How to Send Mail To Gmail Using PHPMailer
In this tutorial we will create a Sending Email Using PHPMailer. PHP is a server-side scripting language designed primarily for web development. Using PHP, you can let your user directly interact with the script and easily to learned its syntax. It is mostly used by a newly coders for its user-friendly environment.
So Let's do the coding...Getting Started:
First you have to download & install XAMPP or any local server that run PHP scripts. Here's the link for XAMPP server https://www.apachefriends.org/index.html.
And, this is the link for the bootstrap that i used for the layout design https://getbootstrap.com/.
Installing the PHPMailer
Using ComposerIf you dont' have a composer installed on your machine you can get it here, https://getcomposer.org/download/
First, Create a file that will receive the phpmailer file, write these code inside the text editor and save it as composer.json
.
- {
- "phpmailer/phpmailer": "~6.0"
- }
Then, open your command prompt and cd to your working directory. After that, type this command and hit enter afterward.
- composer require phpmailer/phpmailer
After installation you will see a different directory inside your working directory, namely vendor, etc.
Without ComposerTo manually install the phpmailer to your app, first download the file here https://github.com/PHPMailer/PHPMailer
After you finish downloading the file, move the file inside the directory that you are working on. Now you're ready to use the phpmailer.
Creating The Interface
This is where we will create a simple form for our application. To create the forms simply copy and write it into your text editor, then save it as index.php
- <!doctype html>
- <html lang="en">
- <head>
- <meta charset="UTF-8" name="viewport" content="width=device-width, initial-scale=1"/>
- <link rel="stylesheet" type="text/css" href="css/bootstrap.css"/>
- </head>
- <body>
- <nav class="navbar navbar-default">
- <div class="container-fluid">
- <a class="navbar-brand" href="https://sourcecodester.com">Sourcecodester</a>
- </div>
- </nav>
- <div class="col-md-3"></div>
- <div class="col-md-6 well">
- <h3 class="text-primary">PHP - Send Mail To Gmail Using PHPMailer</h3>
- <hr style="border-top:1px dotted #ccc;"/>
- <div class="col-md-3"></div>
- <div class="col-md-6">
- <form method="POST" action="send_email.php">
- <div class="form-group">
- <label>Email:</label>
- <input type="email" class="form-control" name="email" required="required"/>
- </div>
- <div class="form-group">
- <label>Subject</label>
- <input type="text" class="form-control" name="subject" required="required"/>
- </div>
- <div class="form-group">
- <label>Message</label>
- <input type="text" class="form-control" name="message" required="required"/>
- </div>
- <center><button name="send" class="btn btn-primary"><span class="glyphicon glyphicon-envelope"></span> Send</button></center>
- </form>
- <br />
- <?php
- if($_SESSION['status'] == "ok"){
- ?>
- <div class="alert alert-info"><?php echo $_SESSION['result']?></div>
- <?php
- }else{
- ?>
- <div class="alert alert-danger"><?php echo $_SESSION['result']?></div>
- <?php
- }
- }
- ?>
- </div>
- </div>
- </body>
- </html>
Creating the Main Function
This is the main function of the application. This code will send requests via phpmailer to be able to send mail to google SMTP. To do that copy and write these inside the text editor, then save it as send_email.php
To make this script work without composer, make sure you change this code:
- require 'vendor/autoload.php';
- require 'path/to/PHPMailer/src/Exception.php';
- require 'path/to/PHPMailer/src/PHPMailer.php';
- require 'path/to/PHPMailer/src/SMTP.php';
So that it will recognize the phpmailer class when you call it in the code.
- <?php
- use PHPMailer\PHPMailer\PHPMailer;
- use PHPMailer\PHPMailer\Exception;
- $email = $_POST['email'];
- $subject = $_POST['subject'];
- $message = $_POST['message'];
- //Load composer's autoloader
- require 'vendor/autoload.php';
- $mail = new PHPMailer(true);
- try {
- //Server settings
- $mail->isSMTP();
- $mail->Host = 'smtp.gmail.com';
- $mail->SMTPAuth = true;
- $mail->Password = 'mypassword';
- 'verify_peer' => false,
- 'verify_peer_name' => false,
- 'allow_self_signed' => true
- )
- );
- $mail->SMTPSecure = 'ssl';
- $mail->Port = 465;
- //Send Email
- //Recipients
- $mail->addAddress($email);
- //Content
- $mail->isHTML(true);
- $mail->Subject = $subject;
- $mail->Body = $message;
- $mail->send();
- $_SESSION['result'] = 'Message has been sent';
- $_SESSION['status'] = 'ok';
- } catch (Exception $e) {
- $_SESSION['result'] = 'Message could not be sent. Mailer Error: '.$mail->ErrorInfo;
- $_SESSION['status'] = 'error';
- }
- }
- ?>
Note: To be able to send a mail to Gmail, make sure you provide your own Gmail username and password or much better is to make a dummy account to use.
Inside your Google account make sure you allow the less secure apps so that you can have permission to send the email.
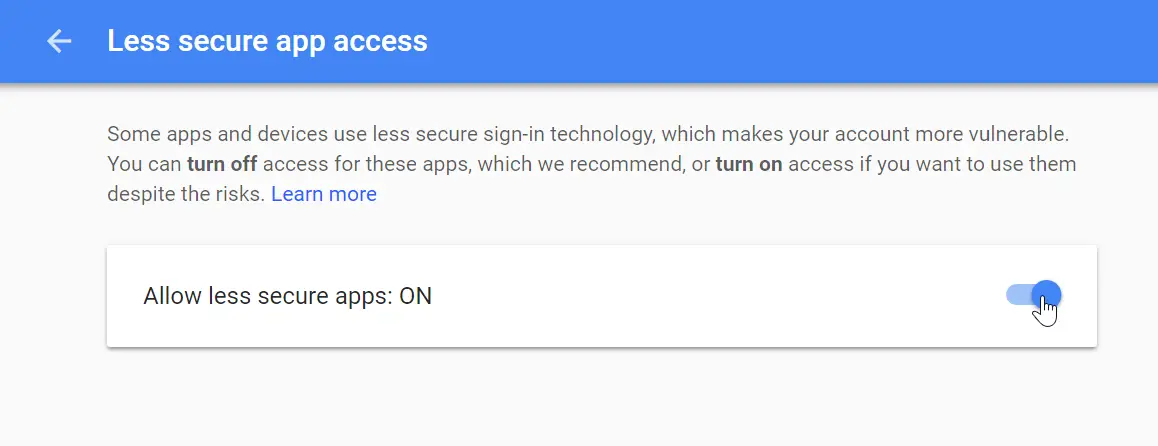
There you have it we successfully created a Send Mail To Gmail Using PHPMailer. I hope that this simple tutorial helps you with your projects. For more updates and tutorials just kindly visit this site.
Enjoy Coding!Comments
Add new comment
- Add new comment
- 8073 views