Focus when Mouse Hover in Java
Submitted by donbermoy on Wednesday, December 17, 2014 - 00:35.
Language
This tutorial will teach you how to create a program that when hovering a mouse it will focus on the button using java.
So, now let's start this tutorial!
1. Open JCreator or NetBeans and make a java program with a file name of focusSample.java.
2. Import the following package library:
3. Create a class name MouseHover that will extend a MouseAdapter to access a component so that it can request focus. We will use the JComponent class so that it will call the component to be focused.
4. We will initialize variables in our Main, variable frame as JFrame, and mouseListener as the MouseHover class that we have declared above.
Now, we will create 6 buttons and will apply the Focus that we have declared the MouseHover class. We will use the setFocusable method of the buttons so that it can be focused.
Output:
Here's the full code of this tutorial:
For more inquiries and need programmer for your thesis systems in any kind of programming languages, just contact my number below.
Best Regards,
Engr. Lyndon Bermoy
IT Instructor/System Developer/Android Developer/Freelance Programmer
Mobile: 09488225971
Landline: 826-9296
E-mail:[email protected]
Add and Follow me on Facebook: https://www.facebook.com/donzzsky
Visit and like my page on Facebook at: https://www.facebook.com/BermzISware
- import java.awt.*; //used to access a Component and GridLayout class
- import java.awt.event.*; //used to access MouseAdapter,MouseEvent, and MouseListener class
- import javax.swing.*; //used to access JButton and JFrame class
- if (!component.hasFocus()) {
- component.requestFocusInWindow();
- }
- }
- }
5. Lastly, set the size and visibility to true, and close its operation. Have this code below:
- frame.setSize(300, 200);
- frame.setVisible(true);
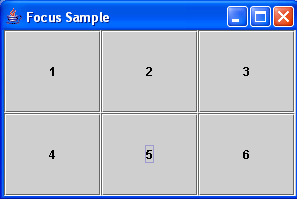
- import java.awt.*; //used to access a Component and GridLayout class
- import java.awt.event.*; //used to access MouseAdapter,MouseEvent, and MouseListener class
- import javax.swing.*; //used to access JButton and JFrame class
- if (!component.hasFocus()) {
- component.requestFocusInWindow();
- }
- }
- }
- public class focusSample{
- for (int i = 1; i <= 6; i++) {
- button.addMouseListener(mouseListener);
- button.setFocusable(true);
- frame.getContentPane().add(button);
- }
- frame.setSize(300, 200);
- frame.setVisible(true);
- }
- }
Note: Due to the size or complexity of this submission, the author has submitted it as a .zip file to shorten your download time. After downloading it, you will need a program like Winzip to decompress it.
Virus note: All files are scanned once-a-day by SourceCodester.com for viruses, but new viruses come out every day, so no prevention program can catch 100% of them.
FOR YOUR OWN SAFETY, PLEASE:
1. Re-scan downloaded files using your personal virus checker before using it.
2. NEVER, EVER run compiled files (.exe's, .ocx's, .dll's etc.)--only run source code.
Add new comment
- 536 views