Creating User Account Information in Java - Adding Records to Database
Submitted by donbermoy on Thursday, May 1, 2014 - 11:17.
Good day! This tutorial will focus on creating a user account information in java particularly in adding records to the database.
Now let's start this tutorial! :)
1. Create your database in ms access and named it sample.mdb with Login table and the following entities below.
2. Create your java program now named UserSettings.java.
3. Import the following libraries.
4. Initialize the following variables. 3 textfield for username, first name and family name, and 1 passwordfield for the password, 1 button named btnNew, and sql connection variables Connection, Statement, and PreparedStatement.
5. Create a constructor named UserSettings() same with your classname for creating the panels to put controls in the form as well as the connection in the database.
7. Create your ActionEvent for clicking the button. This will trigger to add records when clicking our btnNew button.
The sql syntax here "ps=cn.prepareStatement("INSERT INTO Login" + " (username,password,name1,name2) " + " VALUES(?,?,?,?)");" inserts the record in the database.
8. Create a method named clear to clear all your textfield.
9. Lastly create your Main. This will create the size and location of your form.
Output:
Here's the full code of this tutorial:
For more inquiries and need programmer for your thesis systems in any kind of programming languages, just contact my number below.
Best Regards,
Engr. Lyndon Bermoy
IT Instructor/System Developer/Android Developer/Freelance Programmer
Mobile: 09488225971
Landline: 826-9296
E-mail:[email protected]
Add and Follow me on Facebook: https://www.facebook.com/donzzsky
Visit and like my page on Facebook at: https://www.facebook.com/BermzISware


- import javax.swing.*;
- import java.awt.*;
- import java.awt.event.*;
- import java.sql.*;
- import java.lang.*;
- Connection cn;
- Statement st;
- public UserSettings() {
- super("User Account Settings");
- pane.setLayout(null);
- lblUser.setBounds(5,50,80,25);
- pane.add(lblUser);
- txtUser.setBounds(90,50,150,25);
- pane.add(txtUser);
- lblPass.setBounds(5,85,80,25);
- pane.add(lblPass);
- txtPass.setBounds(90,85,150,25);
- txtPass.setEchoChar('*');
- pane.add(txtPass);
- lblName1.setBounds(5,120,80,25);
- pane.add(lblName1);
- txtName1.setBounds(90,120,150,25);
- pane.add(txtName1);
- lblName2.setBounds(5,155,80,25);
- pane.add(lblName2);
- txtName2.setBounds(90,155,150,25);
- pane.add(txtName2);
- btnNew.setBounds(5,190,75,35);
- pane.add(btnNew);
- btnNew.addActionListener(this);
- setContentPane(pane);
- try{
- Class.forName("sun.jdbc.odbc.JdbcOdbcDriver");
- e.printStackTrace();
- }
- e.printStackTrace();
- }
- }
- if(source == btnNew){
- try{
- if (!uname.equals("") && !pass.equals("")&& !name1.equals("") && !name2.equals("")) {
- st= cn.createStatement();
- ps=cn.prepareStatement("INSERT INTO Login" + " (username,password,name1,name2) " + " VALUES(?,?,?,?)");
- ps.setString(1,txtUser.getText());
- ps.setString(2,txtPass.getText());
