Today in C#, i will teach you how to create a program called Slot Machine Game.
Now, let's start this tutorial!
1. Let's start with creating a Windows Form Application in C# for this tutorial by following the following steps in Microsoft Visual Studio: Go to File, click New Project, and choose Windows Application.
2. Next, add only one Button named Button1 and labeled it as "SPIN". Insert three PictureBox named PictureBox1,PictureBox2, and PictureBox3. Add also a timer named Timer1. You must design your interface like this:
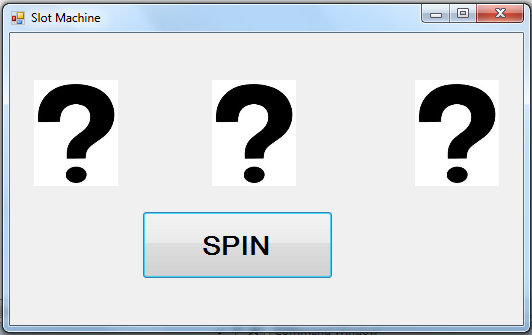
3. Insert the following image files to the resources of your project.
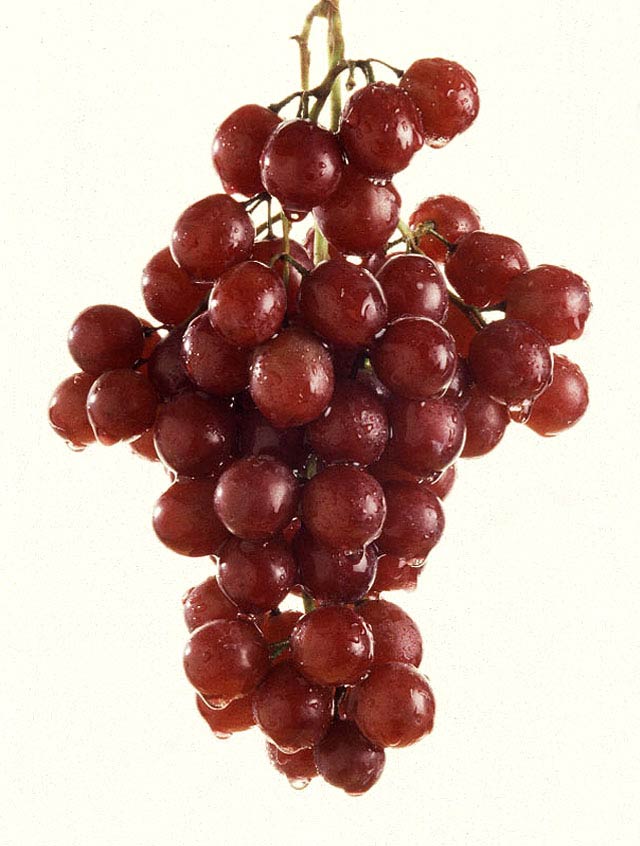
4. Put this code in your code module.
using System.Diagnostics;
using System;
using System.Windows.Forms;
using System.Collections;
using System.Drawing;
using Microsoft.VisualBasic;
using System.Data;
using System.Collections.Generic;
namespace Slot_Machine
{
public partial class Form1 : Form
{
public Form1()
{
InitializeComponent();
}
// initialize global variables
int m;
int a;
int b;
int c;
private void Button1_Click(object sender, EventArgs e)
{
// make timer enabled to true
timer1.Enabled = true;
}
private void Form1_Load(object sender, EventArgs e)
{
// make timer enabled to stop time
timer1.Enabled = false;
}
private void timer1_Tick(object sender, EventArgs e)
{
// variable m will increment by 10
m = m + 10;
// if m will be equal to 1000 and above
if (m <= 1000)
{
// randomize variable a
a = (int)(Conversion.Int(1 + VBMath.Rnd() * 3));
// randomize variable b
b = (int)(Conversion.Int(1 + VBMath.Rnd() * 3));
c = (int)(Conversion.Int(1 + VBMath.Rnd() * 3));
// randomize variable c
//contents of variable a
switch (a)
{
case 1:
//path of the image file of apple
PictureBox1.Image = Image.FromFile("C:\\Users\\don\\Desktop\\bg.o sa vb.net\\Slot Machine\\Slot Machine\\Resources\\apple.jpg");
break;
//path of the image file of grapes
case 2:
PictureBox1.Image = Image.FromFile("C:\\Users\\don\\Desktop\\bg.o sa vb.net\\Slot Machine\\Slot Machine\\Resources\\grapes.jpg");
break;
//path of the image file of strawberry
case 3:
