Display Directory Structure using TreeView in C#
Submitted by donbermoy on Tuesday, July 1, 2014 - 10:23.
Today in C#, i will teach you on how to create a program that loads and displays the directory structure of C using the TreeView control in C#.
So, now let's start this tutorial!
1. Let's start with creating a Windows Form Application in C# for this tutorial by following the following steps in Microsoft Visual Studio: Go to File, click New Project, and choose Windows Application and named your project as TreeViewDirectory.
2. Next, add only one TreeView1 named TreeView11 to display the contents of C directory structure. You must design your interface like this:
3. Add Using System.IO to the topmost part of the code tab because we will access the directory.
4. Create a method named PopulateTreeView with directoryValue As String and parentNode As TreeNode as parameters.
On this, create a try and catch method. In the try method, create an array variable (string) named directoryArray that holds and gets the directory either C or D.
Now, create an If statement that if your computer has obtained a directory this will add all the directory folders to the treeview using For Next Loop.
In the catch method, put this code below to handle UnauthorizedAccessException and will prompt the user "Access Denied".
5. Add this code for the form_load of your program. This will load the C directory structure using the PopulateTreeView method that we have created above.
Full source code:
For more inquiries and need programmer for your thesis systems in any kind of programming languages, just contact my number below.
Best Regards,
Engr. Lyndon Bermoy
IT Instructor/System Developer/Android Developer/Freelance Programmer
If you have some queries, feel free to contact the number or e-mail below.
Mobile: 09488225971
Landline: 826-9296
E-mail:[email protected]
Add and Follow me on Facebook: https://www.facebook.com/donzzsky
Visit and like my page on Facebook at: https://www.facebook.com/BermzISware
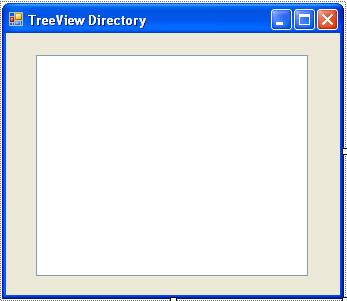
- using System.Diagnostics;
- using System;
- using System.Windows.Forms;
- using System.Collections;
- using System.Drawing;
- using System.Data;
- using System.Collections.Generic;
- using System.IO;
- public void PopulateTreeView(string directoryValue, TreeNode parentNode)
- {
- try
- {
- string[] directoryArray = Directory.GetDirectories(directoryValue);
- if (directoryArray.Length != 0)
- {
- string currentDirectory = default(string);
- foreach (string tempLoopVar_currentDirectory in directoryArray)
- {
- currentDirectory = tempLoopVar_currentDirectory;
- parentNode.Nodes.Add(myNode);
- }
- }
- }
- catch (UnauthorizedAccessException)
- {
- parentNode.Nodes.Add("Access Denied");
- }
- public void Form1_Load(System.Object sender, System.EventArgs e)
- {
- TreeView1.Nodes.Add("C:");
- PopulateTreeView("C:\\", TreeView1.Nodes[0]);
- }
- pub
- using System.Diagnostics;
- using System;
- using System.Windows.Forms;
- using System.Collections;
- using System.Drawing;
- using System.Data;
- using System.Collections.Generic;
- using System.IO;
- namespace TreeViewDirectory
- {
- public partial class Form1
- {
- public Form1()
- {
- InitializeComponent();
- }
- public void Form1_Load(System.Object sender, System.EventArgs e)
- {
- TreeView1.Nodes.Add("C:");
- PopulateTreeView("C:\\", TreeView1.Nodes[0]);
- }
- public void PopulateTreeView(string directoryValue, TreeNode parentNode)
- {
- try
- {
- string[] directoryArray = Directory.GetDirectories(directoryValue);
- if (directoryArray.Length != 0)
- {
- string currentDirectory = default(string);
- foreach (string tempLoopVar_currentDirectory in directoryArray)
- {
- currentDirectory = tempLoopVar_currentDirectory;
- parentNode.Nodes.Add(myNode);
- }
- }
- }
- catch (UnauthorizedAccessException)
- {
- parentNode.Nodes.Add("Access Denied");
- }
- }
- }
- }
Add new comment
- 554 views