Login and Logout Using C#.net and SQL Server 2005
Submitted by janobe on Tuesday, July 12, 2016 - 07:53.
In this tutorial, I will teach you how to create Login and Logout using C#.net and SQL Server 2005 Express Edition. This method is very helpful to ensure the security of the system from invaders who wants to access your system.
3. Make a query for inserting data in the table that you have made.
4. Open Microsoft Visual Studio and create a new windows form application for C#.
5. Do the Form just like this.
6. Go to the solution explorer and hit the "View Code" to display the code editor.
7. Declare all the classes and variables that are needed.
9. Do the following codes for the login method in the
10. Finally, DO the following codes for the logout method.
For all students who need a programmer for your thesis system or anyone who needs a source code in any programming languages. You can contact me @ :
Email – [email protected]
Mobile No. – 09305235027 – TNT
Let's begin:
1. Create a database in SQL Server 2005 Express Edition and name it "userdb". 2. Make a query for creating the table in the database.- /****** Object: Table [dbo].[tbluser] Script Date: 07/11/2016 11:58:48 ******/
- SET ANSI_NULLS ON
- GO
- SET QUOTED_IDENTIFIER ON
- GO
- CREATE TABLE [dbo].[tbluser](
- [ID] [INT] IDENTITY(1,1) NOT NULL,
- [Name] [nvarchar](50) NULL,
- [UNAME] [nvarchar](50) NULL,
- [PASS] [nvarchar](MAX) NULL,
- [UTYPE] [NCHAR](20) NULL,
- CONSTRAINT [PK_tbluser] PRIMARY KEY CLUSTERED
- (
- [ID] ASC
- )WITH (PAD_INDEX = OFF, STATISTICS_NORECOMPUTE = OFF, IGNORE_DUP_KEY = OFF, ALLOW_ROW_LOCKS = ON, ALLOW_PAGE_LOCKS = ON) ON [PRIMARY]
- ) ON [PRIMARY]
- INSERT INTO [dbo].[tbluser]
- (Name
- ,UNAME
- ,PASS
- ,UTYPE)
- VALUES ('Janno Palacios',janobe,janobe,'Administrator')
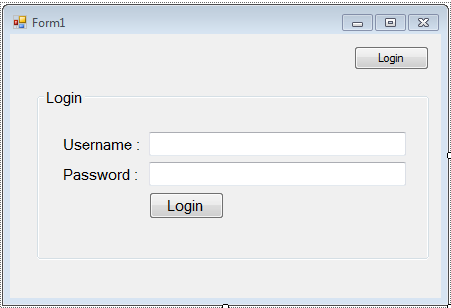
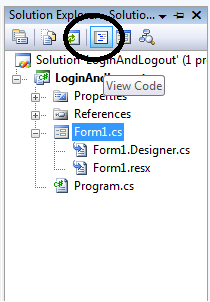
8. Do the following codes for establishing the connection between SQL Server and C#.net.
- //set a connection between SQL server and Visual C#
- conn.ConnectionString = "Data Source=.\\SQLEXPRESS;database=userdb;trusted_connection=true;";
click
event handler of a "Login" button
- try
- {
- conn.Open();
- //create a query for retrieving data in the database.
- strquery = "SELECT * FROM tbluser WHERE UNAME='" + txtUsername.Text + "' and PASS='" + txtPassword.Text + "'";
- //initialize new Sql commands
- //hold the data to be executed.
- cmd.Connection = conn;
- cmd.CommandText = strquery;
- //initialize new Sql data adapter
- //fetching query in the database.
- da.SelectCommand = cmd;
- //initialize new datatable
- //refreshes the rows in specified range in the datasource.
- da.Fill(dt);
- //getting the total row in the table.
- max= dt.Rows.Count;
- //validating the total rows in the table
- if (max > 0)
- {
- //popup message
- MessageBox.Show("Welcome " + dt.Rows[0].Field<string>("UTYPE"));
- //disable the group box.
- groupBox1.Enabled = false;
- //store the value in the Button
- btnLogin.Text = "Logout";
- //clearing textboxes
- txtPassword.Clear();
- txtUsername.Clear();
- }
- else
- {
- //when the account does not exist, it will display this message.
- MessageBox.Show("Account does not exist.");
- }
- }
- catch (Exception ex)
- {
- //catching error
- MessageBox.Show(ex.Message);
- }
- finally
- {
- da.Dispose();
- conn.Close();
- }
- if (btnLogin.Text == "Logout")
- {
- groupBox1.Enabled = true;
- txtUsername.Clear();
- txtPassword.Clear();
- btnLogin.Text = "Login";
- }
- else
- {
- groupBox1.Enabled = true ;
- txtUsername.Clear();
- txtPassword.Clear();
- btnLogin .Text = "Login";
- }
Add new comment
- 750 views