PictureBox1.Image = Image.FromFile("C:\\Users\\don\\Desktop\\bg.o sa vb.net\\Slot Machine\\Slot Machine\\Resources\\strawberry.jpg");
break;
}
//contents of b variable
switch (b)
{
//path of the image file of apple
case 1:
PictureBox2.Image = Image.FromFile("C:\\Users\\don\\Desktop\\bg.o sa vb.net\\Slot Machine\\Slot Machine\\Resources\\apple.jpg");
break;
//path of the image file of grapes
case 2:
PictureBox2.Image = Image.FromFile("C:\\Users\\don\\Desktop\\bg.o sa vb.net\\Slot Machine\\Slot Machine\\Resources\\grapes.jpg");
break;
//path of the image file of strawberry
case 3:
PictureBox2.Image = Image.FromFile("C:\\Users\\don\\Desktop\\bg.o sa vb.net\\Slot Machine\\Slot Machine\\Resources\\strawberry.jpg");
break;
}
//contents of variable c
switch (c)
{
//path of the image file of apple
case 1:
PictureBox3.Image = Image.FromFile("C:\\Users\\don\\Desktop\\bg.o sa vb.net\\Slot Machine\\Slot Machine\\Resources\\apple.jpg");
break;
//path of the image file of grapes
case 2:
PictureBox3.Image = Image.FromFile("C:\\Users\\don\\Desktop\\bg.o sa vb.net\\Slot Machine\\Slot Machine\\Resources\\grapes.jpg");
break;
//path of the image file of strawberry
case 3:
PictureBox3.Image = Image.FromFile("C:\\Users\\don\\Desktop\\bg.o sa vb.net\\Slot Machine\\Slot Machine\\Resources\\strawberry.jpg");
break;
}
}
else
{
// after reaching m variable to 1000 the timer will be disabled
timer1.Enabled = false;
// m variable will be back to 0
m = 0;
// if contents of a will be equal to b and c, you will win the prize
if (System.Convert.ToInt32(a == b) == c)
{
lblMsg.Text = "Jackpot! You won P1,000,000″";
}
// otherwise, you've lost
else
{
lblMsg.Text = "No luck, try again";
}
}
}
}
}
Output:
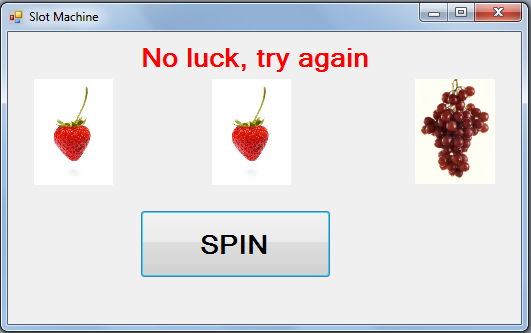
For more inquiries and need programmer for your thesis systems in any kind of programming languages, just contact my number below.
Best Regards,
Engr. Lyndon Bermoy
IT Instructor/System Developer/Android Developer/Freelance Programmer
If you have some queries, feel free to contact the number or e-mail below.
Mobile: 09488225971
Landline: 826-9296
E-mail:
[email protected]
Add and Follow me on Facebook:
https://www.facebook.com/donzzsky
Visit and like my page on Facebook at:
https://www.facebook.com/BermzISware