Today in VB.NET, i will teach you on how to create a program that loads and displays the directory structure of C using the TreeView control in VB.NET.
So, now let's start this tutorial!
1. Let's start with creating a Windows Form Application in Visual Basic for this tutorial by following the following steps in Microsoft Visual Studio: Go to
File, click
New Project, and choose
Windows Application.
2. Next, add only one TreeView1 named
TreeView11 to display the contents of C directory structure. You must design your interface like this:
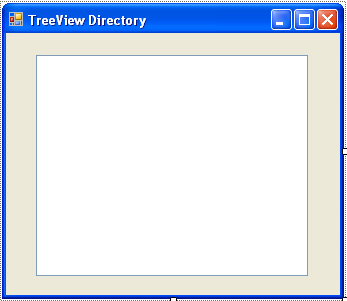
3. Add
Imports System.IO to the topmost part of the code tab because we will access the directory.
4. Create a method named
PopulateTreeView with directoryValue As String and parentNode As TreeNode as parameters.
On this, create a try and catch method. In the try method, create an array variable (string) named
directoryArray that holds and gets the directory either C or D.
Public Sub PopulateTreeView(ByVal directoryValue As String, ByVal parentNode As TreeNode)
Try
Dim directoryArray As String() = Directory.GetDirectories(directoryValue)
Now, create an If statement that if your computer has obtained a directory this will add all the directory folders to the treeview using For Next Loop.
If directoryArray.Length <> 0 Then
Dim currentDirectory As String
For Each currentDirectory In directoryArray
Dim myNode As TreeNode = New TreeNode(currentDirectory)
parentNode.Nodes.Add(myNode)
Next
End If
In the catch method, put this code below to handle UnauthorizedAccessException and will prompt the user "Access Denied".
Catch unauthorized As UnauthorizedAccessException
parentNode.Nodes.Add("Access Denied")
End Try
5. Add this code for the form_load of your program. This will load the C directory structure using the PopulateTreeView method that we have created above.
Private Sub Form1_Load(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles MyBase.Load
TreeView1.Nodes.Add("C:")
PopulateTreeView("C:\", TreeView1.Nodes(0))
End Sub
Full source code:
Imports System.IO
Public Class Form1
Private Sub Form1_Load(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles MyBase.Load
TreeView1.Nodes.Add("C:")
PopulateTreeView("C:\", TreeView1.Nodes(0))
End Sub
Public Sub PopulateTreeView(ByVal directoryValue As String, ByVal parentNode As TreeNode)
Try
Dim directoryArray As String() = Directory.GetDirectories(directoryValue)
If directoryArray.Length <> 0 Then
Dim currentDirectory As String
For Each currentDirectory In directoryArray
Dim myNode As TreeNode = New TreeNode(currentDirectory)
parentNode.Nodes.Add(myNode)
Next
End If
Catch unauthorized As UnauthorizedAccessException
parentNode.Nodes.Add("Access Denied")
End Try
End Sub
End Class
Output:
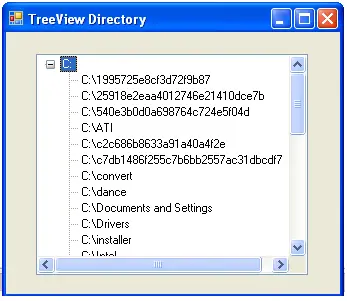
Download the source code below and try it! :)
Best Regards,
Engr. Lyndon R. Bermoy
IT Instructor/System Developer/Android Developer/Freelance Programmer
Mobile: 09488225971
E-mail:
[email protected]
Visit and like my page on Facebook at:
https://www.facebook.com/BermzISware