Count Number of Words using C#
Submitted by donbermoy on Friday, May 30, 2014 - 18:04.
This is a tutorial in which we will going to create a program that will count the number of words that we have inputted using C#.
Now, let's start this tutorial!
1. Let's start with creating a Windows Form Application in C# for this tutorial by following the following steps in Microsoft Visual Studio: Go to File, click New Project, and choose Windows Application and name your project as Word Count Program.
2. Next, add one RichTextBox named RichTextBox1 and a Button named Button1 labeled as "Get Number of Words". You must design your interface like this below:
3. Now put add this code for your code module. This code is for Button1_Click. This will trigger to count the number of words in your RichTextBox.
Initialize variable strInput as String to hold the value inputted in the RichTextBox.
Declare variable strSplit as string to split the words inputted in the RichTextBox. We use to split the words inputted to find the spacing of the text. so, that's how we count the words. Then we create a MessageBox to display the number of words using the Length function.
Full Source code:
Output:
Download the source code and try it!
Best Regards,
Engr. Lyndon Bermoy
IT Instructor/System Developer/Android Developer/Freelance Programmer
If you have some queries, feel free to contact the number or e-mail below.
Mobile: 09488225971
Landline: 826-9296
E-mail:[email protected]
Add and Follow me on Facebook: https://www.facebook.com/donzzsky
Visit and like my page on Facebook at: https://www.facebook.com/BermzISware
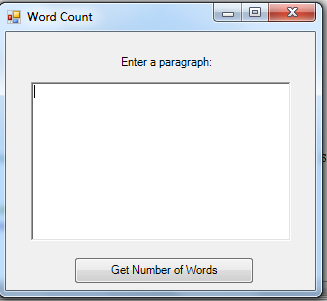
- string strInput = default(string);
- strInput = RichTextBox1.Text;
- string[] strSplit = null;
- strSplit = strInput.Split(' ');
- MessageBox.Show("Number of words: " + strSplit.Length);
- using System.Diagnostics;
- using System;
- using System.Windows.Forms;
- using System.Collections;
- using System.Drawing;
- using System.Data;
- using System.Collections.Generic;
- namespace Word_Count_Program
- {
- public partial class Form1
- {
- public Form1()
- {
- InitializeComponent();
- }
- public void Button1_Click(System.Object sender, System.EventArgs e)
- {
- string strInput = default(string);
- strInput = RichTextBox1.Text;
- string[] strSplit = null;
- strSplit = strInput.Split(' ');
- MessageBox.Show("Number of words: " + strSplit.Length);
- }
- }
- }
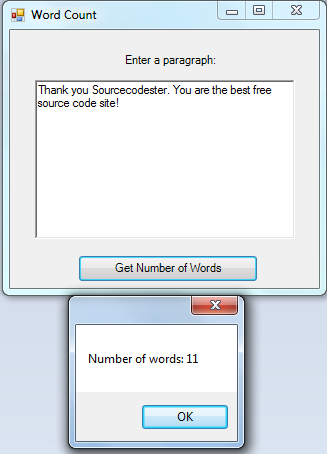
Add new comment
- 726 views