How to Write Data to a Text File using C#
Submitted by donbermoy on Monday, May 26, 2014 - 11:29.
In this tutorial, i will teach you how to create a program that writes data to a text file using C#. This is my continuation of my other tutorial entitled How to Read Data from a Text File using C#.
Now, let's start this tutorial!
1. Let's start with creating a Windows Form Application in C# for this tutorial by following the following steps in Microsoft Visual Studio: Go to File, click New Project, and choose Windows Application.
2. Next, add only one Button named btnSave and labeled it as "Save". Insert also one textbox named txtInput for inputting a text that we will write in the text file and a SaveFileDialog named SaveFileDialog1 to save it as a text file. You must design your interface like this:
3. In your btnSave, put this code below.
Filter your saveFileDialog1 to be saved as a text file or a .txt file extension. And add also a title to this control.
Now, create a code to show the dialog in the saveFileDialog1 for saving the text file.
Initialize the System.IO.StreamWriter having a File variable. This will write data to the saveFileDialog1 filename. We used the StreamWriter for writing a data to this file of saveFileDialog1.
Make a string variable named data that will hold the inputted text of txtInput.txt.
Code for writing data to the file.
Close your file.
Full source code:
Press F5 to run the program.
Input:
Save as sourcodester.txt.
Output:
Hope this helps! :)
Best Regards,
Engr. Lyndon Bermoy
IT Instructor/System Developer/Android Developer/Freelance Programmer
If you have some queries, feel free to contact the number or e-mail below.
Mobile: 09488225971
Landline: 826-9296
E-mail:[email protected]
Add and Follow me on Facebook: https://www.facebook.com/donzzsky
Visit and like my page on Facebook at: https://www.facebook.com/BermzISware
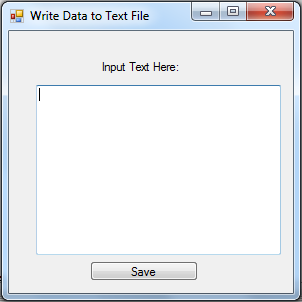
- saveFileDialog1.Filter = "Text Files|*.txt";
- saveFileDialog1.Title = "Write Data to Text File.";
- saveFileDialog1.ShowDialog();
- string data = txtInput.Text;
- file.WriteLine(data);
- file.Close();
- using System;
- using System.Collections.Generic;
- using System.ComponentModel;
- using System.Data;
- using System.Drawing;
- using System.Text;
- using System.Windows.Forms;
- namespace write_data_to_textfile_using_CSharp
- {
- public partial class Form1 : Form
- {
- public Form1()
- {
- InitializeComponent();
- }
- private void button1_Click(object sender, EventArgs e)
- {
- saveFileDialog1.Filter = "Text Files|*.txt";
- saveFileDialog1.Title = "Write Data to Text File.";
- saveFileDialog1.ShowDialog();
- string data = txtInput.Text;
- file.WriteLine(data);
- file.Close();
- }
- }
- }
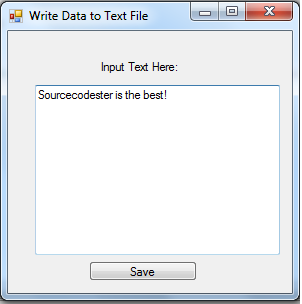
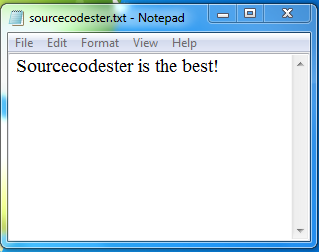
Add new comment
- 106 views