Rock-Paper Scissor Game in C#
Submitted by donbermoy on Friday, June 27, 2014 - 00:19.
In this tutorial, i will teach you how to create a game program called Rock-Paper Scissor Game in C#. A rock-paper-scissor game is one of the most popular kiddie games here in Philippines. It is a simple game played around the world with many names and variations. It's a good way to decide whose turn it is to do something, and it's also played competitively. And today we will make this game and compete with a computer player.
Now, let's start this tutorial!
1. Let's start with creating a Windows Form Application in C# for this tutorial by following the following steps in Microsoft Visual Studio: Go to File, click New Project, and choose Windows Application.
2. Next, add one Combobox named ComboBox1, three labels named Label1 labeled as “Current Choice:”, Label2 labeled as “PC Choice:” and Label3 as “ ”. Add also one button named Button1 for us to know who won the game. You must design your interface like this:
3. Add this code as your Form_Load. This will add the choices of Rock, Paper, and Scissor in our combobox as the input of the player.
4. Now put this code for your code module. This code is for Button1_Click. This will trigger to decide who won the game.
We have initialized r variable as our random number, rr variable that will hold an integer variable of 3 as the ccChoice variable will hold this random integer value and will display the random choice in Label3. We also initialized variable uChoice as an Integer that will locate the inputted index of the Combobox. If the random input of cChoice is the same with variable uChoice, then it will display a draw. Otherwise if the random integer value of uChoice is 0 which means Rock and the inputted index value of the user in the combobox is 1 which means Paper then it will display “You Won” otherwise “You Lose” will display. If the random integer value of uChoice is 1 which means Paper and the inputted index value of the user in the combobox is 0 which means Rock then it will display “You Won” otherwise “You Lose” will display. And otherwise it will trigger to the result value if one them inputted a scissor.
Output:
For more inquiries and need programmer for your thesis systems in any kind of programming languages, just contact my number below.
Best Regards,
Engr. Lyndon Bermoy
IT Instructor/System Developer/Android Developer/Freelance Programmer
If you have some queries, feel free to contact the number or e-mail below.
Mobile: 09488225971
Landline: 826-9296
E-mail:[email protected]
Add and Follow me on Facebook: https://www.facebook.com/donzzsky
Visit and like my page on Facebook at: https://www.facebook.com/BermzISware
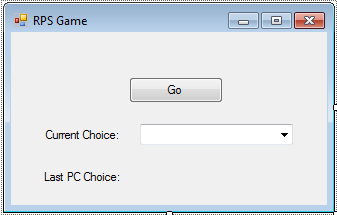
- public void Form1_Load(System.Object sender, System.EventArgs e)
- {
- // add items to combobox
- ComboBox1.Items.Add("Rock");
- ComboBox1.Items.Add("Paper");
- ComboBox1.Items.Add("Scissors");
- }
- public void Button1_Click(System.Object sender, System.EventArgs e)
- {
- int rr = r.Next(3); //int variable that has 3 values
- int cChoice = rr; //holds the rr variable
- int uChoice = ComboBox1.SelectedIndex; // choice as selected in combobox
- string pcChoice = "Rock";
- if (cChoice == 1)
- {
- pcChoice = "Paper";
- }
- if (cChoice == 2)
- {
- pcChoice = "Scissors";
- }
- Label3.Text = pcChoice;
- if (cChoice == uChoice)
- {
- MessageBox.Show("Draw!");
- }
- else
- {
- if (cChoice == 0)
- {
- if (uChoice == 1)
- {
- MessageBox.Show("PC Choice: Rock" + Constants.vbNewLine + "You Win!");
- }
- else
- {
- MessageBox.Show("PC Choice: Rock" + Constants.vbNewLine + "You Lose!");
- }
- }
- else if (cChoice == 1)
- {
- if (uChoice == 0)
- {
- MessageBox.Show("PC Choice: Paper" + Constants.vbNewLine + "You Lose!");
- }
- else
- {
- MessageBox.Show("PC Choice: Paper" + Constants.vbNewLine + "You Win!");
- }
- }
- else
- {
- if (uChoice == 0)
- {
- MessageBox.Show("PC Choice: Scissors" + Constants.vbNewLine + "You Win!");
- }
- else
- {
- MessageBox.Show("PC Choice: Scissors" + Constants.vbNewLine + "You Lose!");
- }
- }
- }
- rr = default(int);
- uChoice = default(int);
- cChoice = default(int);
- }
- }
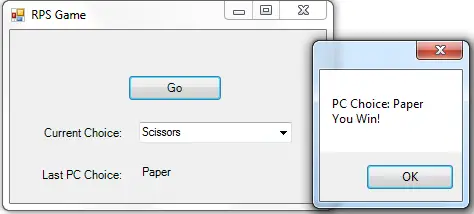
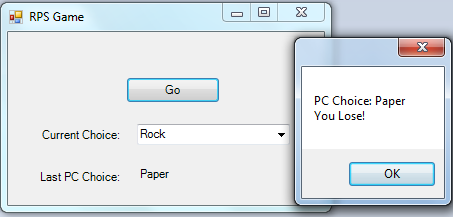
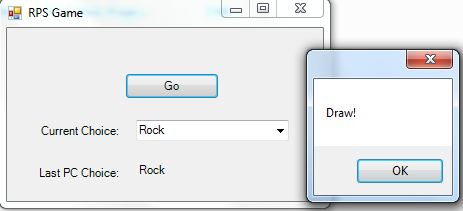
Add new comment
- 567 views