Number Validation: Only numbers in TextField using Java GUI
Submitted by donbermoy on Tuesday, April 29, 2014 - 15:18.
Some of my students before always asked how to filter a textbox with only numbers that can be inputted. Right away, they always made a mistake on writing the code because they are just using the NumberFormatException code. But actually it isn't wrong, it is just sometimes not applicable and not functioning at times when I can still remember my college days.
But now, I wrote this code to filter numbers on textbox or any controls in Java. I want to share and teach about this code.
Now let's begin this tutorial!
1. Create a program with a file name of numberValidation.java.
2. Import the following libraries.
You seemed to figure out that we always used asterisk (*). This asterisk means getting all the classes inside the libraries of awt, io, swing, and util. awt means Abstract Windowing Toolkit. This is a set of application program interfaces ( API) to create graphical user interface (GUI) objects, such as buttons, textfields, or any controls. awt.event gets the action performed or when clicking a button or any events of the controls.
3. Create a numberValidation that will extend JFrame because we will put a frame here.
The name txtNum is our variable in textfield with only 20 characters to be inputted.
4. Create a class named j2 that extends panel. We will make use of the Panel to put our textfield there.
5. Create a method name Numvalidator that has the parameter of JTextField with txtField variable. This will filter all those characters that are not numbers in the textfield. And will prompt "Only numbers are allowed!".
6. Create a constructor named numberValidation() that is the same with the filename.
7. Lastly, create your Main form. This will the contents of your UI with the size, visibility, and location to be displayed.
Here's the full code of this tutorial..
Best Regards,
Engr. Lyndon R. Bermoy
IT Instructor/System Developer/Android Developer/Freelance Programmer
If you have some queries, feel free to contact the number or e-mail below.
Mobile: 09102918563
Landline: 826-9296
E-mail:[email protected]
Visit and like my page on Facebook at: https://www.facebook.com/BermzISware
For more inquiries and need programmer for your thesis systems in any kind of programming languages, just contact my number below.
Best Regards,
Engr. Lyndon Bermoy
IT Instructor/System Developer/Android Developer/Freelance Programmer
Mobile: 09488225971
Landline: 826-9296
E-mail:[email protected]
Add and Follow me on Facebook: https://www.facebook.com/donzzsky
Visit and like my page on Facebook at: https://www.facebook.com/BermzISware
- import java.awt.*;
- import java.io.*;
- import javax.swing.*;
- import java.util.*;
- import java.awt.event.*;
- {
- public j2()
- {
- }
- {
- super.paint(g);
- g.drawString("Number Validation",60,70);
- }
- }
- {
- char c = e.getKeyChar();
- e.consume();
- JOptionPane.showMessageDialog(null, "Only numbers are allowed!","WARNING!!",JOptionPane.WARNING_MESSAGE);
- }
- }
- });
- }
- public numberValidation()
- {
- super("Number Validation ");
- panel1.add(txtNum);
- panel1.setOpaque(true);
- getContentPane().add(new j2(),"NORTH");
- getContentPane().add(panel1,"CENTER");
- Numvalidator(txtNum);
- setDefaultCloseOperation(DISPOSE_ON_CLOSE);
- }
- numberValidation p1= new numberValidation();
- p1.setSize(650,630);
- p1.setLocation(300,50);
- p1.setVisible(true);
- p1.setResizable(false);
- }
Output:
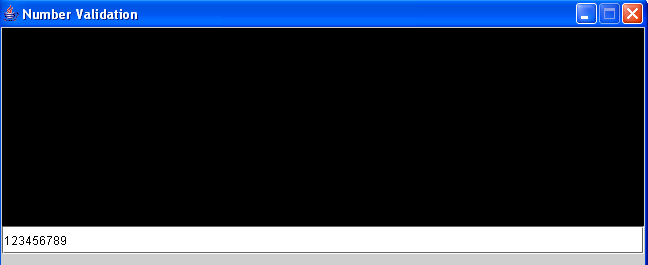
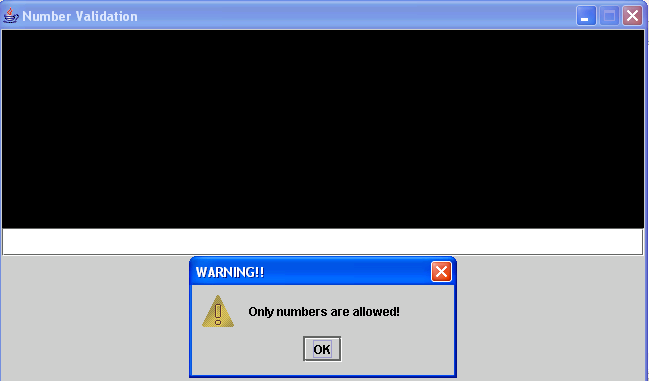
- import java.awt.*;
- import java.io.*;
- import javax.swing.*;
- import java.util.*;
- import java.awt.event.*;
- String dialogmessage;
- String dialogs;
- public static int record;
- {
- public j2()
- {
- }
- {
- super.paint(g);
- g.drawString("Number Validation",60,70);
- }
- }
- public numberValidation()
- {
- super("Number Validation ");
- panel1.add(txtNum);
- panel1.setOpaque(true);
- getContentPane().add(new j2(),"NORTH");
- getContentPane().add(panel1,"CENTER");
- Numvalidator(txtNum);
- setDefaultCloseOperation(DISPOSE_ON_CLOSE);
- }
- {
- char c = e.getKeyChar();
- e.consume();
- JOptionPane.showMessageDialog(null, "Only numbers are allowed!","WARNING!!",JOptionPane.WARNING_MESSAGE);
- }
- }
- });
- }
- numberValidation p1= new numberValidation();
- p1.setSize(650,630);
- p1.setLocation(300,50);
- p1.setVisible(true);
- p1.setResizable(false);
- }
- }
Add new comment
- 1598 views