CD/DVDROM Eject using C#
Submitted by donbermoy on Tuesday, June 3, 2014 - 19:51.
This is a tutorial in which we will going to create a program that will eject the CDROM or DVDROM using C# only.
Now, let's start this tutorial!
1. Let's start with creating a Windows Form Application in C# for this tutorial by following the following steps in Microsoft Visual Studio: Go to File, click New Project, and choose Windows Application and name the project CD eject.
2. Right Click ToolBox, click Choose Items, in the Com Components Tab, check "Windows Media Player" and then click Ok.
3. Next, add only one Button named Button1 and labeled it as "Eject". Insert a combobox named ComboBox1 for displaying the drive of the cdrom. You must design your interface like this:
4. Import AxWMPLib namespace as we access the Windows Media Player.
5. Declare this global variable in your code module. We will just use the WindowsMediaPlayer library to open the cdrom collection which hold the varCDrom variable.
6. In your Form_Load, copy the code below. This will show all the drives for CDROM to be displayed in the combobox. We will initialize first the totalCDDrive variable and i variable to 0. Then the totalCDDrive variable will be equal to number of cdrom drives. Then the cdrom drive will be displayed in the combobox.
7. Put this code in your Button1_Click. This will trigger to eject the drive you have chosen in the combobox. If the combobox is not empty, then the selected index cdrom drive in combobox is ejected as we have the property of cdromCollection has eject property.
Full source code:
Output:
Download the source code below and try it! :)
Best Regards,
Engr. Lyndon Bermoy
IT Instructor/System Developer/Android Developer/Freelance Programmer
If you have some queries, feel free to contact the number or e-mail below.
Mobile: 09488225971
Landline: 826-9296
E-mail:[email protected]
Add and Follow me on Facebook: https://www.facebook.com/donzzsky
Visit and like my page on Facebook at: https://www.facebook.com/BermzISware
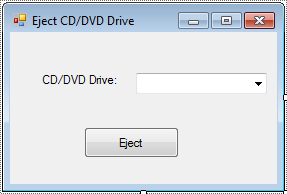
- using System.Diagnostics;
- using System;
- using System.Windows.Forms;
- using System.Collections;
- using System.Drawing;
- using System.Data;
- using System.Collections.Generic;
- using AxWMPLib;
- int totalCDDrive;
- int i;
- public void Form1_Load(System.Object sender, System.EventArgs e)
- {
- totalCDDrive = 0;
- i = 0;
- totalCDDrive = varCDrom.cdromCollection.count;
- if (totalCDDrive >= 1)
- {
- for (i = 0; i <= (totalCDDrive - 1); i++)
- {
- ComboBox1.Items.Add(varCDrom.cdromCollection.Item(i).driveSpecifier);
- }
- ComboBox1.Text = (string) (ComboBox1.Items[0]);
- }
- }
- public void Button1_Click_1(System.Object sender, System.EventArgs e)
- {
- if (!(ComboBox1.Text == ""))
- {
- varCDrom.cdromCollection.Item(ComboBox1.SelectedIndex).eject();
- }
- }
- using System.Diagnostics;
- using System;
- using System.Windows.Forms;
- using System.Collections;
- using System.Drawing;
- using System.Data;
- using System.Collections.Generic;
- using AxWMPLib;
- namespace CD_eject
- {
- public partial class Form1
- {
- public Form1()
- {
- InitializeComponent();
- }
- int totalCDDrive;
- int i;
- public void Form1_Load(System.Object sender, System.EventArgs e)
- {
- totalCDDrive = 0;
- i = 0;
- totalCDDrive = varCDrom.cdromCollection.count;
- if (totalCDDrive >= 1)
- {
- for (i = 0; i <= (totalCDDrive - 1); i++)
- {
- ComboBox1.Items.Add(varCDrom.cdromCollection.Item(i).driveSpecifier);
- }
- ComboBox1.Text = (string) (ComboBox1.Items[0]);
- }
- }
- public void Button1_Click_1(System.Object sender, System.EventArgs e)
- {
- if (!(ComboBox1.Text == ""))
- {
- varCDrom.cdromCollection.Item(ComboBox1.SelectedIndex).eject();
- }
- }
- }
- }
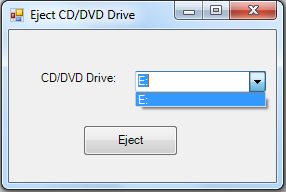
Add new comment
- 283 views