How to Save and Retrieve Data in the ListView Using C#
Submitted by janobe on Tuesday, August 27, 2019 - 22:41.
This time, I will teach you how to save and retrieve data in the listview using c# and MySql database. This is a simple method that you can do in no time. The program contains the following function such as saving data in the database and it will be retrieved in the listview control. Follow the step by step guide to know how the program works. I use Visual Studio 2015 to develop this program.
The complete source code is included. You can download it and run it on your computer.
For any questions about this article. You can contact me @
Email – [email protected]
Mobile No. – 09305235027 – TNT
Or feel free to comment below.
Creating a Database
Create a database in the “phpmyadmin” and named it “tuts_dbperson”. After that, execute the following query to create a table in the database that you have created.Creating Application
Step 1
Open Microsoft Visual Studio 2015 and create a new windows form application in c#.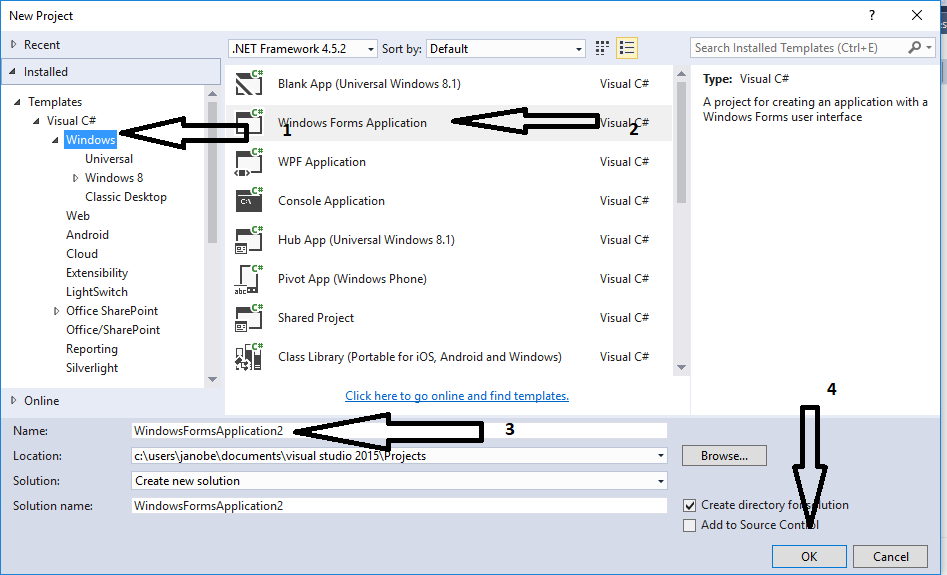
Step 2
Add a ListView, two buttons, two texboxes, and two labels after that do the form just like shown below.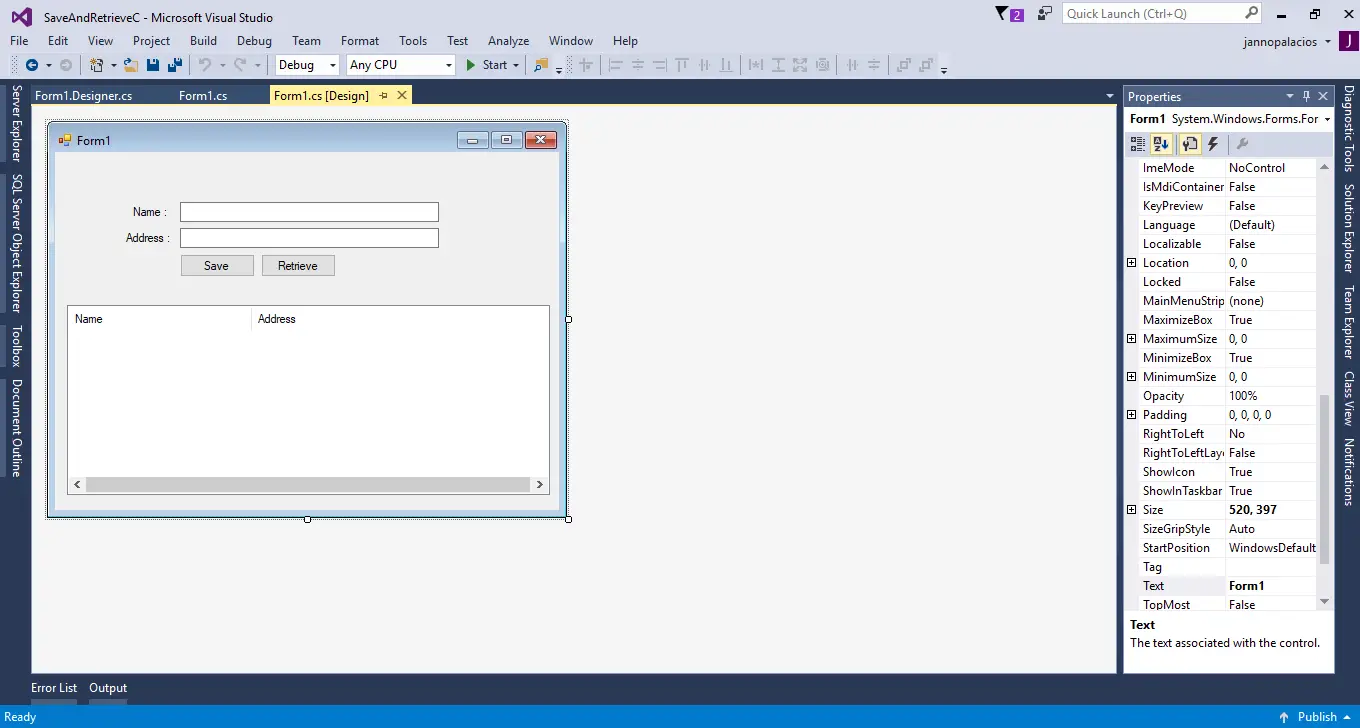
Step 3
Add MySQL.Data.dll as references.Step 4
Press F7 to open the code editor. In the code editor, add a namespace to access MySQL libraries.- using MySql.Data.MySqlClient;
Step 5
Create a connection between c# and MySQL database and declare all the classes that are needed.Step 6
Double click the “Save” button and do the following code for saving data in the database.- try
- {
- con.Open();
- sql = "INSERT INTO `tblperson` (`Fullname`, `Address`) VALUES('" + txtName .Text + "','"+ txtAddress .Text + "')";
- cmd.Connection = con;
- cmd.CommandText = sql;
- result = cmd.ExecuteNonQuery();
- if(result > 0)
- {
- MessageBox.Show("Data has been saved in the database");
- }
- else
- {
- MessageBox.Show("Error to execute the query");
- }
- }
- catch (Exception ex)
- {
- MessageBox.Show(ex.Message);
- }
- finally
- {
- con.Close();
- }
Step 5
Double click the “Retrieve” button and do the following code for retrieving data in the database.- try
- {
- con.Open();
- sql = "SELECT * FROM `tblperson`";
- cmd.Connection = con;
- cmd.CommandText = sql;
- da.SelectCommand = cmd;
- da.Fill(dt);
- listView1.Items.Clear();
- foreach(DataRow r in dt.Rows)
- {
- ListViewItem list = listView1.Items.Add(r.Field<string>(1));
- list.SubItems.Add(r.Field<string>(2));
- }
- }
- catch (Exception ex)
- {
- MessageBox.Show(ex.Message);
- }
- finally
- {
- con.Close();
- da.Dispose();
- }
Add new comment
- 1527 views