the JProgressBar, sets the minimum and maximum value of the progressbar, and will add this component in the JPanel.
5. Create also a method named updateBar and has variable newValue as Integer because this will be used to update the progress of the bar using the loop.
6. In your Main, instantiate the ProgressBar constructor that has the new keyword and make it final.
Set the title.
Set the windows characteristics.
Create a For Loop statement that the minimum value is equal to 0 and maximum value is 100 that has an increment capability with an i variable as integer. Initialize percent variable as integer that will be equal to i with the final keyword.
Now, create a try and catch method. In the try method, update the progressbar with it variable name and its value of for loop in percent variable using run method of SwingUtilities. And have a delay value of 100 millisecond. Then in the catch method, preferred to use the InterruptedException.
Press F5 to run the program.
Output:
Full source code:
Best Regards,
Engr. Lyndon Bermoy
IT Instructor/System Developer/Android Developer/Freelance Programmer
If you have some queries, feel free to contact the number or e-mail below.
Mobile: 09488225971
Landline: 826-9296
E-mail:[email protected]
Add and Follow me on Facebook: https://www.facebook.com/donzzsky
Visit and like my page on Facebook at: https://www.facebook.com/BermzISware
- import javax.swing.*;
- JProgressBar pbar;
- public ProgressBar() {
- pbar.setMinimum(0);
- pbar.setMaximum(100);
- add(pbar);
- }
- public void updateBar(int newValue) {
- pbar.setValue(newValue);
- }
- final ProgressBar it = new ProgressBar();
- frame.setContentPane(it);
- frame.pack();
- frame.setVisible(true);
- frame.setLocation(300,250);
- frame.setSize(200,150);
- for (int i = 0; i <= 100; i++) {
- final int percent = i;
- try {
- public void run() {
- it.updateBar(percent);
- }
- });
- ;
- }
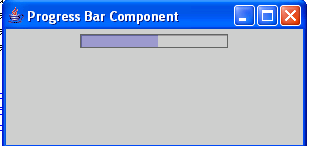
- import javax.swing.*;
- JProgressBar pbar;
- public ProgressBar() {
- pbar.setMinimum(0);
- pbar.setMaximum(100);
- add(pbar);
- }
- public void updateBar(int newValue) {
- pbar.setValue(newValue);
- }
- final ProgressBar it = new ProgressBar();
- frame.setContentPane(it);
- frame.pack();
- frame.setVisible(true);
- frame.setLocation(300,250);
- frame.setSize(200,150);
- for (int i = 0; i <= 100; i++) {
- final int percent = i;
- try {
- public void run() {
- it.updateBar(percent);
- }
- });
- ;
- }
- }
- }
- }
Comments
Add new comment
- Add new comment
- 259 views