How to Accept String that Start with a Vowel in Python
In this tutorial, we will learn how to program "How to Accept a String that Starts with a Vowel in Python." We will check if the given string begins with a vowel. The objective is to safely and accurately validate if the entered string starts with a vowel. A sample program will be provided to demonstrate the coding process.
This topic is easy to understand. Just follow the instructions I provide, and you’ll be able to do it yourself with ease. The program I’ll show you demonstrates the correct way to validate if a string starts with a vowel. I’ll also provide a simple and efficient method to achieve this. So, let’s start coding!
Getting Started:
First you will have to download & install the Python IDLE's, here's the link for the Integrated Development And Learning Environment for Python https://www.python.org/downloads/.
Creating Main Function
This is the main function of the application. The following code will display a simple GUI in terminal console that will display program. To do this, simply copy and paste these blocks of code into the IDLE text editor.- import re
- regex = '^[aeiouAEIOU][A-Za-z0-9_]*'
- def check(string):
- if(re.search(regex, string)):
- print("This string is Valid!")
- else:
- print("This string is not Invalid!")
- if __name__ == '__main__' :
- while True:
- print("\n================= Accept String that Start with a Vowel =================\n\n")
- string = input("Enter a string: ")
- check(string)
- opt = input("\nDo you want to try again?(yes/no): ")
- if opt.lower() == 'yes':
- ret=False
- elif opt.lower() == 'no':
- ret=True
- print("Exiting program....")
- else:
- print("Please enter yes/no:")
- break
- if ret == False:
- continue
This script checks if a given string starts with a vowel, followed by any combination of letters, numbers, or underscores. If the string meets these criteria, the program responds, "This string is Valid!" Otherwise, it responds, "This string is not Invalid!" The program then asks if the user wants to check another string, continuing until the user chooses to stop.
Output:
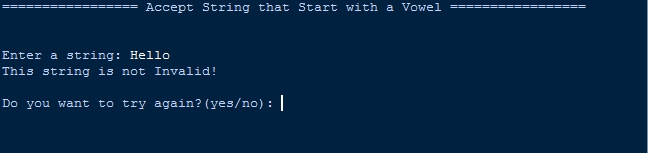
There you have it we successfully created How to Accept String that Start with a Vowel in Python. I hope that this simple tutorial help you to what you are looking for. For more updates and tutorials just kindly visit this site. Enjoy Coding!
More Tutorials for Python Language
Add new comment
- 12 views