How To Create a Stopwatch in C#
Submitted by donbermoy on Saturday, June 21, 2014 - 19:00.
In this tutorial, I will teach you how to create a program that has a function of a digital stopwatch. Stopwatch is a timepiece that can be started or stopped for exact timing as of a race or any activity.
Now, let's start this tutorial!
1. Let's start with creating a Windows Form Application for this tutorial by following the following steps in Microsoft Visual Studio: Go to File, click New Project, and choose Windows Application.
2. Next, add four Buttons named Button1 and labeled it as "Start” for starting the time, Button 2 and labeled it as "Stop” for stopping the time, Button3 and labeled it as "Mark” for marking the time, and Button4 and labeled it as "Reset” for resetting the time back to 0. Insert a timer named Timer1 for creating the time, Label named Label1 for displaying the time, and ListView named ListView1 for displaying the marked time. You must design your interface like this:
3. First, we will code for Timer1. Put this code below.
We have first initialized variable StopWatch As New Diagnostics.Stopwatch. Stopwatch Class provides a set of methods and properties that you can use to accurately measure elapsed time.
We have initialized variable elapsed As TimeSpan which will be equal to the elapsed time of variable StopWatch. A TimeSpan object represents a time interval (duration of time or elapsed time) that is measured as a positive or negative number of days, hours, minutes, seconds, and fractions of a second. The TimeSpan structure can also be used to represent the time of day, but only if the time is unrelated to a particular date. We used Label1 as the timer that has the format like the real stopwatch. We have the format "{0:00}:{1:00}:{2:00}:{3:00}" for time. Math.Floor Method here returns the largest integer less than or equal to the specified double-precision floating-point number. It does have elapsed time for hours, minutes, seconds, and milliseconds.
4. Then we will code for Button1 to enable and start the timer and the stopwatch.
5. In Button2 as the stop button, put this code below to stop the time in the stopwatch.
6. In Button3 as marking the time or putting the time into the ListBox, put this code below.
7. For Button4 as reset button, put this code below to go back the time into 0 or restart it.
Full source code:
Output:
For more inquiries and need programmer for your thesis systems in any kind of programming languages, just contact my number below.
Best Regards,
Engr. Lyndon Bermoy
IT Instructor/System Developer/Android Developer/Freelance Programmer
If you have some queries, feel free to contact the number or e-mail below.
Mobile: 09488225971
Landline: 826-9296
E-mail:[email protected]
Add and Follow me on Facebook: https://www.facebook.com/donzzsky
Visit and like my page on Facebook at: https://www.facebook.com/BermzISware
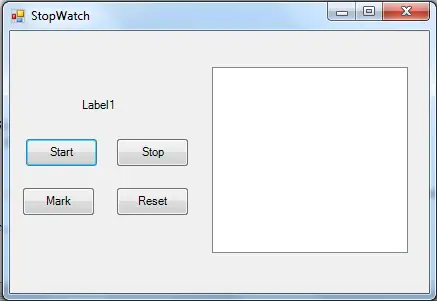
- public void Timer1_Tick(System.Object sender, System.EventArgs e)
- {
- TimeSpan elapsed = this.StopWatch.Elapsed;
- Label1.Text = string.Format("{0:00}:{1:00}:{2:00}:{3:00}", Math.Floor(elapsed.TotalHours), elapsed.Minutes, elapsed.Seconds, elapsed.Milliseconds);
- }
- public void Button1_Click(System.Object sender, System.EventArgs e)
- {
- Timer1.Start();
- this.StopWatch.Start();
- }
- public void Button1_Click(System.Object sender, System.EventArgs e)
- {
- Timer1.Start();
- this.StopWatch.Start();
- }
- public void Button3_Click_1(System.Object sender, System.EventArgs e)
- {
- ListBox1.Items.Add(Label1.Text);
- }
- public void Button4_Click(System.Object sender, System.EventArgs e)
- {
- this.StopWatch.Reset();
- Label1.Text = "00:00:00:000";
- ListBox1.Items.Clear();
- }
- using System.Diagnostics;
- using System;
- using System.Windows.Forms;
- using System.Collections;
- using System.Drawing;
- using System.Data;
- using System.Collections.Generic;
- namespace StopWatch
- {
- public partial class Form1
- {
- public Form1()
- {
- InitializeComponent();
- }
- public void Timer1_Tick(System.Object sender, System.EventArgs e)
- {
- TimeSpan elapsed = this.StopWatch.Elapsed;
- Label1.Text = string.Format("{0:00}:{1:00}:{2:00}:{3:00}", Math.Floor(elapsed.TotalHours), elapsed.Minutes, elapsed.Seconds, elapsed.Milliseconds);
- }
- public void Button1_Click(System.Object sender, System.EventArgs e)
- {
- Timer1.Start();
- this.StopWatch.Start();
- }
- public void Button2_Click(System.Object sender, System.EventArgs e)
- {
- Timer1.Stop();
- this.StopWatch.Stop();
- }
- public void Button4_Click(System.Object sender, System.EventArgs e)
- {
- this.StopWatch.Reset();
- Label1.Text = "00:00:00:000";
- ListBox1.Items.Clear();
- }
- public void Button3_Click_1(System.Object sender, System.EventArgs e)
- {
- ListBox1.Items.Add(Label1.Text);
- }
- }
- }
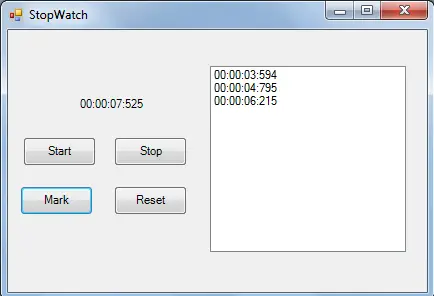
Add new comment
- 6439 views