- ps.setString(3,txtName1.getText());
- ps.setString(4,txtName2.getText());
- ps.executeUpdate();
- JOptionPane.showMessageDialog(null,"New account has been successfully added.","Payroll System: User settings",JOptionPane.INFORMATION_MESSAGE);
- txtUser.requestFocus(true);
- st.close();
- clear();
- }
- else{
- JOptionPane.showMessageDialog(null,"Please Fill Up The Empty Fields","Warning",JOptionPane.WARNING_MESSAGE);
- }
- sqlEx.printStackTrace();
- JOptionPane.showMessageDialog(null,"Unable to save!.","Payroll System: User settings",JOptionPane.ERROR_MESSAGE);}
- }
- }
- public void clear(){
- txtUser.setText("");
- txtPass.setText("");
- txtName1.setText("");
- txtName2.setText("");
- }
- UserSettings panel = new UserSettings();
- panel.setSize(370,350);
- panel.setVisible(true);
- panel.setLocation(350,200);
- panel.setResizable(false);
- }
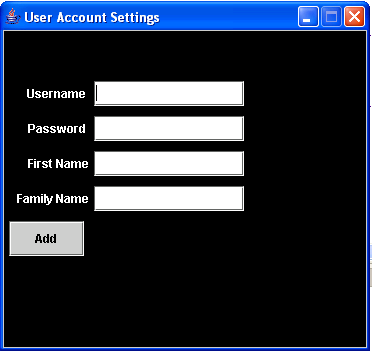
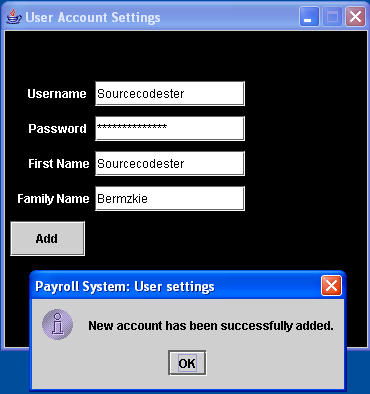
- import javax.swing.*;
- import java.awt.*;
- import java.awt.event.*;
- import java.sql.*;
- import java.lang.*;
- Connection cn;
- Statement st;
- public void clear(){
- txtUser.setText("");
- txtPass.setText("");
- txtName1.setText("");
- txtName2.setText("");
- }
- public UserSettings() {
- super("User Account Settings");
- pane.setLayout(null);
- lblUser.setBounds(5,50,80,25);
- pane.add(lblUser);
- txtUser.setBounds(90,50,150,25);
- pane.add(txtUser);
- lblPass.setBounds(5,85,80,25);
- pane.add(lblPass);
- txtPass.setBounds(90,85,150,25);
- txtPass.setEchoChar('*');
- pane.add(txtPass);
- lblName1.setBounds(5,120,80,25);
- pane.add(lblName1);
- txtName1.setBounds(90,120,150,25);
- pane.add(txtName1);
- lblName2.setBounds(5,155,80,25);
- pane.add(lblName2);
- txtName2.setBounds(90,155,150,25);
- pane.add(txtName2);
- btnNew.setBounds(5,190,75,35);
- pane.add(btnNew);
- btnNew.addActionListener(this);
- setContentPane(pane);
- try{
- Class.forName("sun.jdbc.odbc.JdbcOdbcDriver");
- e.printStackTrace();
- }
- e.printStackTrace();
- }
- }
- if(source == btnNew){
- try{
- if (!uname.equals("") && !pass.equals("")&& !name1.equals("") && !name2.equals("")) {
- st= cn.createStatement();
- ps=cn.prepareStatement("INSERT INTO Login" + " (username,password,name1,name2) " + " VALUES(?,?,?,?)");
- ps.setString(1,txtUser.getText());
- ps.setString(2,txtPass.getText());
- ps.setString(3,txtName1.getText());
- ps.setString(4,txtName2.getText());
- ps.executeUpdate();
- JOptionPane.showMessageDialog(null,"New account has been successfully added.","Payroll System: User settings",JOptionPane.INFORMATION_MESSAGE);
- txtUser.requestFocus(true);
- st.close();
- clear();
- }
- else{
- JOptionPane.showMessageDialog(null,"Please Fill Up The Empty Fields","Warning",JOptionPane.WARNING_MESSAGE);
- }
- sqlEx.printStackTrace();
- JOptionPane.showMessageDialog(null,"Unable to save!.","Payroll System: User settings",JOptionPane.ERROR_MESSAGE);}
- }
- }
- // public void frameUser(){
- UserSettings panel = new UserSettings();
- panel.setSize(370,350);
- panel.setVisible(true);
- panel.setLocation(350,200);
- panel.setResizable(false);
- }
- }