Filling Data in a ListBox Using C# and MySQL Database
Submitted by janobe on Thursday, April 4, 2019 - 22:11.
In this tutorial, I will teach you how to fill data in a ListBox using C# and MySQL database. This process will illustrate how to get the data in the database and display it on a ListBox. This operation will be a big help for you when you are dealing with a ListBox and MySQL Database.
The complete source code is included you can download it and run it on your computer.
For any questions about this article. You can contact me @
Email – [email protected]
Mobile No. – 09305235027 – TNT
Or feel free to comment below.
Creating Application
Step 1
Open Microsoft Visual Studio 2015 and create a new windows form application for c#.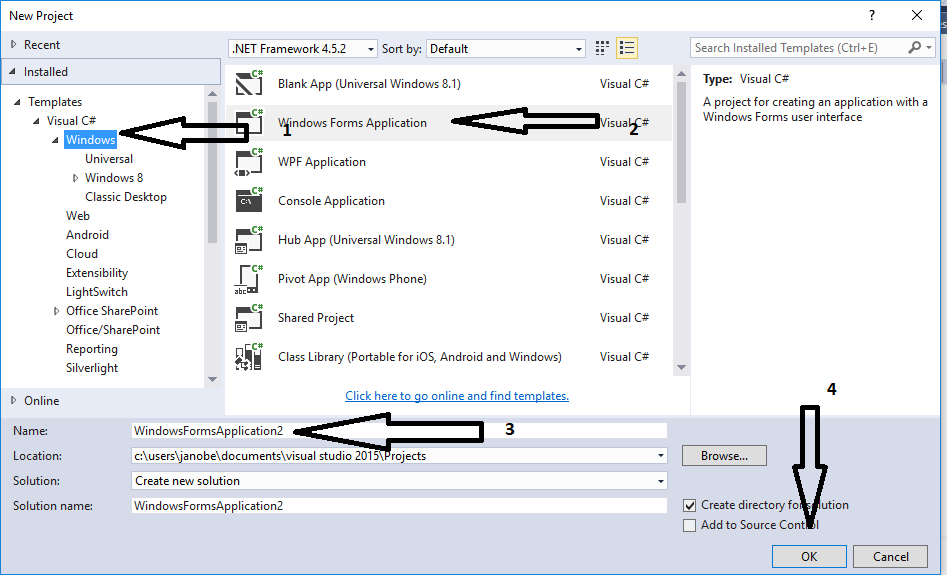
Step 2
Do the form just like shown below.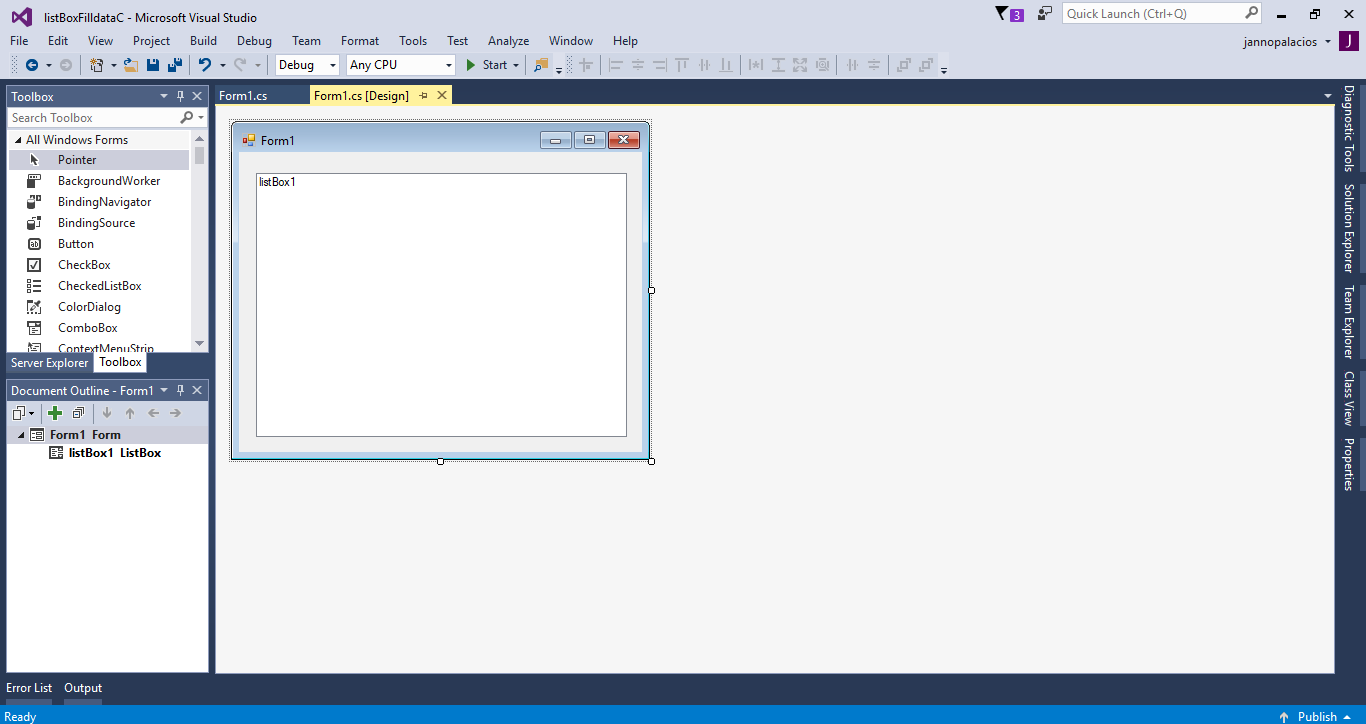
Step 3
Press F7 to open the code editor. In the code editor, add a namespace to accessMySQL
libraries
- using MySql.Data.MySqlClient;
Step 4
Create a connection between C# and MySQL database. After that, declare all the classes and variable that is needed.- MySqlConnection con = new MySqlConnection("server=localhost;user id=root;password=;database=dbpeople;sslMode=none");
- MySqlCommand cmd;
- MySqlDataAdapter da;
- DataTable dt;
- string sql;
Step 5
Create a method to fill the data inside a ListBox.- private void fill_data(string sql,ListBox lst)
- {
- try
- {
- con.Open();
- cmd.Connection = con;
- cmd.CommandText = sql;
- da.SelectCommand = cmd;
- da.Fill(dt);
- lst.DataSource = dt;
- lst.DisplayMember = "FNAME";
- }
- catch (Exception ex)
- {
- MessageBox.Show(ex.Message);
- }
- finally
- {
- con.Close();
- da.Dispose();
- }
- }
Step 6
Writ the following codes to execute the method in the first load of the form.- private void Form1_Load(object sender, EventArgs e)
- {
- sql = "SELECT FNAME FROM `tblperson`";
- fill_data(sql, listBox1);
- }
Add new comment
- 1753 views