Bubble Sort in C# Console
Submitted by donbermoy on Monday, July 14, 2014 - 09:48.
Today in C#, I will teach you how to create a program for bubble sorting using C# console. We all know that bubble sort is a sorting algorithm that is repeatedly searching through lists that need to be sorted, comparing each pair of items and swapping them if they are in the wrong order.
Now, let's start this tutorial!
1. Let's start with creating a Console Application in C# for this tutorial by following the following steps in Microsoft Visual Studio 2010: Go to File, click New Project, and choose Console Application.
2. Name your project as bubbleSort as your module.
Note: This is a console application so we cannot have visual controls for this tutorial.
3. Add the following code in your module.
Make a function named sorting. This will automatically sort the inputted elements that we will create in Sub Main.
5. For entering number of elements, put this code below.
6. For printing the inputted elements above, put this code below.
7. Lastly, we will code for the sorting of elements (bubble sort), put this code below.
Full source code:
Output:
For more inquiries and need programmer for your thesis systems in any kind of programming languages, just contact my number below.
Engr. Lyndon Bermoy
IT Instructor/System Developer/Android Developer/Freelance Programmer
If you have some queries, feel free to contact the number or e-mail below.
Mobile: 09488225971
Landline: 826-9296
E-mail:[email protected]
Add and Follow me on Facebook: https://www.facebook.com/donzzsky
Visit and like my page on Facebook at: https://www.facebook.com/BermzISware
- static public void sorting(int[] x, int y)
- {
- int i = default(int);
- int a = default(int);
- int t = default(int);
- for (i = 0; i <= y - 1; i++)
- {
- for (a = i + 1; a <= y - 1; a++)
- {
- if (x[i] > x[a])
- {
- t = x[i];
- x[i] = x[a];
- x[a] = t;
- }
- }
- }
- }
- Console.WriteLine("Bubble Sorting");
- Console.WriteLine();
- int num = default(int);
- int i = default(int);
- Console.Write("Enter Number of Elements: ");
- num = int.Parse(Console.ReadLine());
- Console.WriteLine();
- for (i = 0; i <= num - 1; i++)
- {
- Console.Write("Enter Element(" + (i + 1) + "): ");
- arr[i] = int.Parse(Console.ReadLine());
- }
- Console.WriteLine();
- Console.WriteLine("Inputted Elements");
- Console.WriteLine();
- for (i = 0; i <= num - 1; i++)
- {
- Console.WriteLine("Element in (" + i.ToString() + "): " + arr[i]);
- }
- Console.WriteLine();
- sorting(arr, num);
- Console.WriteLine("Sorted Elements");
- Console.WriteLine();
- for (i = 0; i <= num - 1; i++)
- {
- Console.WriteLine("Element in (" + i.ToString() + "): " + arr[i]);
- }
- Console.ReadLine();
- }
- Console.WriteLine();
- sorting(arr, num);
- Console.WriteLine("Sorted Elements");
- Console.WriteLine();
- for (i = 0; i <= num - 1; i++)
- {
- Console.WriteLine("Element in (" + i.ToString() + "): " + arr[i]);
- }
- Console.ReadLine();
- }
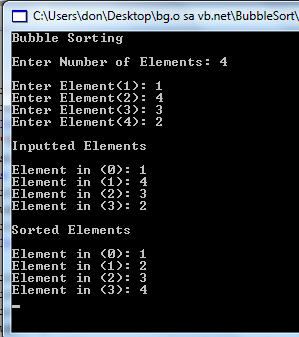
Add new comment
- 271 views